How to find the sum of an array of numbers
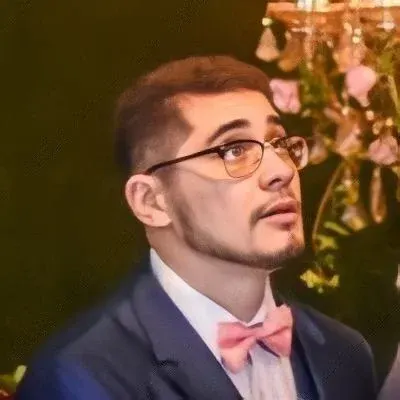

How to 🧮 find the sum of an array of numbers?
You have an array and you want to find the sum of its elements 🤔. Don't worry, I've got you covered! In this post, I'll show you an easy solution to this problem, along with some common issues you might encounter. So let's dive in! 💪
Understanding the problem
Let's start with a given array: [1, 2, 3, 4]
. Our goal is to find the sum of its elements, which in this case would be 10
. Easy peasy, right? 😉
Solution using JavaScript's reduce() method
JavaScript provides a handy method called reduce()
which can help us solve this problem effortlessly. Here's how you can use it:
const array = [1, 2, 3, 4];
const sum = array.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
console.log(sum); // Output: 10
Let's understand what's happening here:
We start with the
array
to which we want to find the sum.We call the
reduce()
method on thearray
.Inside the
reduce()
method, we define a callback function that takes two parameters:accumulator
andcurrentValue
.In each iteration, the
reduce()
method updates theaccumulator
by adding thecurrentValue
to it.Finally, the
reduce()
method returns the accumulated sum.
Here's a step-by-step breakdown of the code for better clarity:
First iteration:
accumulator = 0
(default value),currentValue = 1
➡️accumulator = 0 + 1 = 1
.Second iteration:
accumulator = 1
(previous sum),currentValue = 2
➡️accumulator = 1 + 2 = 3
.Third iteration:
accumulator = 3
(previous sum),currentValue = 3
➡️accumulator = 3 + 3 = 6
.Fourth iteration:
accumulator = 6
(previous sum),currentValue = 4
➡️accumulator = 6 + 4 = 10
.
And voila! We've found the sum of all the elements in the array. 🎉
Common issues to watch out for
While finding the sum of an array seems straightforward, there are a couple of things you should keep in mind to avoid potential pitfalls:
Empty array: If the array is empty, the
reduce()
method will throw an error. Make sure to handle this case separately if you anticipate empty arrays.Non-numeric values: If the array contains non-numeric values, such as strings or objects, the addition operation will concatenate them instead of summing them up. Ensure your array only contains numeric values for accurate results.
Now that you know how to find the sum of an array easily, try it out with different arrays and see how it works! 🚀
Your turn! 📣
I hope this guide helped you in understanding how to find the sum of an array. Now it's your turn to put it into practice! 😊
Task: Take any array of your choice and find its sum using the approach we discussed. Share your code in the comments below and let's compare results! 💻📝
If you have any questions or other cool ways to find the sum, feel free to share them too. Let's discuss and learn from each other! 💬
Happy coding! 💙
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
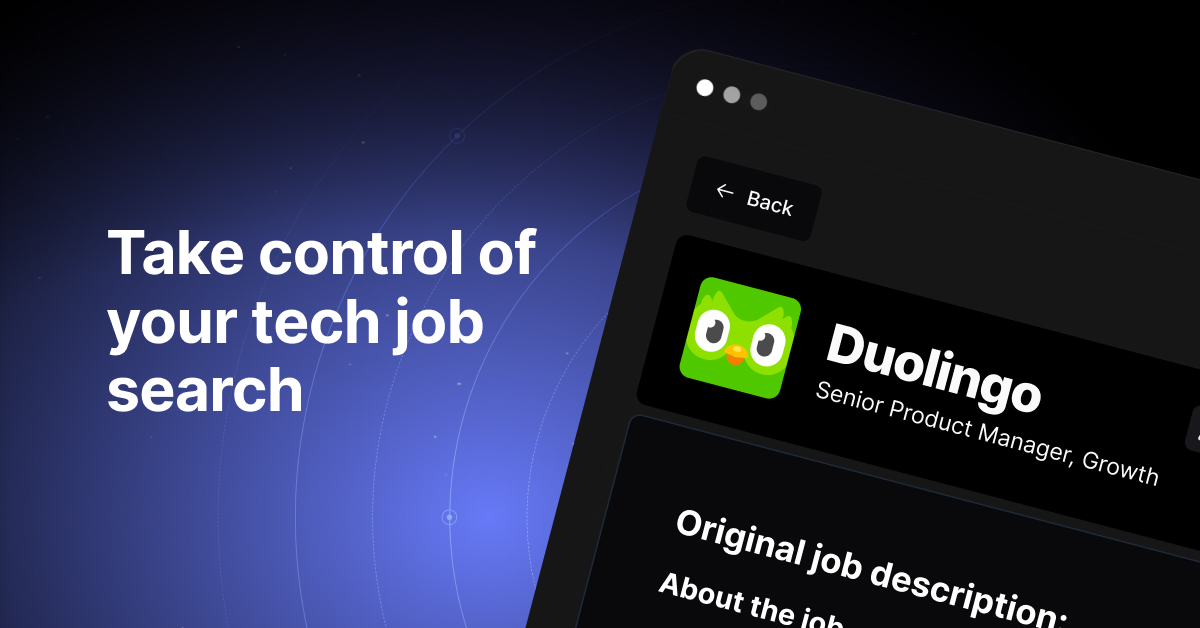