How to get file name from content-disposition
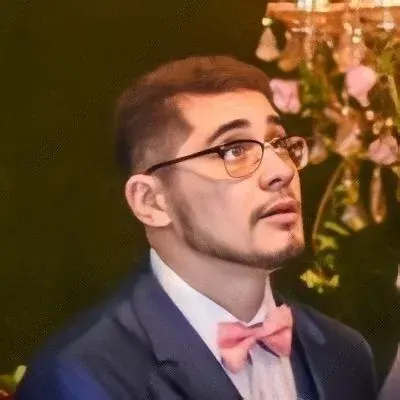
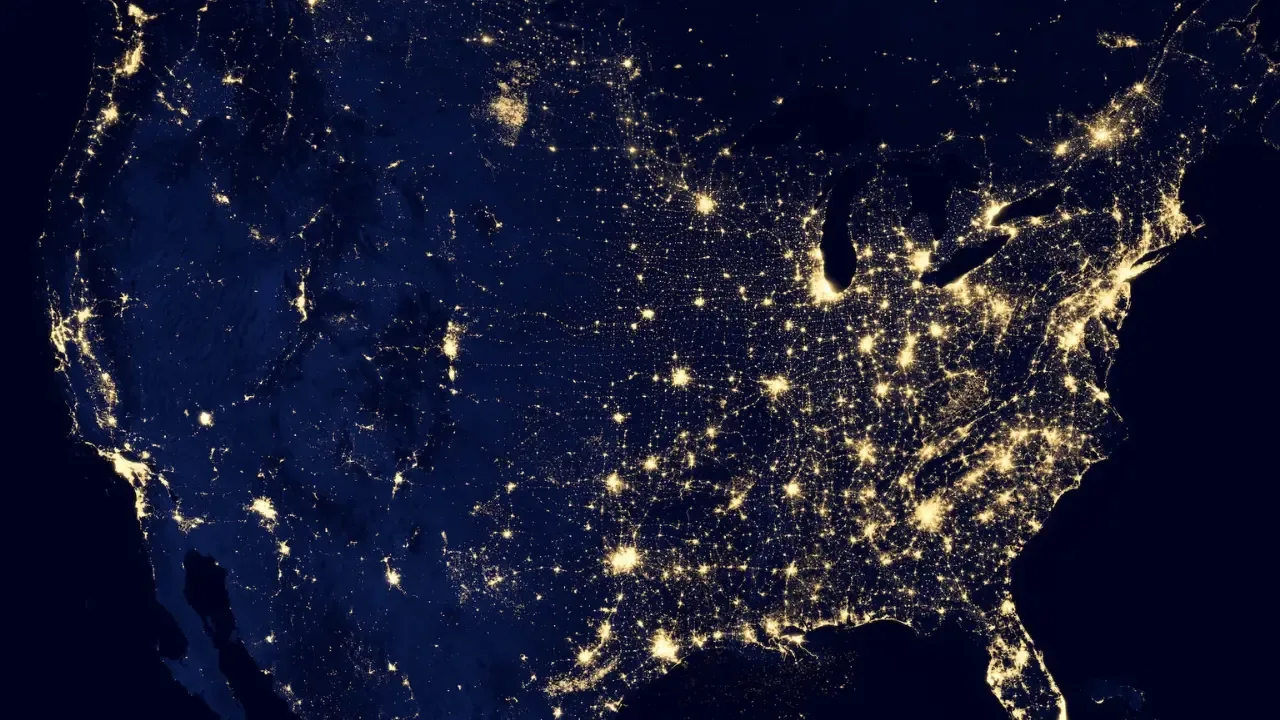
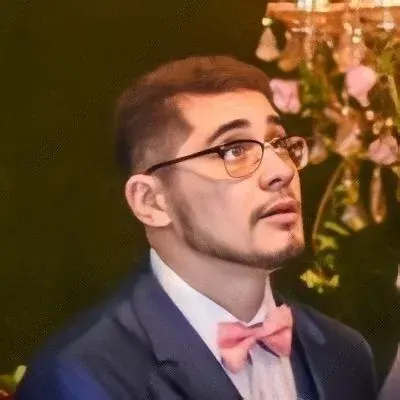
📝 Tech Blog: How to Get File Name from Content-Disposition
Are you struggling to get the file name from the Content-Disposition header in your AJAX response? Don't worry, we've got you covered! In this blog post, we'll address this common issue and provide you with easy solutions to help you display the file name and file type. Let's dive in!
🧩 The Problem: Getting the File Name and File Type
Say you're downloading a file as a response to an AJAX request, but you need to extract the file name and file type from the Content-Disposition header. However, you've searched high and low and couldn't find the right way to do it. Sound familiar? Many developers have faced this challenge, so you're not alone.
💡 The Solution: Step-by-Step Guide
We're here to help! Follow these steps to extract the file name and file type from the Content-Disposition header and display a thumbnail for the downloaded file:
Begin by capturing the AJAX response in the success callback function. In the example code, the response is stored in the
header
variable.success: function (response, status, xhr) { var header = xhr.getResponseHeader('Content-Disposition'); console.log(header); }
The
xhr.getResponseHeader('Content-Disposition')
function retrieves the content disposition header as a string. In our example, it returnsinline; filename=demo3.png
as the console output.To extract the file name from the header, you can use JavaScript string manipulation techniques. In this case, we can split the header string on the '; ' separator and retrieve the second part.
var filename = header.split('; ')[1].split('=')[1]; console.log('File Name:', filename);
The output will be:
File Name: demo3.png
.To extract the file type, we can split the header string again, but this time on the '/' separator and retrieve the second part.
var fileType = header.split('; ')[1].split('=')[1].split('.')[1]; console.log('File Type:', fileType);
The output will be:
File Type: png
.With the file name and file type extracted, you can now display a thumbnail for the downloaded file using appropriate HTML and CSS. For example:
<div> <img src="thumbnails/demo3.png" alt="Thumbnail of demo3.png"> <p>File Name: demo3.png</p> <p>File Type: png</p> </div>
Customize the HTML and CSS with your desired styling and file paths.
Finally, test your implementation by triggering the AJAX request and ensure that the file name and file type are correctly extracted and displayed.
📣 Stay Engaged!
Now that you've learned how to extract the file name and file type from the Content-Disposition header, why not share your experience in the comments section below? Have you faced any challenges with AJAX responses before? We'd love to hear about it and help you out!
Don't forget to subscribe to our newsletter for more insightful guides and helpful solutions to common tech problems. And, as always, happy coding! 😄👩💻👨💻