How to get the url parameters using AngularJS
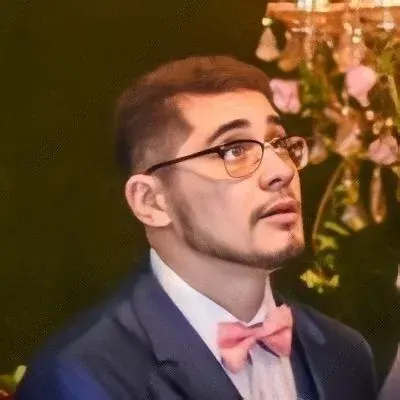
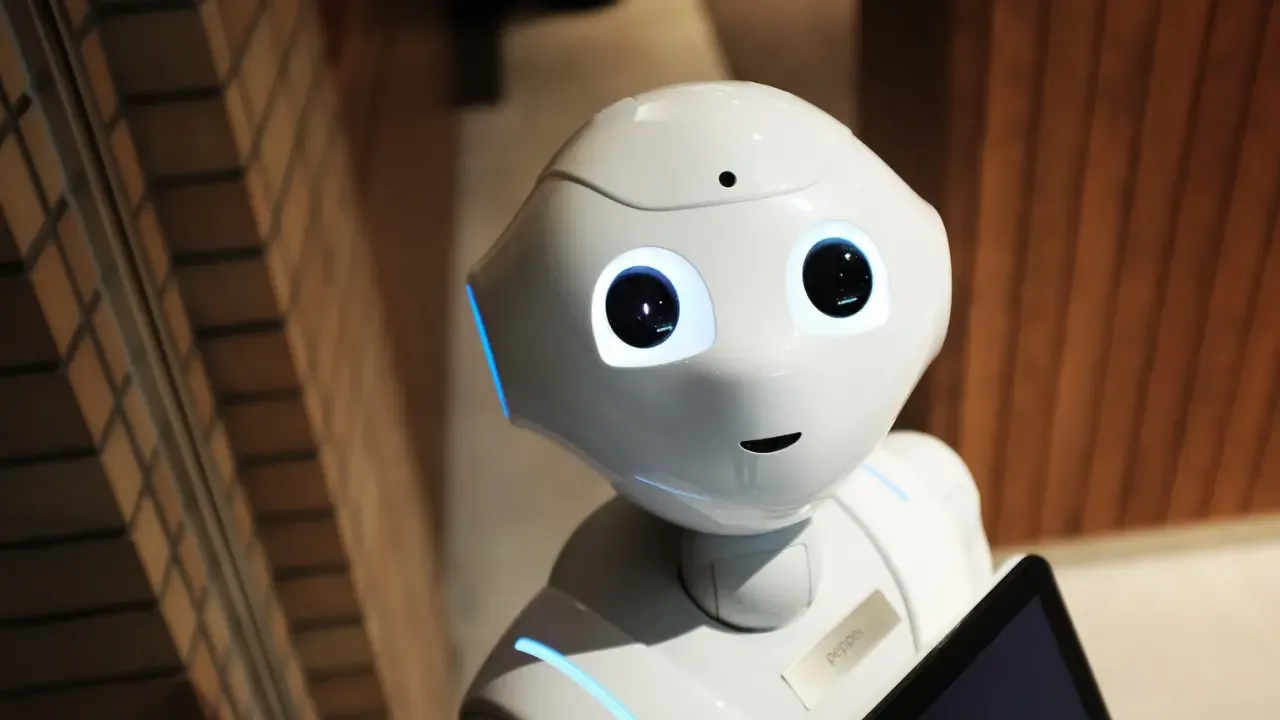
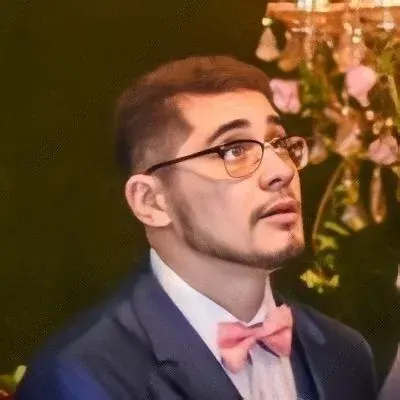
How to Get URL Parameters Using AngularJS
š Have you ever wanted to get the parameters from a URL using AngularJS? š It can be a bit confusing, especially if you're new to AngularJS. But fear not! š In this blog post, we'll guide you through the process step by step and provide easy solutions to this problem. Let's get started! š
The Problem
š The problem is to extract the URL parameters using AngularJS. We have some HTML source code and AngularJS code to work with. Our goal is to obtain the values of the URL parameters and store them in variables.
The HTML Source Code
āļø Before we dive into the solution, let's take a look at the provided HTML source code. It contains a div
element with some AngularJS directives, such as ng-app
and ng-controller
. Inside this div
, we have a div
element with the ng-address-bar
directive, and some expressions that display the results of the URL parameters values. Here's the code:
<div ng-app="">
<div ng-controller="test">
<div ng-address-bar browser="html5"></div>
<br><br>
$location.url() = {{$location.url()}}<br>
$location.search() = {{$location.search('keyword')}}<br>
$location.hash() = {{$location.hash()}}<br>
keyword value is={{loc}} and ={{loc1}}
</div>
</div>
The AngularJS Source Code
š Now, let's examine the AngularJS source code provided. It contains a controller function called test
, which takes two parameters: $scope
and $location
. Inside this function, we assign the $location
object to the $scope.$location
variable. Then, we set the value of $scope.url
to the result of calling $location.url()
with a test URL. Finally, we extract the values of the URL parameters and store them in the variables loc
and loc1
. Here's the code:
function test($scope, $location) {
$scope.$location = $location;
$scope.url = $scope.$location.url('www.html.com/x.html?keyword=test#/x/u');
$scope.loc1 = $scope.$location.search().keyword;
if ($location.url().indexOf('keyword') > -1) {
$scope.loc = $location.url().split('=')[1];
$scope.loc = $scope.loc.split("#")[0];
}
}
The Solution
š” To solve the problem of extracting URL parameters using AngularJS, we'll modify the AngularJS code provided. We'll make use of the $location.search()
method, which returns an object containing all the URL parameters. Here's the updated code:
function test($scope, $location) {
$scope.$location = $location;
$scope.url = $scope.$location.url('www.html.com/x.html?keyword=test#/x/u');
$scope.loc1 = $scope.$location.search().keyword;
if ($scope.loc1) {
$scope.loc = $scope.loc1;
}
}
š In the updated code, we assign the URL to $scope.url
just like before. Then, we use $scope.$location.search().keyword
to get the value of the keyword
parameter. If the parameter exists, we assign its value to $scope.loc
.
Is This the Correct Way?
ā Finally, the question arises: "Is this the correct way to extract URL parameters using AngularJS?" š¤ The answer depends on your specific use case. If your URL always contains a keyword
parameter and you're interested in its value, then yes, the provided solution is correct. However, if your URL parameters may vary or you need to handle more complex scenarios, you might need to modify the solution accordingly.
š” Always keep in mind that the solution should be tailored to your specific requirements. Don't hesitate to experiment and adapt the code to fit your needs.
Conclusion
š Congratulations! š You now know how to extract URL parameters using AngularJS! We've walked you through the problem, provided an easy solution, and explained how to modify the code to meet your requirements. Now it's your turn to give it a try! š Feel free to experiment, ask questions, and share your experiences in the comments below. We'd love to hear from you! š¬