How to get the image size (height & width) using JavaScript
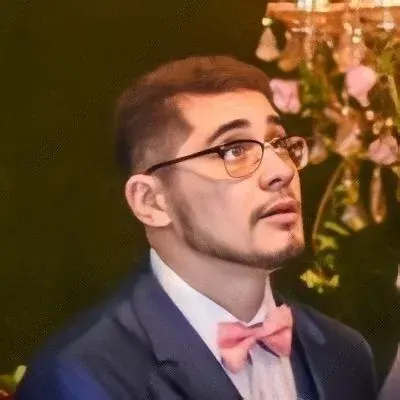
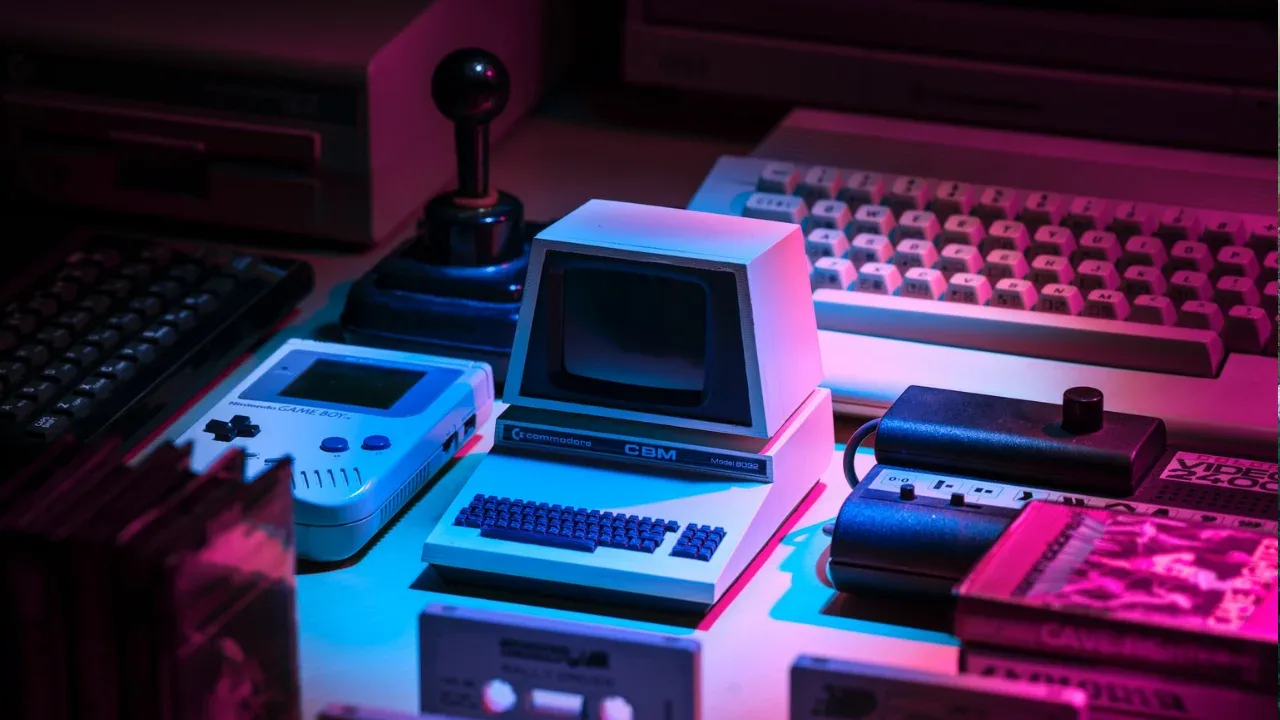
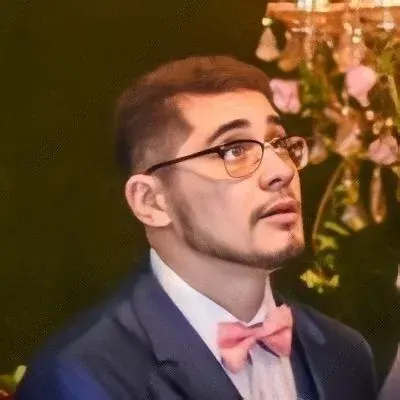
📷 How to Get the Size of an Image Using JavaScript 🖥️
Do you ever find yourself needing to find out the dimensions (height and width) of an image on a web page using JavaScript? 🤔 Well, you're in luck! In this blog post, we'll explore different methods and provide easy solutions on how to achieve this. Let's dive right in! 🚀
The Common Problem 🔍
Many developers often encounter situations where they need to retrieve the dimensions of an image dynamically. This could be useful for various reasons, such as when you want to resize, scale, or position the image based on its original size. However, it can be tricky to know how to tackle this problem. 😓
Easy Solutions 💡
1. The Vanilla JavaScript Way
One simple and straightforward method to obtain the dimensions of an image using JavaScript is by leveraging the power of the Image
object. Here's an example:
const img = new Image();
img.onload = function() {
const imageWidth = this.width;
const imageHeight = this.height;
console.log(`Image Width: ${imageWidth}px`);
console.log(`Image Height: ${imageHeight}px`);
};
img.src = 'path/to/your/image.jpg';
By creating a new Image
object and setting the onload
event handler, we can access the width
and height
properties once the image has finished loading.
2. The jQuery Shortcut 🎯
For those of you who are already using jQuery on your web pages, here's a more concise way to achieve the same result:
const $img = $('<img src="path/to/your/image.jpg">');
$img.on('load', function() {
const imageWidth = this.width;
const imageHeight = this.height;
console.log(`Image Width: ${imageWidth}px`);
console.log(`Image Height: ${imageHeight}px`);
});
As you can see, we create a new img
element using the $()
function and set the load
event handler to retrieve the dimensions once the image is fully loaded.
Want More? 🌟
If you found this post helpful and want to explore more JavaScript tricks and tips, be sure to check out our other blog posts! And don't forget to follow us on social media for regular updates and new content. 📚🌐
Now that you know how to fetch the size of an image using JavaScript and jQuery, go ahead and implement this knowledge in your own projects. 🙌 Feel free to share any other cool and creative ways you've used this technique in the comments below! Let's learn from each other. 👇
Happy coding! 💻💡