How to determine if a JavaScript array contains an object with an attribute that equals a given value
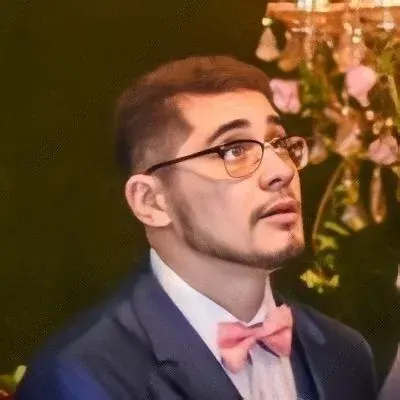
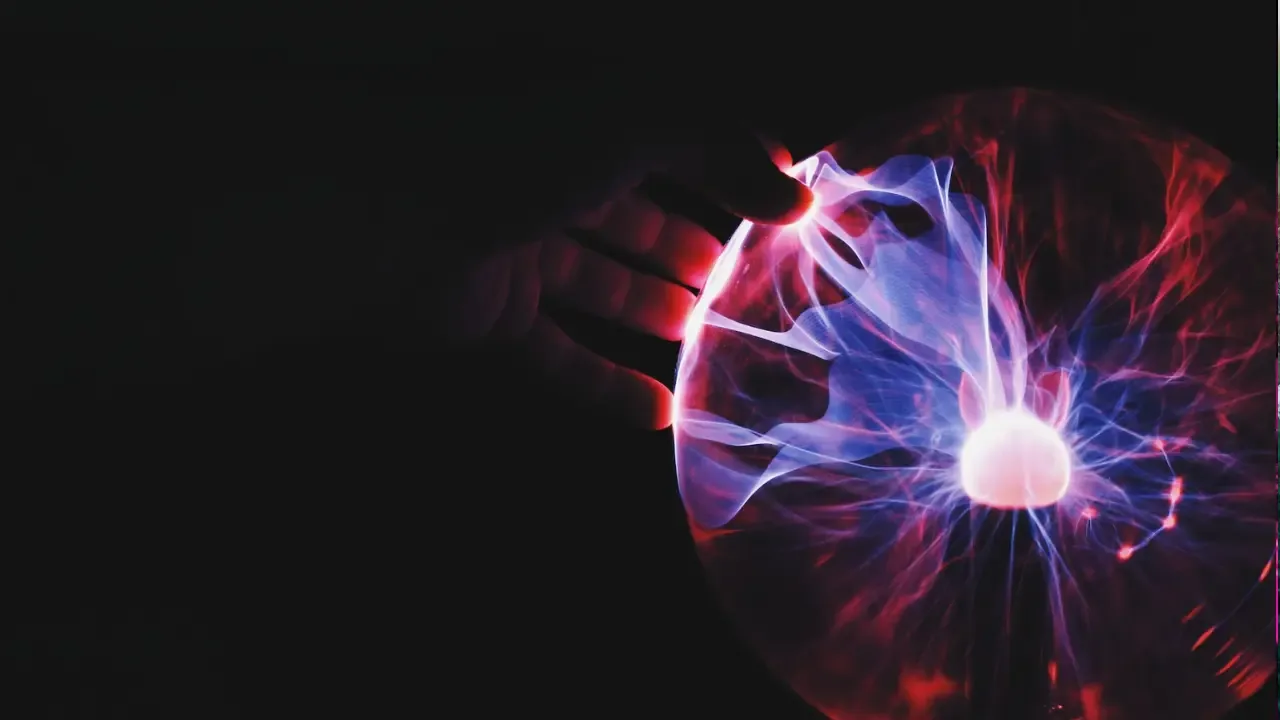
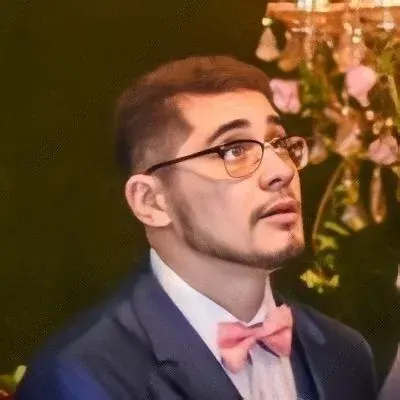
🤔 How to determine if a JavaScript array contains an object with an attribute that equals a given value
So you have an array of objects in JavaScript, and you want to check if there is an object in the array that has a specific attribute value. You're also concerned about performance since you might have a large number of records to check. Let's explore some solutions for this problem!
💡 Solution 1: The Array.prototype.find()
method
If you want a clean and concise solution, you can use the Array.prototype.find()
method. This method returns the first element in the array that satisfies the provided testing function. Here's how you can use it to determine if an object with a specific attribute value exists:
const vendors = [
{
Name: 'Magenic',
ID: 'ABC'
},
{
Name: 'Microsoft',
ID: 'DEF'
},
// ... and so on ...
];
const vendorExists = vendors.find(vendor => vendor.Name === 'Magenic') !== undefined;
console.log(vendorExists); // Outputs: true
🔍 Here's how it works:
The
Array.prototype.find()
method searches the arrayvendors
for an object that satisfies the condition specified by the arrow functionvendor => vendor.Name === 'Magenic'
.If a matching object is found, the
find()
method returns that object. Otherwise, it returnsundefined
.By checking if the returned value is not equal to
undefined
, we can determine if the object exists in the array.
✅ This approach allows you to find the object you're looking for without having to loop through the entire array. It stops as soon as it finds the first matching object.
⚡ Solution 2: The Array.prototype.some()
method
Another powerful method you can use is Array.prototype.some()
. This method tests whether at least one element in the array satisfies the provided testing function. Here's how you can use it:
const vendors = [
{
Name: 'Magenic',
ID: 'ABC'
},
{
Name: 'Microsoft',
ID: 'DEF'
},
// ... and so on ...
];
const vendorExists = vendors.some(vendor => vendor.Name === 'Magenic');
console.log(vendorExists); // Outputs: true
🔍 Here's how it works:
The
Array.prototype.some()
method tests each object in the arrayvendors
against the condition specified by the arrow functionvendor => vendor.Name === 'Magenic'
.It stops as soon as it finds an object that satisfies the condition and returns
true
. If no objects satisfy the condition, it returnsfalse
.
✅ This approach is similar to Array.prototype.find()
, but instead of finding the object, it focuses on determining if at least one object satisfies the condition.
🚀 Call-to-Action: Share your thoughts and engage with us!
Now that you have learned two ways to determine if a JavaScript array contains an object with an attribute that equals a given value, why not put your knowledge into action?
👉 Do you have any other solutions to this problem? Share them in the comments below! We would love to hear from you.
👉 If you found this blog post helpful, consider sharing it with your friends and colleagues. It might help them too!
Happy coding! 💻✨