How to download a file with Node.js (without using third-party libraries)?
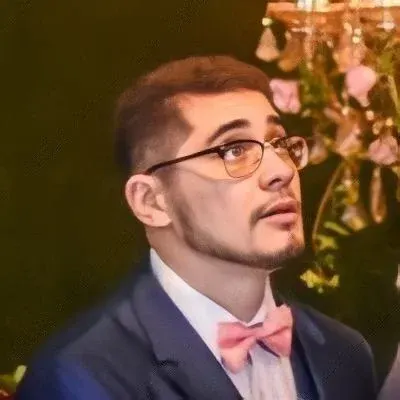
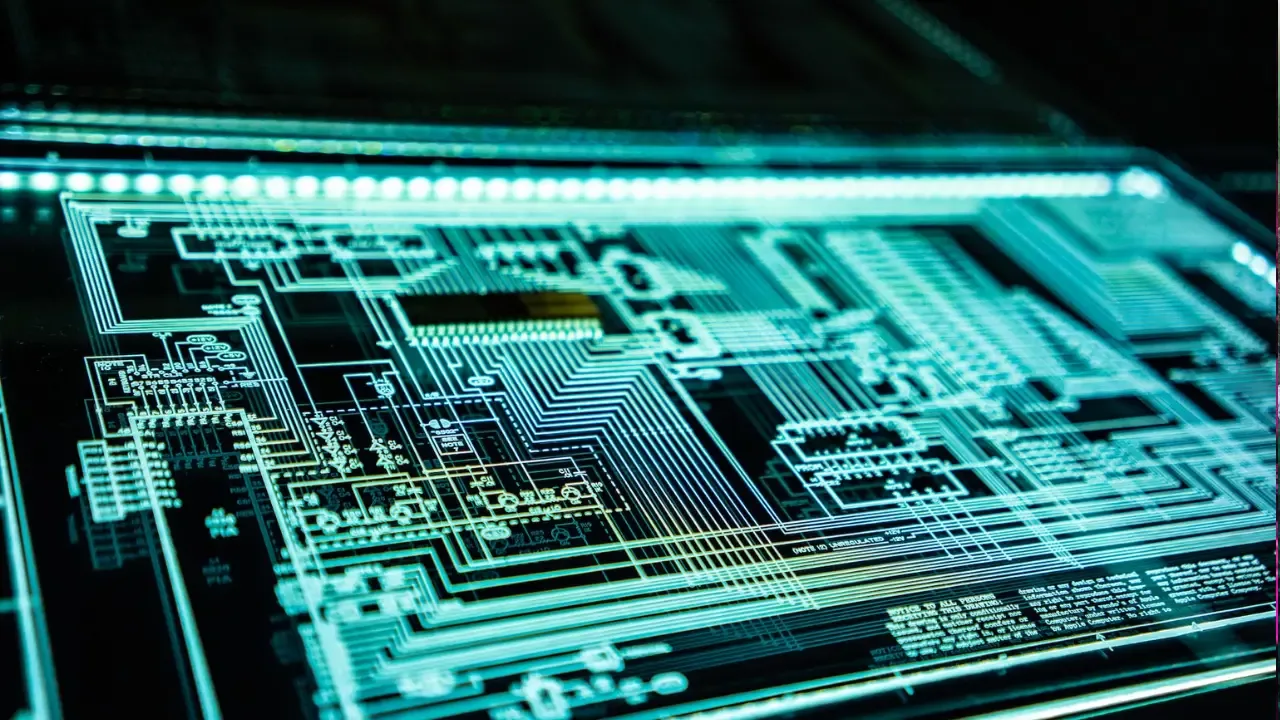
π How to Download a File with Node.js (Without Using Third-Party Libraries) π₯
So you want to download a file with Node.js, but you don't want to rely on any third-party libraries? No problem, we've got you covered! In this guide, we'll walk you through a simple and effective way to accomplish this task using pure Node.js.
π― Common Issue: Downloading a File without Dependencies
Before we dive into the solution, let's quickly address the common issue at hand. Many developers prefer to avoid third-party libraries due to concerns about security, performance, or simply to keep their codebase lightweight. If you find yourself in this boat, you're not alone!
π The Solution: Utilizing Native Node.js Modules
Node.js provides a number of native modules that make certain tasks, like file system operations, incredibly straightforward. We'll leverage two of these modulesβhttp
and fs
βto achieve our goal.
Here's a step-by-step breakdown of the process:
Import the necessary modules:
const http = require('http'); const fs = require('fs');
Define the URL of the file to download and the destination directory:
const fileUrl = 'https://example.com/path/to/file.ext'; const destinationDir = '/path/to/destination';
Create a writable stream to save the downloaded file:
const fileStream = fs.createWriteStream(`${destinationDir}/file.ext`);
Send an HTTP GET request to fetch the file:
http.get(fileUrl, (response) => { // Pipe the response stream into the file stream response.pipe(fileStream); });
Handle the completion of the download:
fileStream.on('finish', () => { console.log('File downloaded successfully!'); });
And that's it! π You've successfully downloaded a file using Node.js without any third-party libraries.
β‘οΈ Optional Tweaks and Enhancements
You can customize the
fileStream
variable to choose the desired file name or extension.You may want to handle potential errors that could occur during the download process. Consider adding an
error
event listener to thefileStream
object.For more complex scenarios, you might need to handle redirects or utilize authentication headers. In that case, you can explore the
http.request
method provided by the nativehttp
module.
πͺ Call-to-Action: Share Your Experience!
We hope this guide has helped you successfully download files with Node.js, without the need for any third-party libraries. Now it's your turn to put it into action! Share your experience and let us know if you encounter any issues or have any additional questions.
Happy downloading! π
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
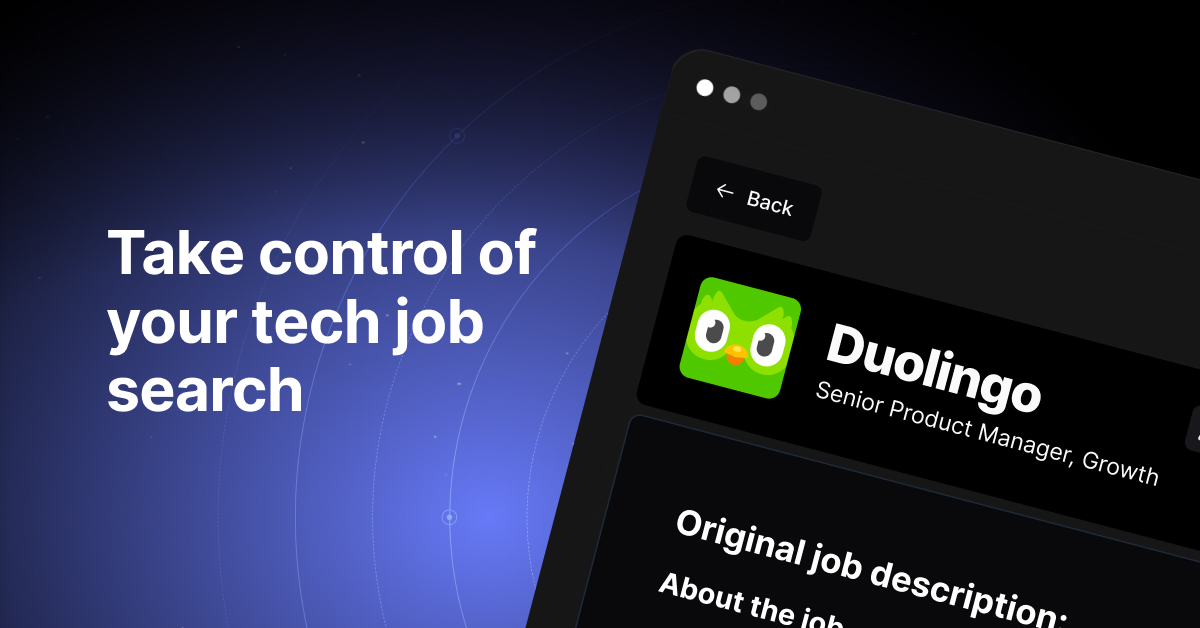