How to get client"s IP address using JavaScript?
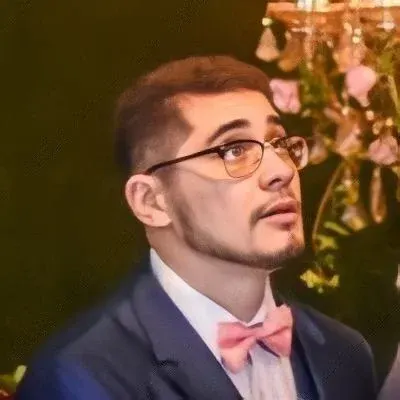
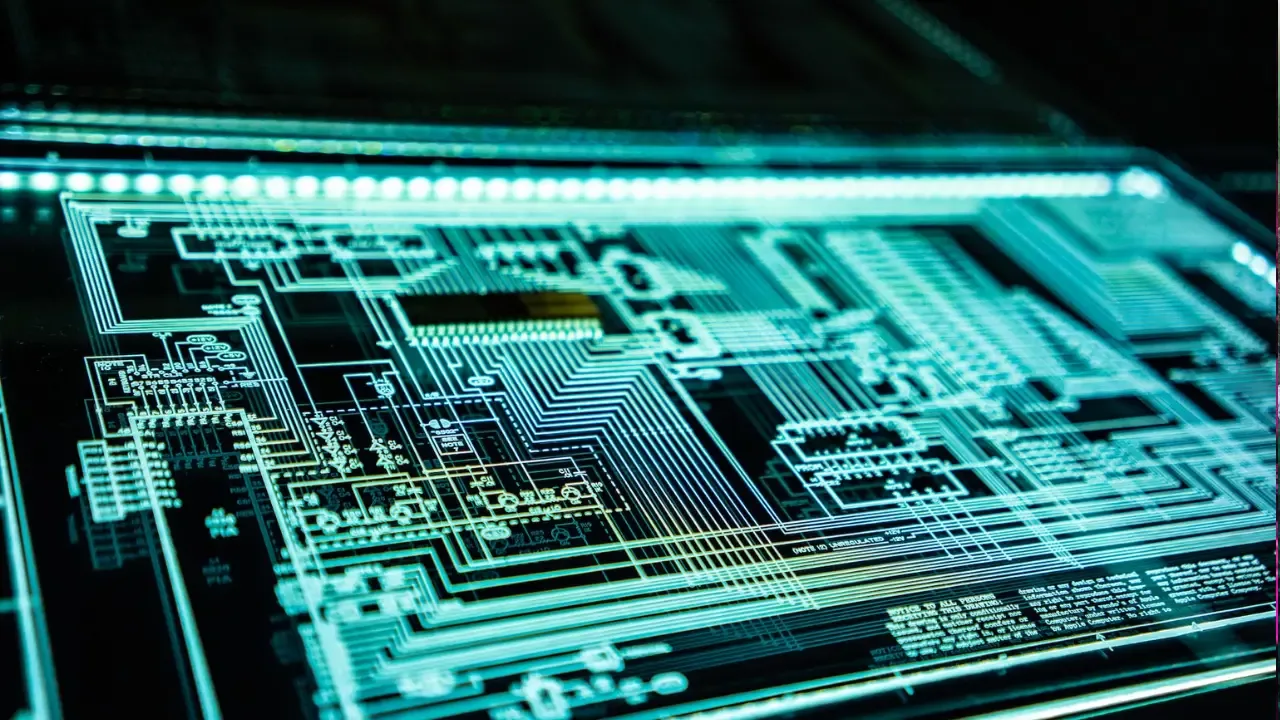
How to get client's IP address using JavaScript?
šš»š
Have you ever wondered how you can retrieve the IP address of your website visitors using JavaScript? Maybe you want to personalize their experience or track their location for analytics purposes. In this blog post, we'll explore different methods to get a client's IP address and provide easy solutions to common issues.
The Challenge:
The challenge posed here is to retrieve the client's IP address using JavaScript without any server-side code, even Server Side Includes (SSI). But fear not! We have a couple of options that don't require server-side code.
Solution 1: Free 3rd Party Script/Service
As mentioned in the context, you can use a third-party service to obtain the IP address. There are many free services available that provide simple JavaScript snippets to get the client's IP address. One popular option is to use the ipify service.
Here's how you can utilize ipify in your JavaScript code:
fetch('https://api.ipify.org?format=json')
.then(response => response.json())
.then(data => {
const ipAddress = data.ip;
console.log(ipAddress);
// Do something with the IP address
});
In this example, we use the Fetch API to send a GET request to the ipify API. The response is then converted into JSON format, and the IP address is extracted from the data.
Solution 2: WebSocket Connection
Another way to retrieve the client's IP address is by establishing a WebSocket connection. This method allows for real-time communication between the client and server. By utilizing the WebSocket
API, we can extract the IP address during the handshake process.
Here's an example of how to achieve this:
const socket = new WebSocket('wss://echo.websocket.org');
socket.addEventListener('open', () => {
const ipAddress = socket._socket.remoteAddress;
console.log(ipAddress);
// Do something with the IP address
});
// Handle the rest of the WebSocket events accordingly
In this code snippet, we establish a WebSocket connection with the wss://echo.websocket.org
server. Upon a successful connection, the open
event is triggered, allowing us to access the remote address, which represents the client's IP address.
Conclusion:
Retrieving the client's IP address using JavaScript might seem like a daunting task, especially without server-side code. But with the help of third-party services like ipify or by utilizing a WebSocket connection, we can easily obtain this information.
Remember to always check the terms and conditions of any third-party service you use and consider the impact on your website's performance and privacy.
Now it's your turn! Share your experiences or any other methods you have tried in the comments below. And don't forget to follow us for more helpful tech tips! šš¬
P.S. If you found this guide helpful, share it with your fellow developers to help them solve this common problem! ššØāš»š©āš»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
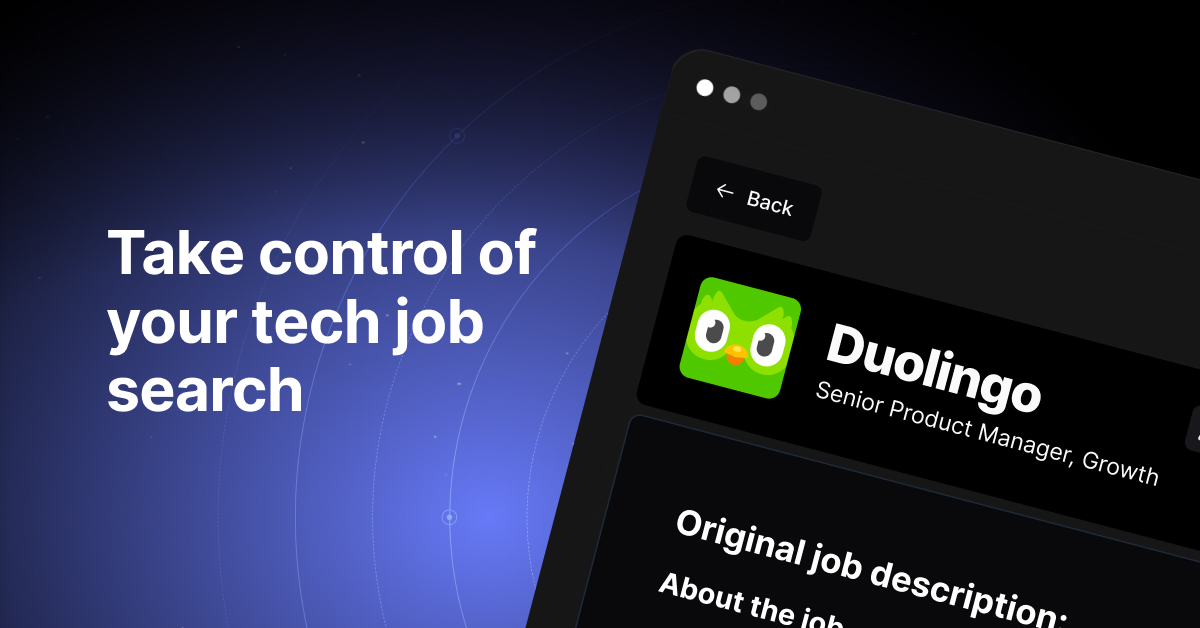