How to dynamically change a web page"s title?
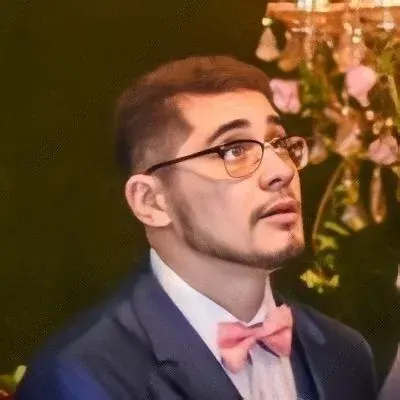
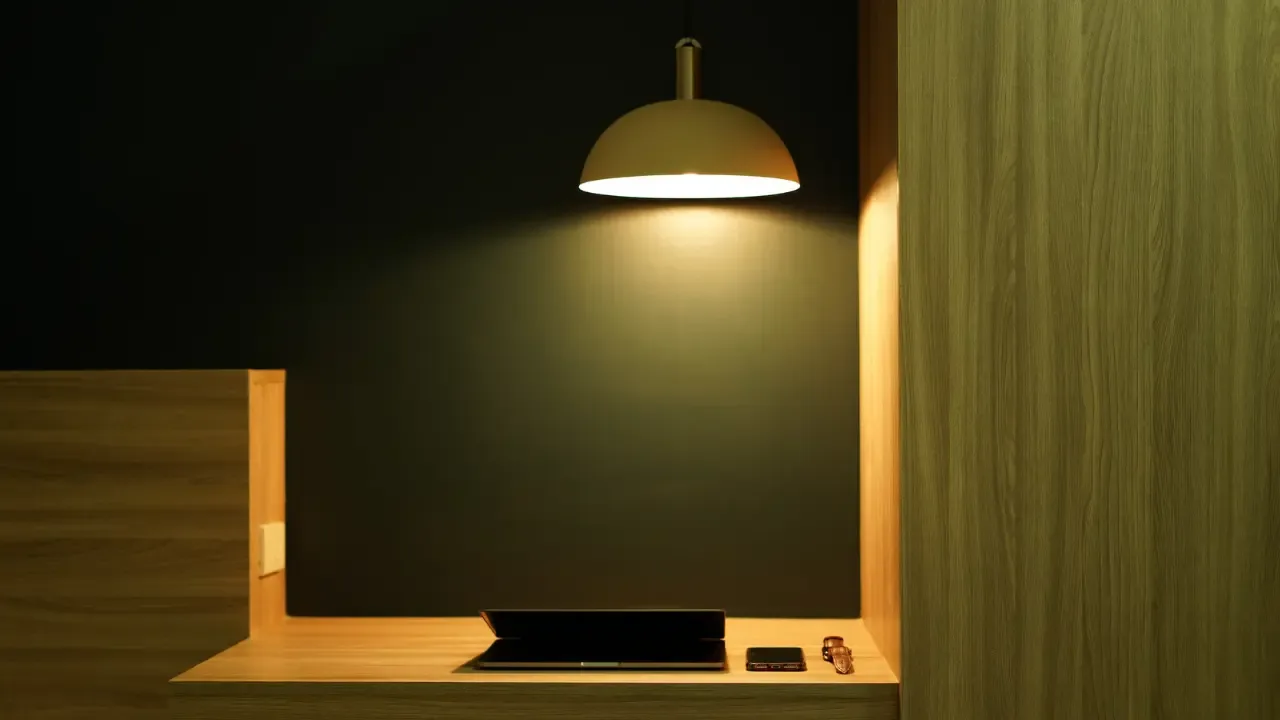
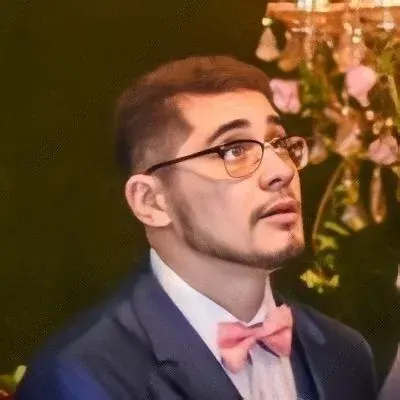
How to Dynamically Change a Web Page's Title with JavaScript 💻✨
Have you ever come across a situation where you need to update the title of a web page dynamically without refreshing it? Well, you're in luck! In this blog post, we'll explore a common issue faced by web developers and provide easy solutions on how to tackle it. So, let's dive right in! 🏊♀️
The Challenge ❓
The problem at hand is to change the page title whenever a tab is selected on a webpage. Traditionally, when a page is refreshed, the title can be easily modified using HTML's <title>
tag. However, in this scenario, we cannot rely on page refreshes. Instead, we need to update the title dynamically using JavaScript, all while maintaining a smooth user experience.
The Solution 💡
To dynamically change a web page's title without reloading it, we can utilize the power of JavaScript. Here's how you can achieve this:
HTML Setup: Make sure your HTML structure includes the necessary elements. In this case, we have a set of tabs with corresponding content as described in the context.
<!DOCTYPE html>
<html>
<head>
<title>My Web Page</title>
</head>
<body>
<div id="tabs">
<div class="tab" data-tab="tab1">Tab 1</div>
<div class="tab" data-tab="tab2">Tab 2</div>
<div class="tab" data-tab="tab3">Tab 3</div>
</div>
<div id="tab-content">
<div id="tab1">Content for Tab 1</div>
<div id="tab2">Content for Tab 2</div>
<div id="tab3">Content for Tab 3</div>
</div>
<script src="script.js"></script>
</body>
</html>
JavaScript Magic: Now, let's add some JavaScript logic to dynamically update the page title based on the selected tab.
// script.js
const tabs = document.querySelectorAll('.tab');
tabs.forEach((tab) => {
tab.addEventListener('click', () => {
const selectedTab = tab.getAttribute('data-tab');
document.title = `My Web Page - ${selectedTab}`;
});
});
By attaching a click event listener to each tab element, we can capture the selected tab's value and update the document's title using document.title
. Feel free to customize the title format according to your needs!
Voilà! 🎉
With just a few lines of code, you can dynamically change the page title without reloading the entire web page. Your users will be able to appreciate the seamless transition between tabs while search engine optimization (SEO) is also catered for. So, go ahead and try implementing this solution in your own projects! 💪
Your Turn! 🤔
Have you ever had to dynamically change the page title for any other use case? Share your experiences and any additional tips or tricks you have with our vibrant community in the comment section below! Let's learn from each other and level up our web development skills together! 👩💻👨💻
Remember to subscribe to our newsletter to stay up to date with the latest web development insights, and don't forget to share this blog post with your friends who might find it helpful. Sharing is caring! 💌
Happy coding! 😄✨