How to get object length
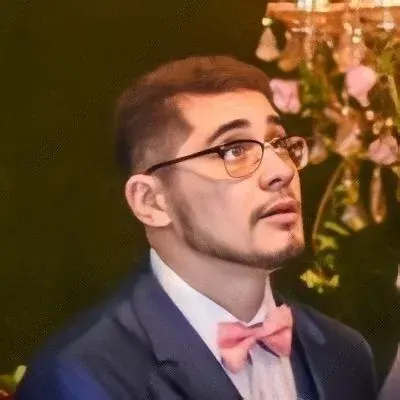
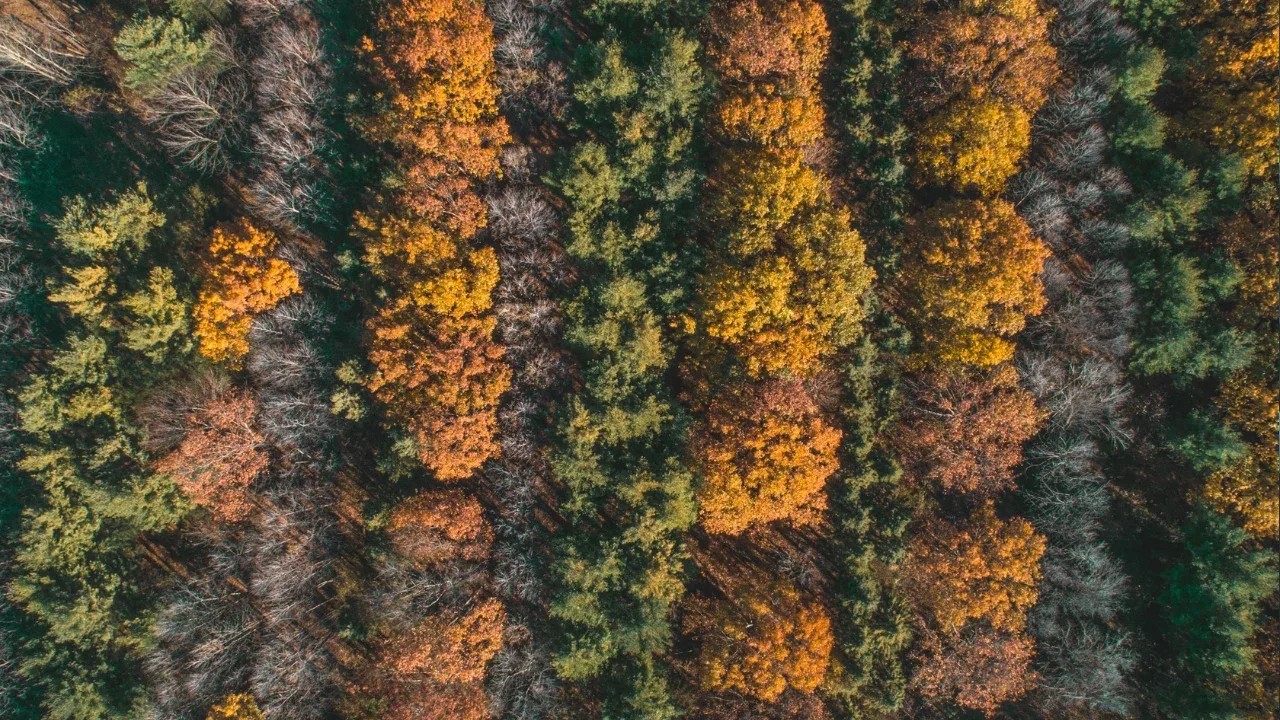
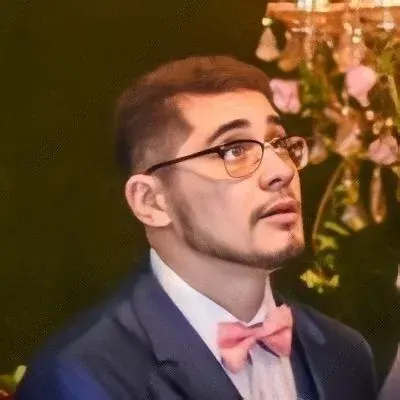
How to Get Object Length: A Simple Guide ๐
Have you ever found yourself wondering how to determine the length of an object in JavaScript? ๐ค You're not alone! Understanding how to obtain the length of an object can be puzzling, especially when built-in functions don't seem to work. But fret not, as we've got you covered with some easy solutions! ๐ช
The Dilemma โ
Let's consider the following scenario: You have an object ๐ฆ, let's call it a
, with three key-value pairs:
a = { 'a': 1, 'b': 2, 'c': 3 };
Naturally, you'd expect the length of this object to be 3, right? Well, if you try to obtain the length using the built-in length
property like this:
a.length;
You'll be disappointed to find that it returns undefined
. ๐ฑ Don't worry, this is simply because the length
property is designed specifically for arrays, not objects!
The Workaround ๐ก
So, what's the solution? While there isn't a direct built-in function to get the length of an object, we can easily achieve our goal using a simple workaround. ๐กโจ
Solution 1: Object.keys() Method ๐
One way to get the length of an object is by using the Object.keys()
method. This method returns an array containing the object's enumerable property names. By accessing the length
property of this array, we can obtain the desired result. ๐
Here's how you can do it:
const length = Object.keys(a).length;
By applying this method to our object a
, we'll get a length of 3
. ๐
Solution 2: for...in Loop ๐
Another option is to use a for...in
loop to iterate over the object's properties and increment a counter variable. This allows us to count each key-value pair, resulting in the object's length.
Let's take a look at the code:
let counter = 0;
for (let key in a) {
if (a.hasOwnProperty(key)) {
counter++;
}
}
const length = counter;
Using this loop, we traverse through each property of the object a
, incrementing the counter
variable for each key-value pair found. In the end, length
will hold the desired length. ๐
The Call to Action ๐ข
Now that you know how to get the length of an object, it's time to put your skills to the test! ๐ช Share your thoughts and experiences below in the comments section. Did you find these solutions helpful? Do you have any other clever workarounds to share? Let's keep the conversation going! ๐ฌโจ
Happy coding! ๐๐ฉโ๐ป๐จโ๐ป