How to generate a simple popup using jQuery
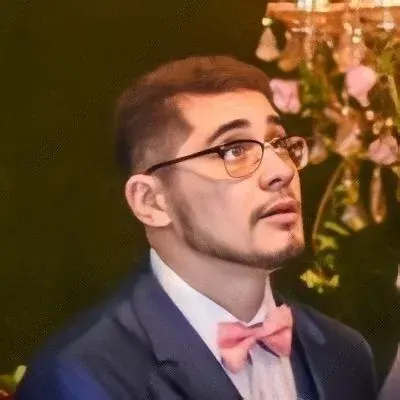
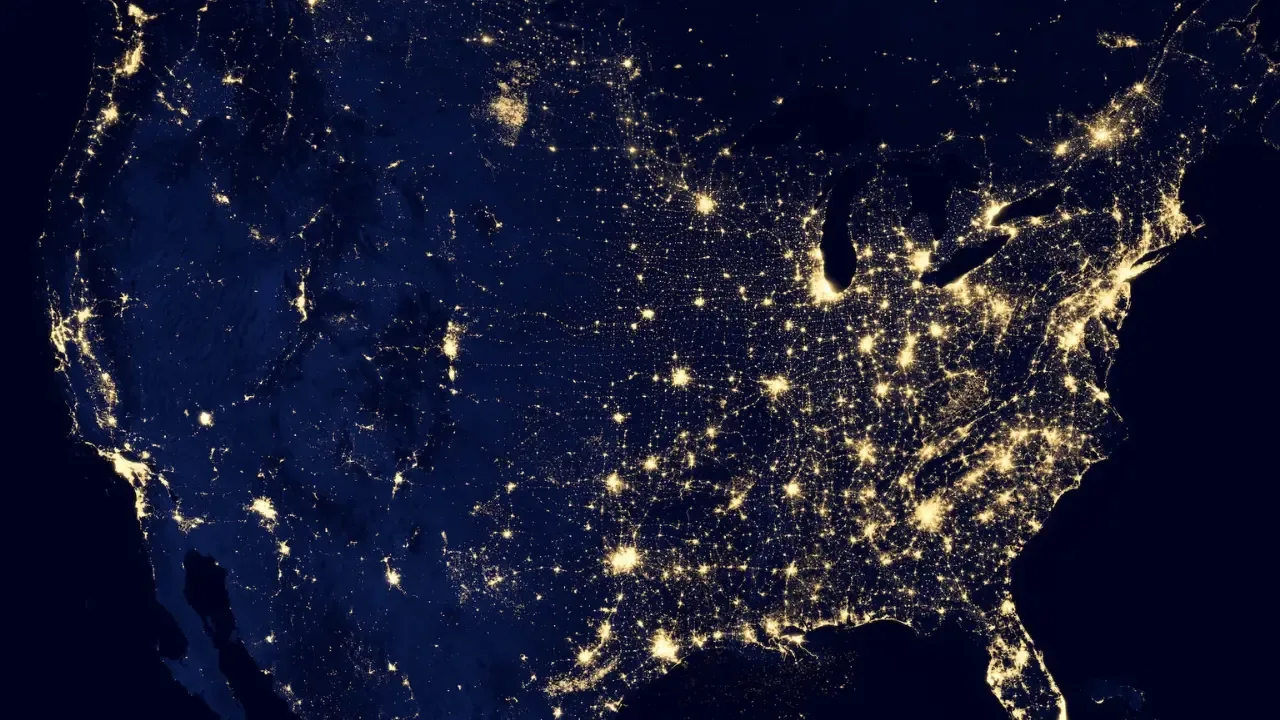
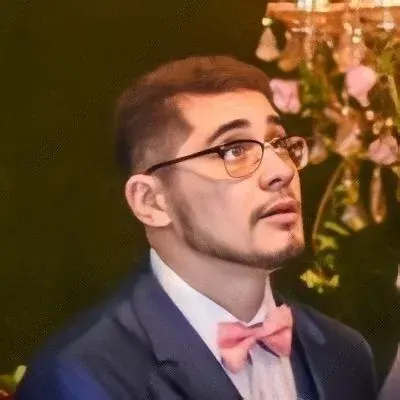
How to Generate a Simple Popup Using jQuery
So, you're designing a web page and want to add a cool popup window when someone clicks on the content of a specific div. 🤔 No worries, I've got you covered! In this guide, we'll walk through the process of creating a simple popup using jQuery. By the end, you'll have an awesome and interactive way to display information to your users. Let's get started! 🚀
The Problem
Your main challenge is to show a popup window with a label for email and a text box when someone clicks on a specific div. This is a common issue when adding interactivity to a webpage, and luckily, jQuery offers a simple solution for that. 💡
The Solution
To achieve the desired effect, follow these easy steps:
Step 1: Include jQuery Library
First things first, make sure to include the jQuery library in your HTML file. You can either download it and host it yourself or use a Content Delivery Network (CDN) to include it from a remote location. Here's an example of how to include it using a CDN:
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
Step 2: Create HTML Structure
Next, let's create the necessary HTML structure for the popup. In this case, we'll use a <div>
element with an ID of "popup" that will contain the label and textbox. Here's an example:
<div id="popup">
<label for="email">Email:</label>
<input type="text" id="email" />
</div>
Step 3: Add CSS Styling
To make the popup visually appealing, we'll need to add some CSS styles. Feel free to customize this part to match your website's design. Here's an example of CSS styles you can add:
#popup {
display: none;
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background-color: #fff;
padding: 20px;
border-radius: 5px;
box-shadow: 0 2px 5px rgba(0, 0, 0, .5);
}
#popup label {
display: block;
margin-bottom: 10px;
}
#popup input {
width: 100%;
padding: 5px;
border-radius: 3px;
border: 1px solid #ccc;
}
Step 4: Add jQuery Code
Now it's time to add the jQuery code that will handle the popup functionality. We'll use the .click()
method to trigger the popup when the div is clicked, and the .toggle()
method to show and hide the popup. Here's an example of how to do it:
$(document).ready(function() {
$('#mail').click(function() {
$('#popup').toggle();
});
});
Step 5: Test It Out
That's it! Now you should have a working popup on your web page. Give it a try by clicking the div with the ID of "mail" and see the magic happen! ✨
Take It Further
Now that you have a basic understanding of how to generate a simple popup using jQuery, you can take it further by adding more functionality. For example, you could include form validation, animations, or even integrate it with a server-side script to store the submitted data.
Your Turn!
Give it a try and let me know how it goes! Share your experience in the comments below and feel free to ask any questions you might have. I'd love to see what cool popups you come up with using jQuery! 😄