How to display length of filtered ng-repeat data
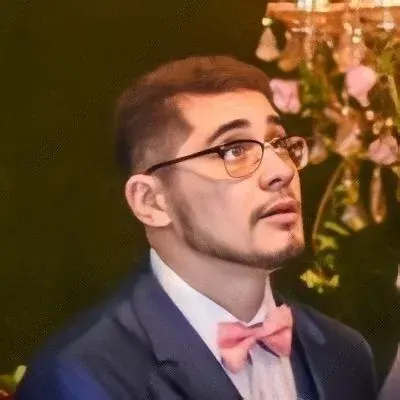
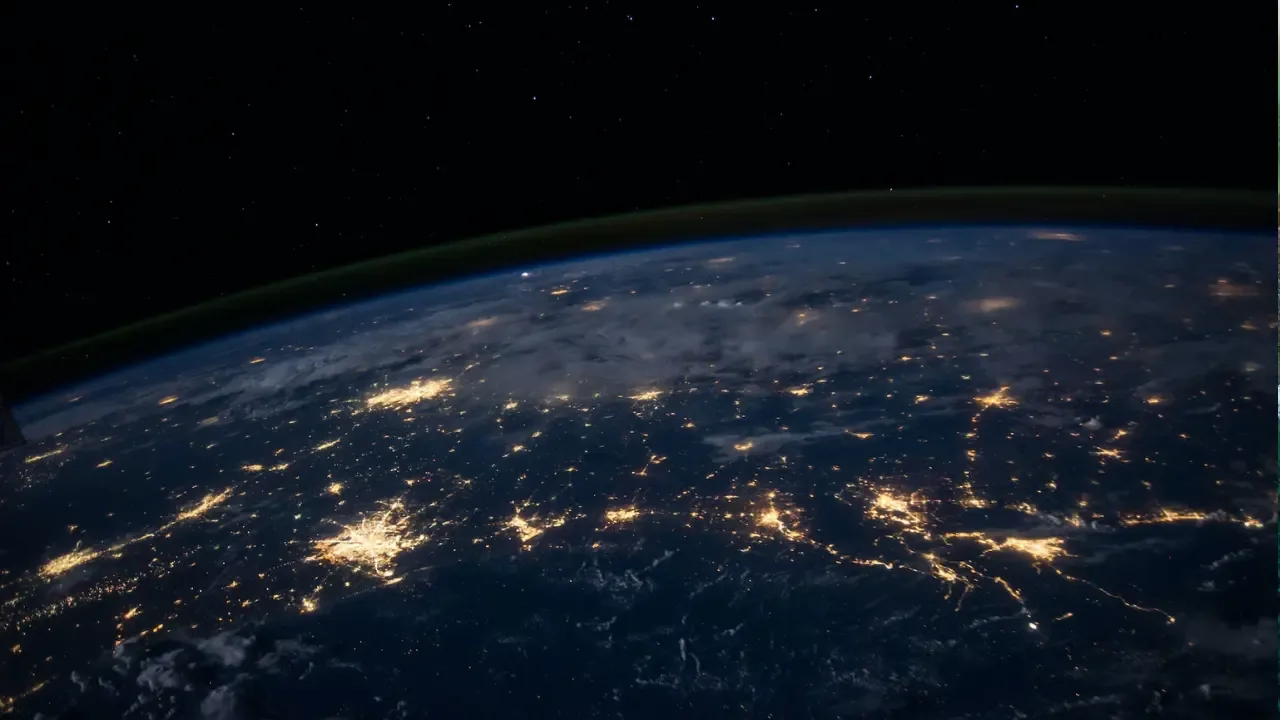
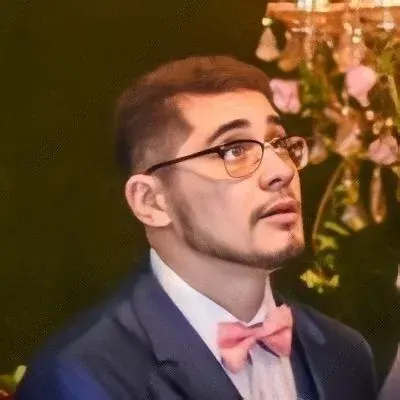
📝 How to Display the Length of Filtered ng-repeat Data
Hey there tech enthusiasts! 👋 Are you stuck on how to display the length of filtered ng-repeat data in your AngularJS application? Don't worry, we've got you covered! In this blog post, we'll address this common issue and provide you with easy solutions.
Let's dive right in! 😎
The Problem
So, you have a data array that contains multiple objects in JSON format. You use the ng-repeat directive to display this data on your UI, and you also have an input field where users can search and filter the data. However, when the data is filtered, the count of displayed objects does not update. 😫
The Solution
To solve this issue and display the count of filtered data, follow these simple steps:
Step 1: Set up the Filter
Make sure you have set up the filter correctly in your ng-repeat directive. In your HTML code, it should look something like this:
<div ng-repeat="person in data | filter: query">
<!-- Display person details here -->
</div>
Step 2: Add a Filtered Data Array
Create a new variable in your controller to store the filtered data. We'll call it filteredData
for this example. Initialize it with the original data array.
$scope.filteredData = $scope.data;
Step 3: Watch for Changes
Now, we need to watch for changes in the query
value and update the filteredData
array accordingly. Add the following code to your controller:
$scope.$watch('query', function(newValue, oldValue) {
if (newValue !== oldValue) {
$scope.filteredData = $filter('filter')($scope.data, $scope.query);
}
});
Here, we use the $watch
function to detect changes in the query
value. If the new value is different from the old value, we update the filteredData
array using the $filter
service.
Step 4: Display the Filtered Data Count
Finally, update your UI to display the count of filtered data. Use the filteredData.length
property to get the length of the filtered array.
<p>Showing {{filteredData.length}} Persons</p>
Voila! 🎉 Now, the count of displayed objects will update dynamically as the user filters the data.
Call-to-Action
We hope this guide helped you solve the issue of displaying the length of filtered ng-repeat data. If you found this blog post useful, make sure to share it with your fellow developers who might be facing the same problem. Remember, sharing is caring! ❤️
If you have any other AngularJS or tech-related questions, feel free to drop them in the comments section below. Let's keep the tech community thriving! 💪
Happy filtering! ✨