How to format numbers as currency strings
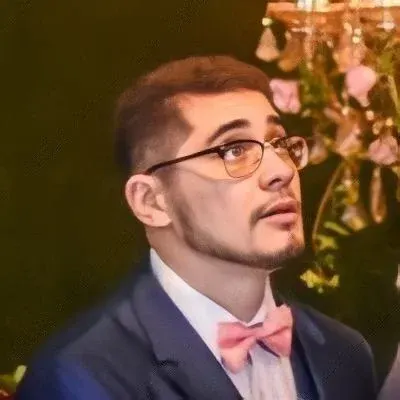

π°π΅πΈ How to Format Numbers as Currency Strings π°π΅πΈ
So you're looking to format a number as a currency string in JavaScript, huh? π»ππ‘ Formatting numbers as currency strings can be quite useful when dealing with financial applications, e-commerce platforms, or any situation where you need to display monetary values in a user-friendly way. In this blog post, I'll show you some easy solutions to achieve this formatting feat. πͺπ€π°
π The Problem: You need a function that takes a float as an argument and returns a string formatted like "$ 2,500.00". π²π°β
π οΈ The Solution: We have a couple of options to achieve the desired outcome. Let's take a look at two popular approaches:
1οΈβ£ Using the toLocaleString() method: The toLocaleString() method is a built-in JavaScript method that helps format numbers according to the language and region settings of the user.
function formatCurrency(number) {
return number.toLocaleString('en-US', { style: 'currency', currency: 'USD' });
}
The code snippet above takes advantage of the toLocaleString() method, specifying 'en-US' as the locale for English (United States) and 'USD' as the currency symbol. You can modify these values as necessary to match your requirements.
π‘ The toLocaleString() method automatically handles decimal separators, thousands separators, and currency symbol placement based on the user's locale.
Example usage:
console.log(formatCurrency(2500)); // Output: "$2,500.00"
2οΈβ£ Using Intl.NumberFormat: The Intl.NumberFormat API, added in ECMAScript Internationalization API, provides more flexibility for formatting numbers, including currency formatting.
function formatCurrency(number) {
return new Intl.NumberFormat('en-US', { style: 'currency', currency: 'USD' }).format(number);
}
Similar to the previous solution, we can specify 'en-US' as the locale and 'USD' as the currency symbol, but this time using the Intl.NumberFormat API. The output will be formatted as a currency string.
Example usage:
console.log(formatCurrency(2500)); // Output: "$2,500.00"
ππ That's it! You've now learned two easy ways to format numbers as currency strings in JavaScript. Feel free to tweak the code snippets to suit your specific requirements and locales. π°πΈπ΅
π£π¬ Are you struggling with any other JavaScript conundrums? Do you have any other questions or suggestions? Let us know in the comments section below! We'd love to hear from you. Let's keep learning and coding together! π€π©βπ»π¨βπ»
Happy formatting! πΈβ¨β¨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
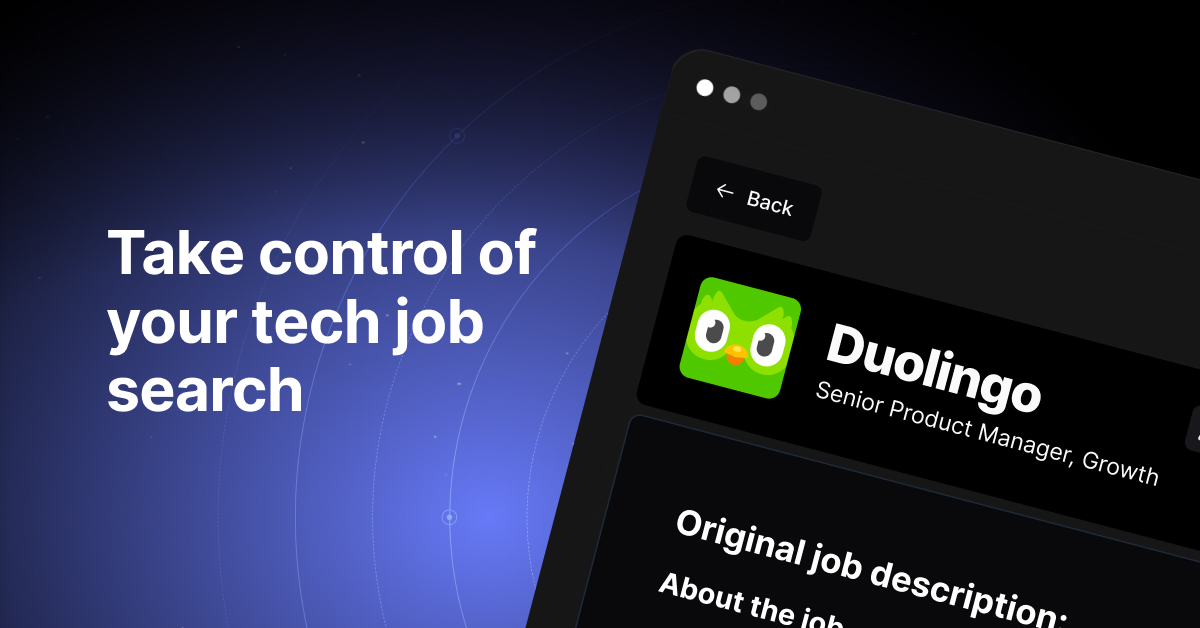