How to find if an array contains a specific string in JavaScript/jQuery?
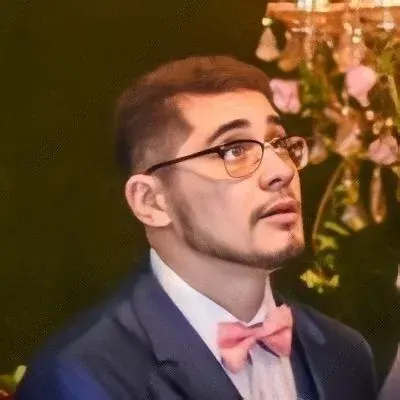
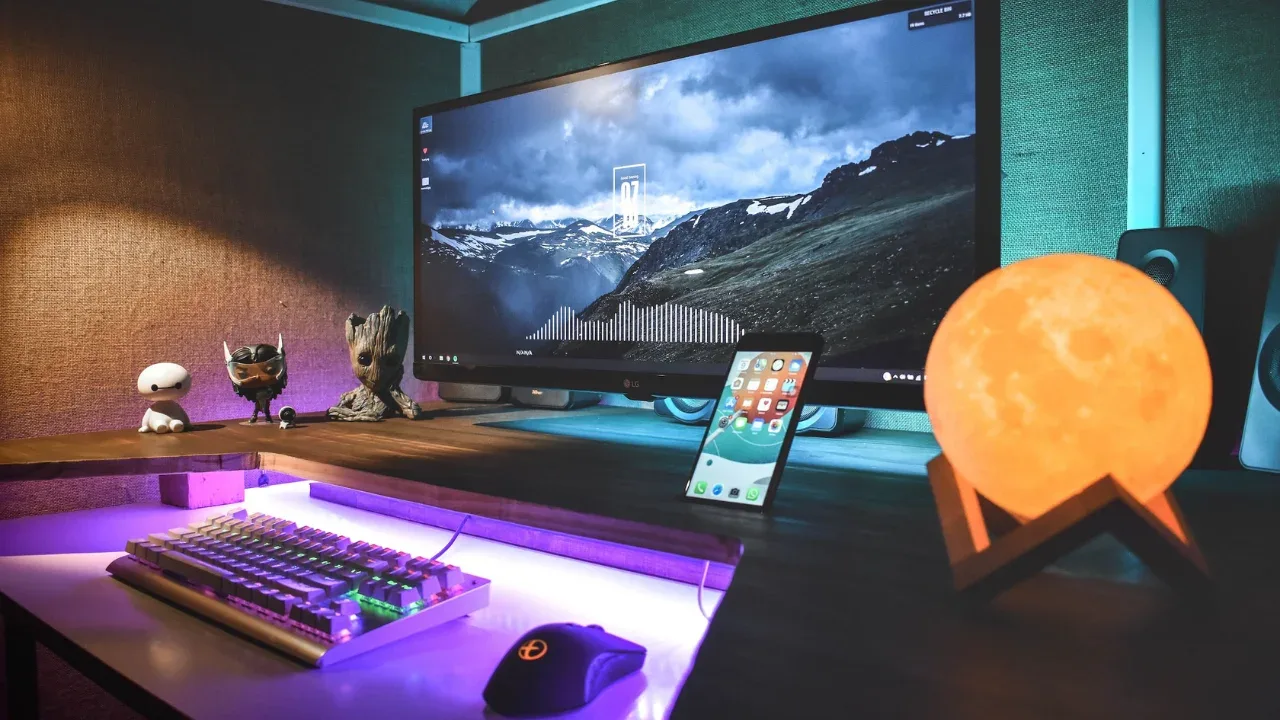
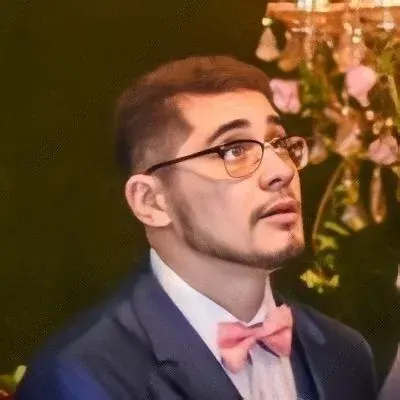
🕵️♂️ How to Find if an Array Contains a Specific String in JavaScript 🧩
Have you ever wondered how to check if a specific string is present in an array? If you're working with JavaScript or jQuery, you're in luck! In this blog post, we'll explore different methods to check if an array contains a specific string, and provide you with easy-to-understand solutions that you can try right away. 🚀
🤔 The Problem: Detecting if a Specific String is in the Array
Let's say you have an array of categories, and you want to find out if the string "specialword"
is present in the array. Here's an example to give you some context:
const categories = [
"specialword",
"word1",
"word2",
// ...
];
Now, the question is: how can we efficiently determine if "specialword"
is included in the categories
array, without manually searching through each element? Let's dive into the solutions below. 💡
🛠️ Solution 1: Using the includes()
Method in JavaScript
The easiest and most straightforward way to check if an array contains a specific string is to use the includes()
method in JavaScript. This method checks if an element is present in an array and returns either true
or false
.
Here's how you can use the includes()
method to solve our problem:
const hasSpecialWord = categories.includes("specialword");
if (hasSpecialWord) {
console.log("The array contains 'specialword'! 🎉");
} else {
console.log("Oops! 'specialword' is not found. 😔");
}
In the above code, the includes()
method is called on the categories
array, passing "specialword"
as the argument. If it returns true
, we log a success message; otherwise, we log a failure message.
🧰 Solution 2: Using a Loop to Check each Element
Another approach to find if a specific string is present in an array is to use a loop, such as a for
loop or the forEach()
method. This method is useful if you need to perform additional actions or gather information about the index of the element.
Here's an example that demonstrates the use of a for
loop:
let hasSpecialWord = false;
for (let i = 0; i < categories.length; i++) {
if (categories[i] === "specialword") {
hasSpecialWord = true;
break;
}
}
if (hasSpecialWord) {
console.log("The array contains 'specialword'! 🎉");
} else {
console.log("Oops! 'specialword' is not found. 😔");
}
In the above code, we initialize a boolean variable hasSpecialWord
to false
. Then, we iterate through each element of the array using a for
loop, checking if the element is equal to "specialword"
. If a match is found, we set hasSpecialWord
to true
and break out of the loop.
🌟 Wrap Up
Detecting if a specific string is present in an array is a common task when working with JavaScript or jQuery. In this blog post, we explored two simple yet powerful solutions to solve this problem: using the includes()
method and utilizing a loop.
Now that you have these techniques at your disposal, you can easily determine if a string exists in an array without any hassle. Give them a try and see how they work with your code! 🔍
If you found this blog post helpful, feel free to share it with your fellow developers and spread the knowledge. Should you have any questions or suggestions, please leave a comment below. Happy coding! 💻🎉