How to format a number with commas as thousands separators?
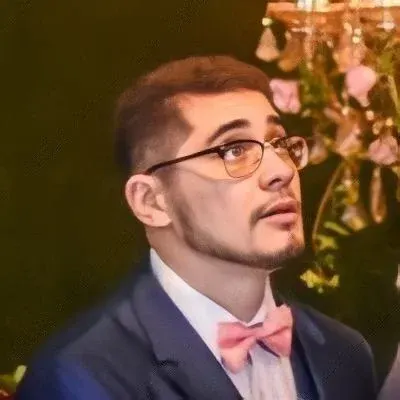
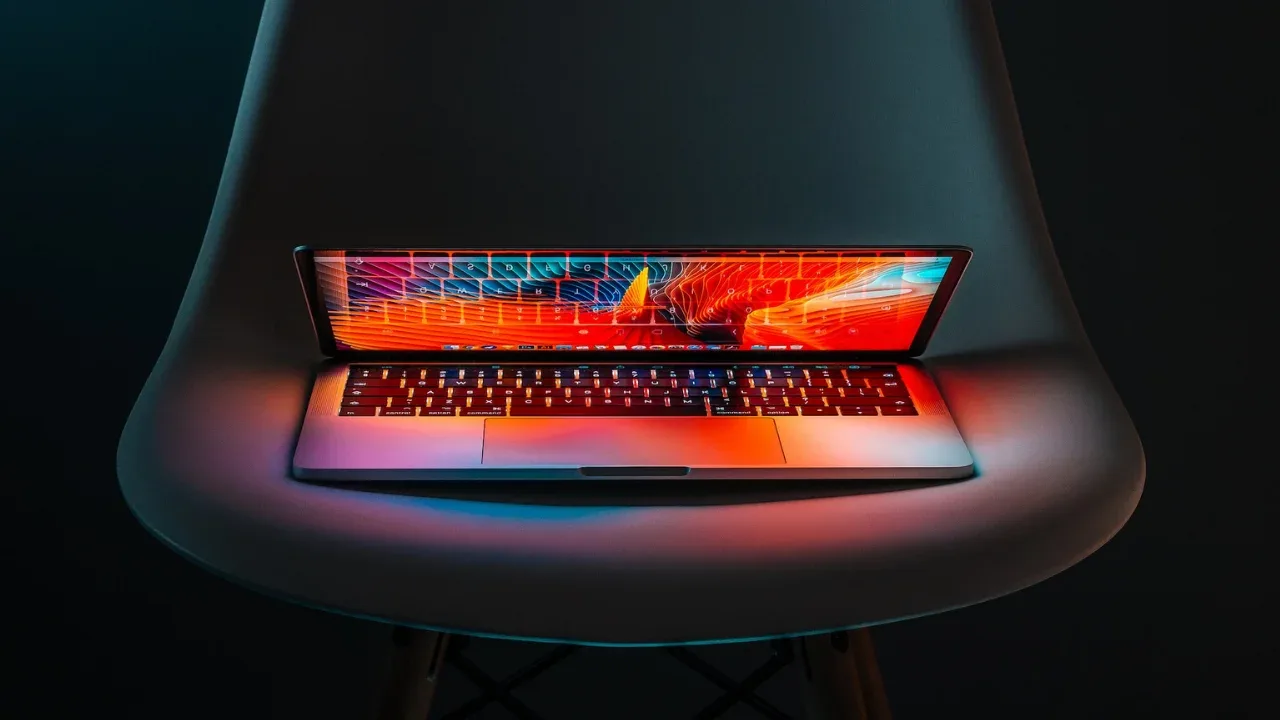
How to Format a Number with Commas as Thousands Separators? 💸
Do you want to make those big numbers easier to read? Need those commas to separate thousands like a pro? Well, you've come to the right place! In this guide, we'll show you how to format a number with commas as thousands separators in JavaScript, addressing common issues and providing easy solutions. Let's dive in!
The Problem 😔
So, you want to print an integer or float in JavaScript with commas as thousands separators. For example, you have the number 1234567, and you want it to be displayed as "1,234,567". Easy, right? But how do you achieve that?
The Solution 🌟
Luckily, we have a nifty little function that does the trick: numberWithCommas()
. Here's the code snippet you can use:
function numberWithCommas(x) {
x = x.toString();
var pattern = /(-?\d+)(\d{3})/;
while (pattern.test(x))
x = x.replace(pattern, "$1,$2");
return x;
}
console.log(numberWithCommas(1000));
The numberWithCommas
function takes an input number and converts it into a string using the toString()
method. It then applies a regex pattern to insert commas every three digits from the right. The replace()
function replaces the matched pattern with the desired format. Finally, it returns the formatted number.
Let's break it down:
x = x.toString();
: This converts the input number to a string, allowing us to manipulate it easily.var pattern = /(-?\d+)(\d{3})/;
: This regex pattern searches for any digit groupings followed by three digits. The(-?\d+)
captures the first group, which can include a negative sign (-
). The(\d{3})
captures the second group, which consists of three digits.while (pattern.test(x))
: This checks if the pattern matches any portion of the string.x = x.replace(pattern, "$1,$2");
: This replaces the matched pattern with itself, but inserts a comma (","
) between the two captured groups.return x;
: This returns the formatted number.
Hooray! 🎉 You can now use the numberWithCommas()
function to format any number with commas as thousands separators.
The Simplified Approach 🤩
But, wait! Is there a simpler or more elegant way to achieve the same result? Absolutely! Let's introduce a built-in JavaScript method, toLocaleString()
. This method formats a number based on the given locale, including comma formatting.
Here's how you can use it:
var number = 1234567;
var formattedNumber = number.toLocaleString();
console.log(formattedNumber);
In this approach, we use the toLocaleString()
method directly on the number. It automatically formats the number according to the user's locale settings, including comma separators for thousands.
💡 Pro Tip: toLocaleString()
also has parameters that allow you to specify the locale and customize the formatting further. Check out the MDN documentation for more details.
Conclusion and Call-to-Action 🚀
Formatting numbers with commas as thousands separators can greatly enhance readability and improve the user experience. Whether you choose the numberWithCommas()
function or the toLocaleString()
method, make sure to apply the one that suits your requirements best.
Now it's your turn! Give it a go and experiment with formatting numbers in JavaScript. Let us know which approach you found most useful and share your thoughts in the comments below.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
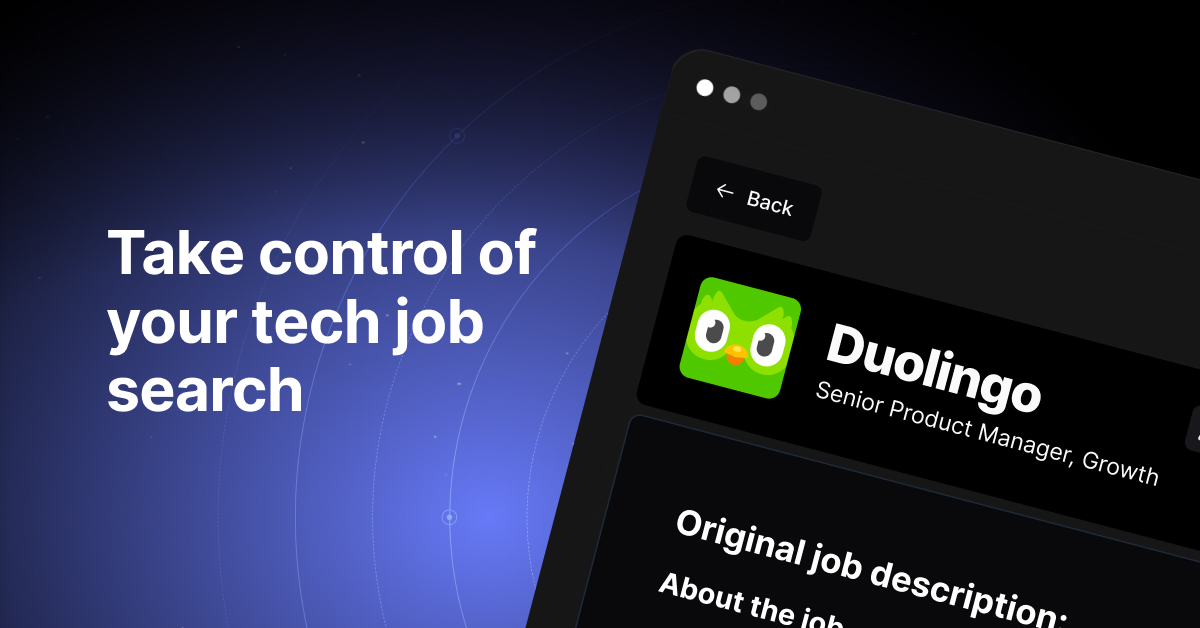