How to escape regular expression special characters using javascript?
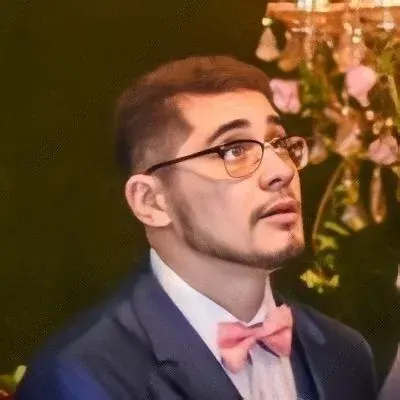
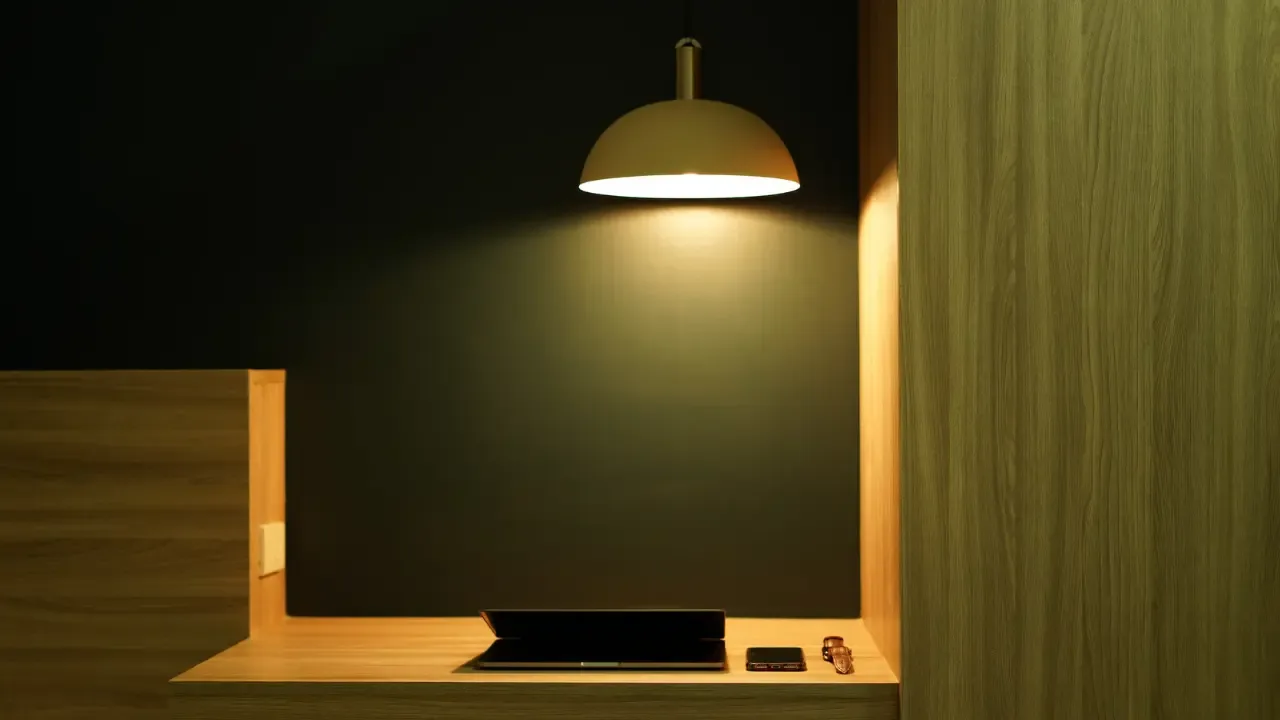
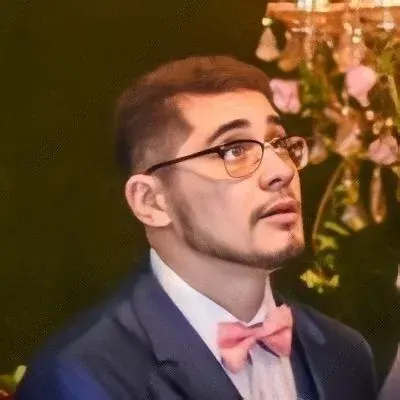
How to Escape Regular Expression Special Characters Using JavaScript: A Complete Guide
Hey there 👋! Are you having trouble escaping regular expression special characters in JavaScript? Don't worry, you're not alone! In this blog post, we'll walk through the common issues, provide easy solutions, and help you escape those pesky special characters like a pro. Let's dive in!
Understanding the Problem
Regular expressions are powerful tools for pattern matching in JavaScript. However, some characters have special meanings in regular expressions, such as /
, .
, *
, +
, ?
, (
, )
, [
, ]
, {
, }
, and \
. To treat these characters as literal characters and not as special characters, we need to escape them.
The Provided Code
In the code snippet you shared, it seems you're attempting to create a custom function called RegExp.escape
to escape all the special characters. While it's great to experiment and learn, there are simpler and more efficient ways to achieve this in JavaScript. Let's explore some of those methods!
Method 1: Using the RegExp.escape
Polyfill
The code you shared includes a polyfill for the RegExp.escape
method. This method adds an escape
function to the RegExp
object, making it easier to escape special characters in regular expressions. However, the implementation of this function is incorrect.
Here's a corrected version of the RegExp.escape
function:
RegExp.escape = function(str) {
return str.replace(/[.*+?^${}()|[\]\\]/g, '\\$&');
};
Method 2: Using the replace
Method
A simpler and cleaner approach is to use the built-in replace
method to escape the special characters. Here's an example of how you can escape the special characters in a given string:
function escapeRegexSpecialChars(str) {
return str.replace(/[.*+?^${}()|[\]\\]/g, '\\$&');
}
In this code snippet, we're using the replace
method with a regular expression that matches all the special characters. By replacing them with \\$&
, we're escaping them appropriately.
Testing the Solutions
To test both methods, you can modify your regExpFind
function as follows:
function regExpFind() {
var searchString = "[Munees]waran";
// Method 1
var escapedString1 = RegExp.escape(searchString);
console.log(escapedString1);
// Method 2
var escapedString2 = escapeRegexSpecialChars(searchString);
console.log(escapedString2);
}
By calling regExpFind()
, you'll see the escaped strings logged to the console. You can then use these escaped strings in your regular expressions without running into any issues caused by the special characters.
Conclusion
Escaping regular expression special characters in JavaScript is crucial when you want to treat them as literal characters in your patterns. Today, we explored two different methods: using the RegExp.escape
polyfill and applying the replace
method. Both approaches are simple and effective.
Now that you know how to escape those tricky special characters, go ahead and level up your pattern matching game in JavaScript! If you have any questions or face any issues, feel free to reach out to us. Happy coding! 😄🚀
Have you ever struggled with escaping regular expression special characters in JavaScript? Share your experiences and tips in the comments below! Let's learn from each other.