How to efficiently count the number of keys/properties of an object in JavaScript
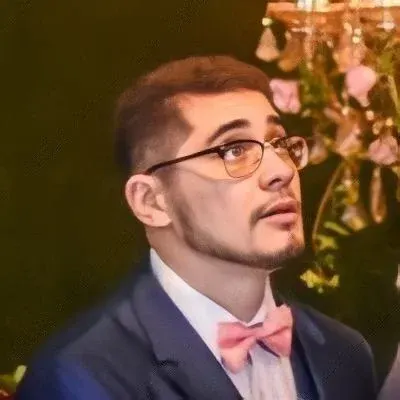
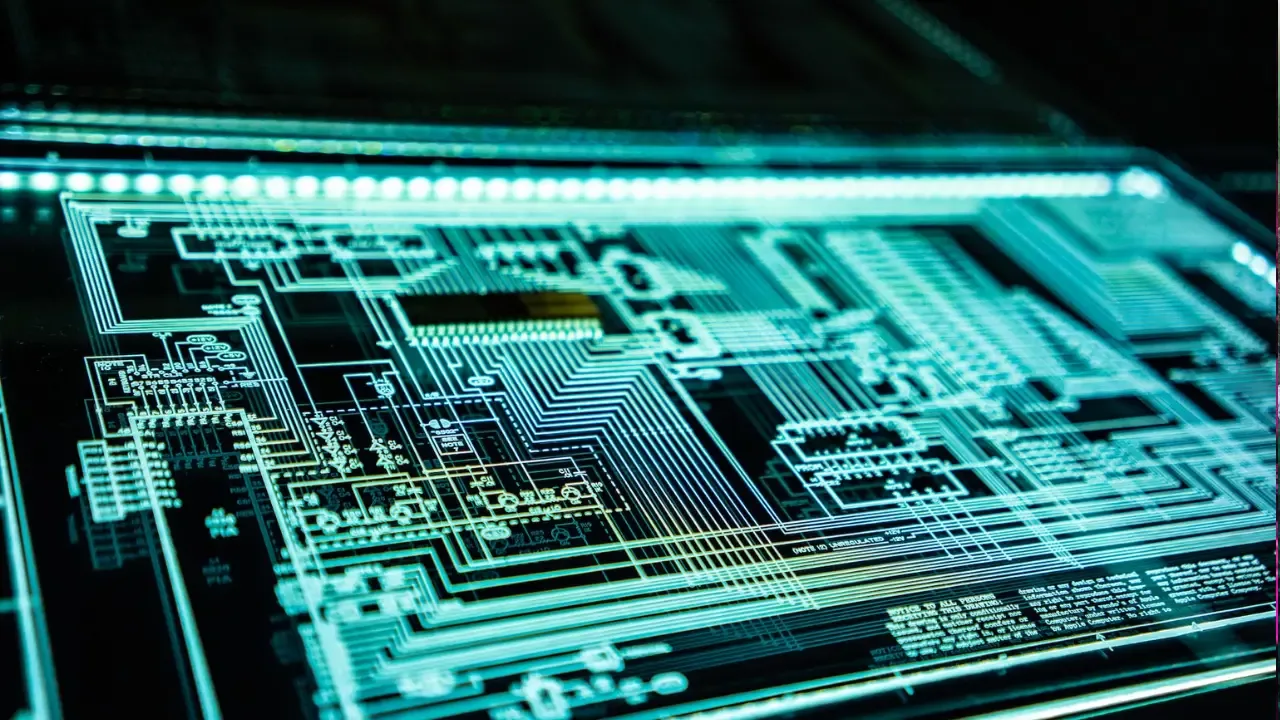
How to efficiently count the number of keys/properties of an object in JavaScript 😎🔢
So you want to count the number of keys/properties of an object in JavaScript, but you're wondering if there's a faster and more efficient way to do it without iterating over the object. Well, you're in luck because we've got some cool tricks up our sleeves! 🎩✨
The Traditional Approach 🔄
Before diving into alternative solutions, let's talk about the traditional approach, which involves iterating over the object using a for...in
loop. Here's an example:
var count = 0;
for (var key in myobj) {
if (myobj.hasOwnProperty(key)) {
count++;
}
}
This approach works perfectly fine, but it can be a bit slow for large objects or in situations where performance is critical.
The Object.keys()
Method 🔑
Fortunately, JavaScript provides us with a handy method called Object.keys()
that makes counting object properties a breeze! 🌬️💨
Here's how you can use it:
var count = Object.keys(myobj).length;
By using Object.keys()
, we can directly get an array of all the keys in the object and then simply retrieve its length. This eliminates the need for a loop and makes our code more concise and readable.
Compatibility Considerations 🚦
It's worth mentioning that Object.keys()
is supported in all modern browsers, including IE9+. However, if you're working with older browsers or need to support legacy code, you may encounter compatibility issues.
In those cases, you can use a polyfill or a library like Lodash, which provides a _.keys()
function that behaves similarly to Object.keys()
.
Wrapping Up 🎁
Counting the number of keys/properties of an object in JavaScript doesn't have to be a tedious task. With the Object.keys()
method, you can efficiently accomplish this in a single line of code! 🚀
Remember, the key (pun intended) to writing great code is knowing the right tools at your disposal. So give Object.keys()
a try and let us know what you think.
Have you encountered any unique challenges when working with object properties? How did you handle them? Share your experiences with us in the comments section below! ✍️💬
Keep learning, keep coding! 💪
PS: If you found this post helpful, don't forget to share it with your fellow developers! 🌟📣
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
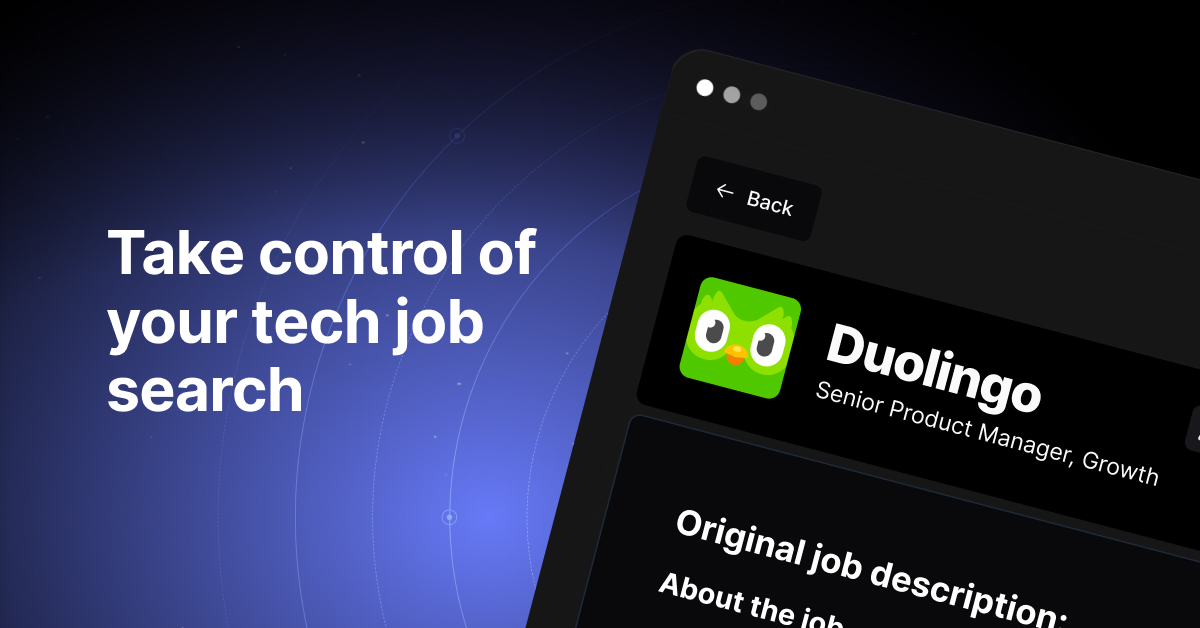