How to do case insensitive string comparison?
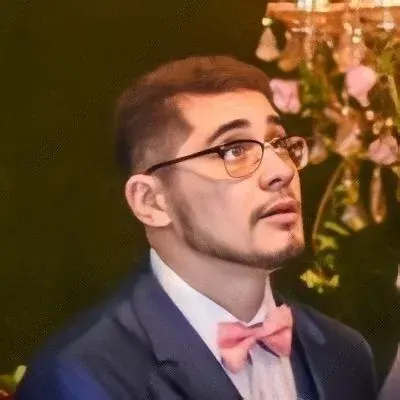
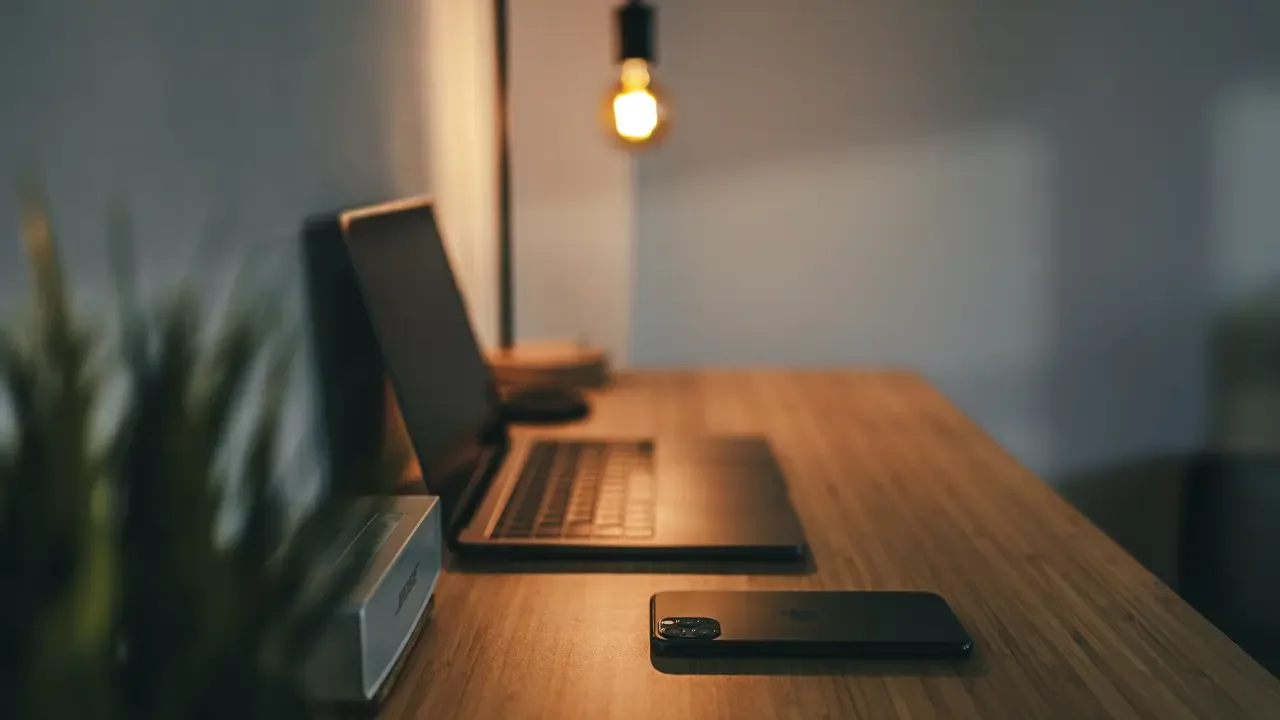
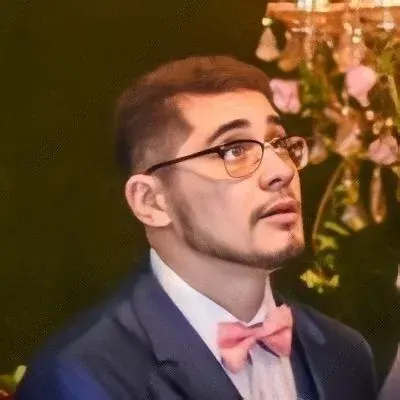
How to do Case Insensitive String Comparison in JavaScript 🤔🔄📝
JavaScript is a powerful programming language that allows developers to create dynamic and interactive web applications. However, when working with strings, one common issue that can arise is the need to perform case-insensitive string comparison.
Understanding the Problem 🤷♂️
String comparison in JavaScript is by default case-sensitive. This means that when comparing two strings, the comparison is made considering the character casing. For example, the strings "hello" and "Hello" would be considered different.
Common Issues 😩
However, in some cases, we may want to compare strings ignoring the casing, such as when validating user inputs like email addresses or usernames. This can lead to confusion and potential bugs if not handled correctly.
Easy Solutions 💡
Luckily, JavaScript provides us with several simple solutions to overcome this challenge. Let's explore some of the most common ones:
1. Using the toLowerCase() method
One way to perform case-insensitive string comparison is to convert both strings to lowercase using the toLowerCase()
method before comparing them. This ensures that the casing is ignored.
const string1 = "hello";
const string2 = "Hello";
if (string1.toLowerCase() === string2.toLowerCase()) {
console.log("Strings are equal (case-insensitive)");
} else {
console.log("Strings are not equal (case-insensitive)");
}
In the example above, both string1
and string2
are converted to lowercase using the toLowerCase()
method. Then, the comparison can be made without considering the casing.
2. Using the localeCompare() method with options
Another approach is to use the localeCompare()
method, which allows us to specify options for the comparison. By setting the sensitivity
option to "base"
, we can perform a case-insensitive string comparison.
const string1 = "hello";
const string2 = "Hello";
if (string1.localeCompare(string2, undefined, { sensitivity: "base" }) === 0) {
console.log("Strings are equal (case-insensitive)");
} else {
console.log("Strings are not equal (case-insensitive)");
}
In this example, the localeCompare()
method is used with the sensitivity
option set to "base"
. This ensures that the comparison is made without considering the casing.
Conclusion and Call-to-Action 🎉✍️💬
Performing case-insensitive string comparison in JavaScript is a common need, especially when dealing with user inputs. By using the toLowerCase()
method or the localeCompare()
method with the appropriate options, you can easily overcome this challenge and avoid potential bugs.
Next time you encounter a situation where case-insensitive string comparison is needed, remember these easy solutions and ensure your code works flawlessly.
Have you ever faced issues with case-insensitive string comparison? What other JavaScript topics would you like to read about? Let us know in the comments below! Happy coding! 😄🚀
Stay updated with our latest articles by subscribing to our newsletter and follow us on social media channels for more insightful content.