How to get progress from XMLHttpRequest
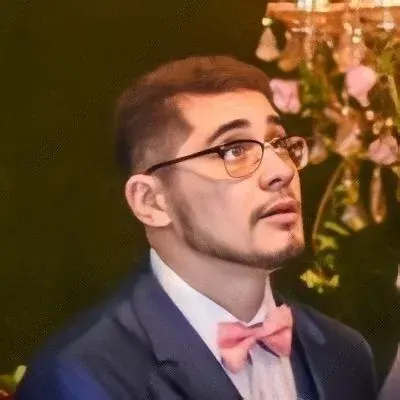
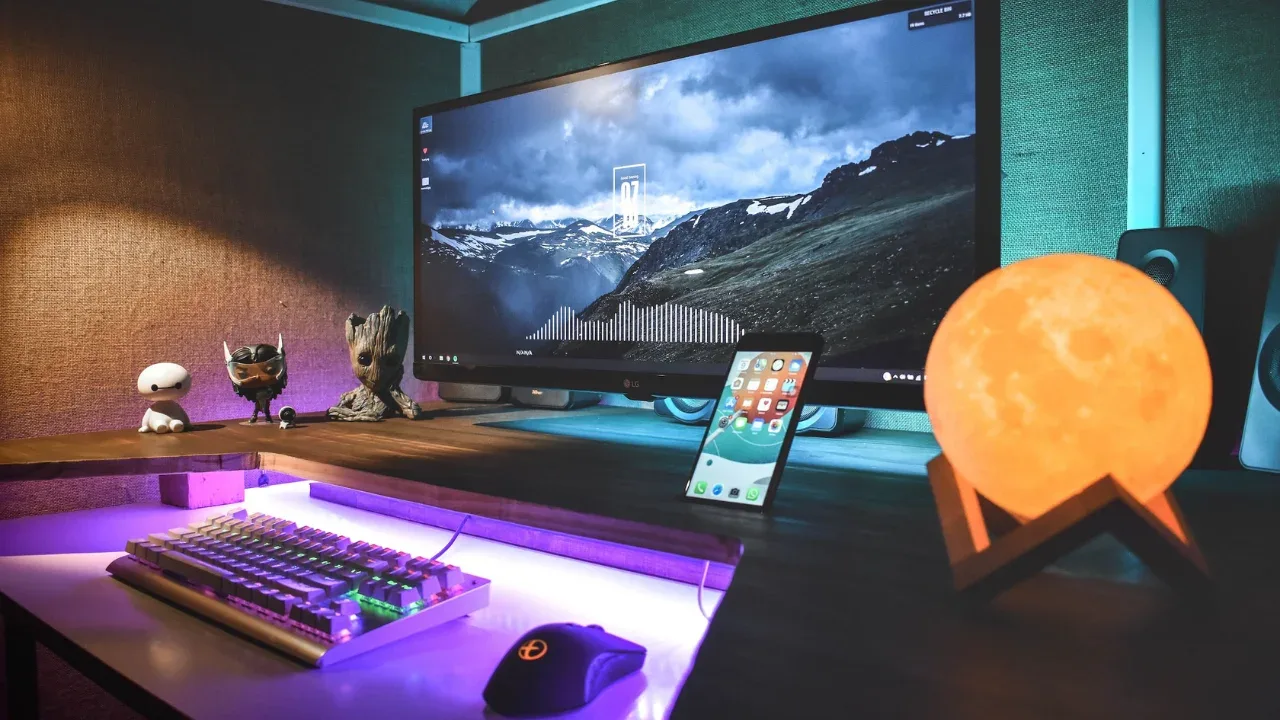
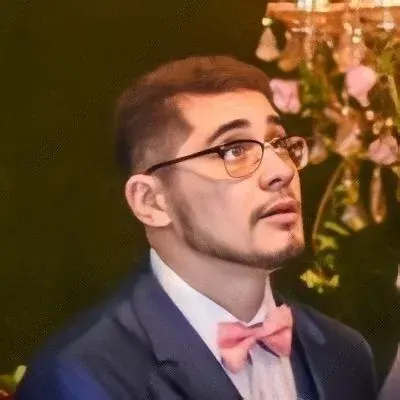
How to Get Progress from XMLHttpRequest 📶
Introduction 🔍
Do you ever wonder how to display a progress bar when uploading large files using XMLHttpRequest? 📁 It seems like a basic and essential feature to have, as the client knows the number of bytes uploaded or downloaded. 🔄 But unfortunately, the standard XMLHttpRequest API does not directly support this. 😞 However, worry not! In this blog post, we will explore a few simple solutions to tackle this common problem. Let's dive in! 🏊♂️
The Problem and Challenges 🤔
The primary issue with the standard XMLHttpRequest is the lack of built-in progress information. 😓 To address this, the default workaround is to continuously poll the server for progress updates. 👀 While this method works, it comes with its own set of challenges. Let's take a look at them:
Complicated server-side code: Constantly polling the server requires additional code on the server-side to handle and respond to these requests. This can significantly increase the complexity of your codebase. 🧩
Poor upstream connections: Uploading large files can be a painstakingly slow process, especially when most ISPs offer poor upstream bandwidth. When the user's connection is struggling, making extra requests for progress updates adds further burden to the responsiveness. ⏳
Solutions at Hand 🙌
Although the standard API lacks the progress functionality, there are alternative approaches to overcome this limitation. Let's explore a couple of solutions:
1. Use the non-standard progress
event 🔄
Some modern browsers provide a non-standard extension to XMLHttpRequest called the progress
event. 🌐 This event allows you to track the progress of an ongoing request. Here's a simple example of how you can utilize it:
const xhr = new XMLHttpRequest();
xhr.addEventListener('progress', (event) => {
if (event.lengthComputable) {
const percent = (event.loaded / event.total) * 100;
console.log(`Progress: ${percent.toFixed(2)}%`);
}
});
xhr.open('POST', 'your-upload-endpoint');
xhr.send(formData);
By listening to the progress
event, you can access the loaded
and total
attributes in the event object, which represent the number of bytes uploaded or downloaded and the total file size, respectively. 📤
However, keep in mind that this approach should be used sparingly as it is non-standard and may not be supported across all browsers. 🚨
2. Utilize newer technologies such as Fetch API 🌟
Another solution is to move away from XMLHttpRequest and embrace newer technologies like the Fetch API. 🚀 The Fetch API provides a more modern and streamlined way to make HTTP requests, including support for tracking progress.
Here's an example of using the Fetch API with progress tracking:
fetch('your-upload-endpoint', {
method: 'POST',
body: formData,
}).then((response) => {
const reader = response.body.getReader();
let totalBytes = 0;
let loadedBytes = 0;
return reader.read().then(function processResult(result) {
if (result.done) {
console.log('Upload complete!');
return;
}
loadedBytes += result.value.length;
totalBytes = response.headers.get('Content-Length');
const percent = (loadedBytes / totalBytes) * 100;
console.log(`Progress: ${percent.toFixed(2)}%`);
return reader.read().then(processResult);
});
});
In this example, we use the Fetch API's streaming feature to access a ReadableStream
from the response body. This allows us to track progress by reading chunks of data and calculating the percentage based on the total bytes. 📊
The Fetch API offers more control and flexibility, making it an excellent alternative for handling progress tracking.
Conclusion and Next Steps 🏁
While the standard XMLHttpRequest lacks native progress tracking, there are viable alternatives to overcome this limitation. 🚀 By utilizing non-standard features like the progress
event or embracing modern technologies such as the Fetch API, you can display progress bars and provide a more interactive user experience during file uploads. 📥
Now that you have learned how to get progress from XMLHttpRequest, it's time to take action and implement these solutions in your own projects. 💪 Remember, user engagement can be significantly enhanced by utilizing progress bars and providing real-time feedback on long-running processes. So go ahead, experiment, and transform your user experiences like a pro! 🌟