How to extend an existing JavaScript array with another array, without creating a new array
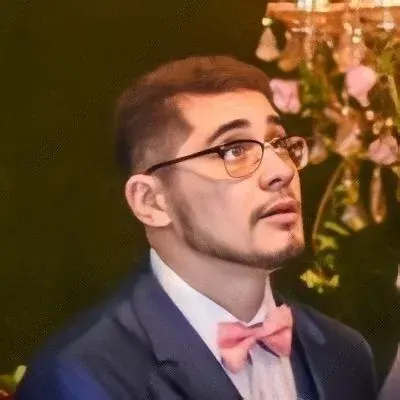
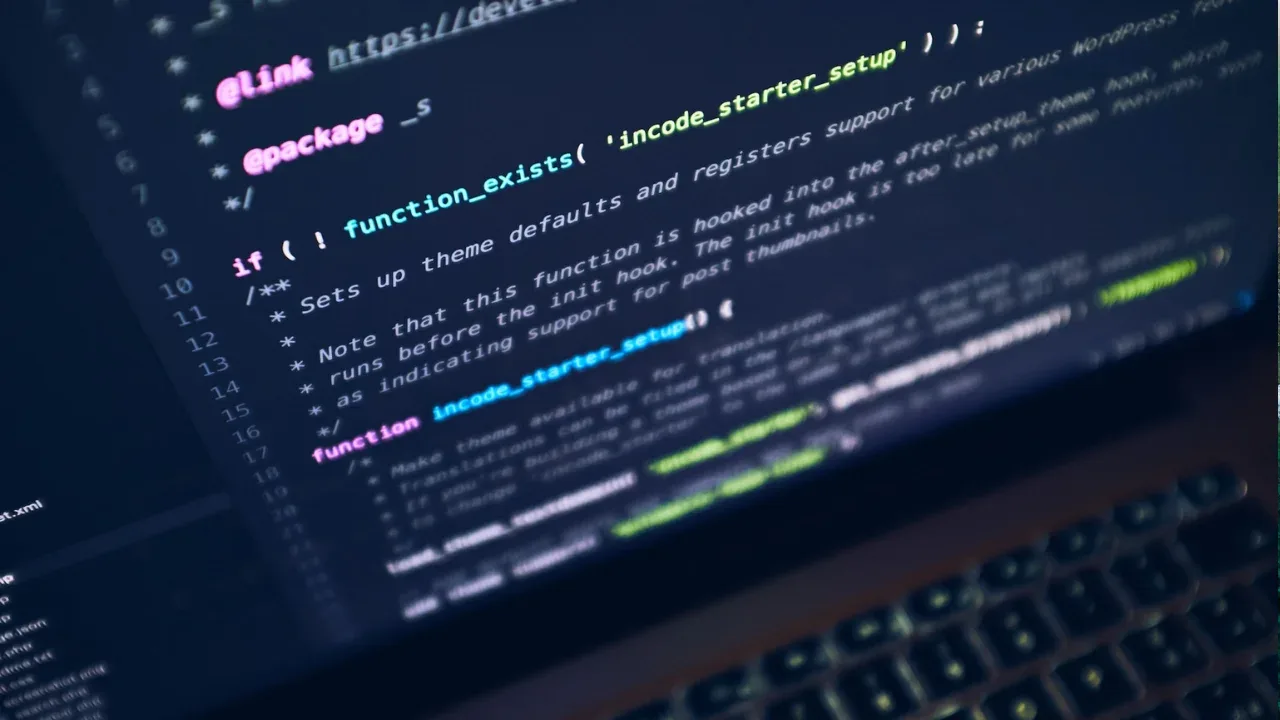
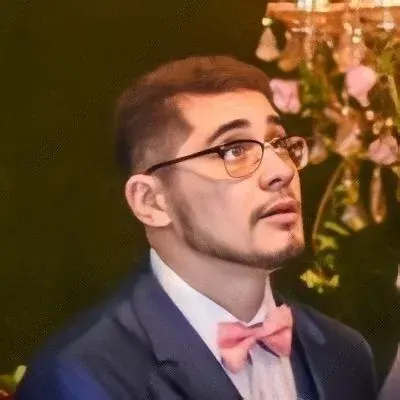
How to Extend an Existing JavaScript Array without Creating a New Array 🚀
Have you ever wanted to extend an existing JavaScript array with another array, without creating a new array? 🤔 If you have, you're not alone! Many developers have struggled with this challenge, especially when the first array is significantly larger than the second one.
In this blog post, we'll explore this problem and provide you with an efficient and easy solution. Let's dive in! 💪
The Challenge 👾
The goal is simple: we want to add all the elements from one array to another, without creating a new array. Think of it as emulating Python's extend
method. Here's an example to illustrate what we want to achieve:
let a = [1, 2];
let b = [3, 4, 5];
// After extending `a` with `b`, `a` should contain [1, 2, 3, 4, 5]
The Solution 💡
One might think that the JavaScript array has a built-in method to solve this problem, but unfortunately, there isn't one. However, fear not! We can leverage a little-known method in JavaScript: push.apply()
.
The push.apply()
method allows us to push multiple elements into an array. Here's how we can use it to extend an existing array:
let a = [1, 2];
let b = [3, 4, 5];
Array.prototype.push.apply(a, b);
console.log(a); // Output: [1, 2, 3, 4, 5]
In the code snippet above, we called push.apply()
on the prototype of the Array object. By passing in a
as the context and b
as the arguments, we were able to achieve our goal. Notice that the original array a
is modified in place.
Efficiency Matters ⚡
You might be wondering about the efficiency of this solution, especially when a
is significantly larger than b
. The good news is, this method is efficient because it doesn't involve copying the contents of a
. Instead, it directly modifies the existing array. This makes it a great option when performance is a concern.
Conclusion 🎉
Now you know how to extend an existing JavaScript array with another array without creating a new array. By using the push.apply()
method, you can efficiently achieve this without worrying about performance issues.
So go ahead, give it a try! Start extending your arrays like a pro. If you have any questions or alternative solutions, feel free to share them in the comments section below.
📢 What's your favorite way to extend JavaScript arrays? Let us know! 💬