How to detect if URL has changed after hash in JavaScript
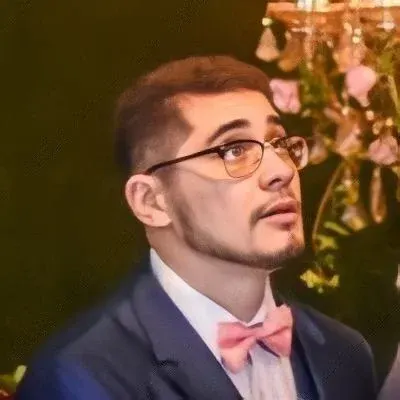
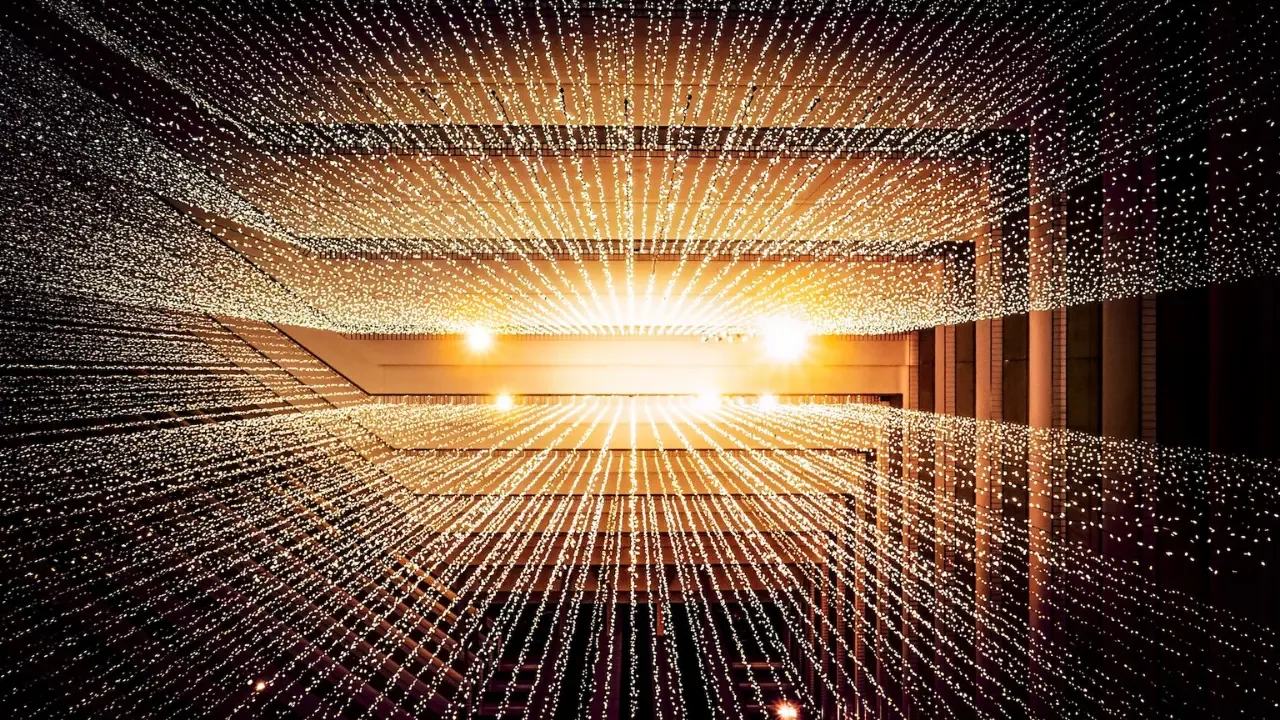
How to Detect if URL has Changed after Hash in JavaScript ๐ก๐
Have you ever wondered how the websites like GitHub dynamically change the URL without reloading the entire page? ๐ค They cleverly use the hash symbol "#" to append page information, allowing them to create a unique URL for each page. But how can we detect if this URL has changed using JavaScript? ๐
In this blog post, we will explore some common issues and provide easy solutions to detect URL changes after the hash. Let's dive in! ๐โโ๏ธ
Issue 1: Is the onload
Event Called Again? ๐
One might think that if the URL changes, the onload
event will be called again. However, this is not the case. The onload
event is only triggered once when the page is initially loaded. So, relying solely on onload
won't help us detect URL changes after the hash. ๐
Issue 2: Is there an Event Handler for the URL? ๐๏ธ
Although JavaScript doesn't provide a dedicated event for URL changes after the hash, we can utilize the onhashchange
event handler. This event is triggered whenever the hash portion of the URL changes. ๐
Here's an example of how you can use the onhashchange
event to detect URL changes:
window.onhashchange = function() {
// Code to handle URL change after the hash
console.log("URL has changed!");
}
By attaching the event handler to the window
object, we can capture the URL changes whenever the hash is modified. ๐ฃ
Issue 3: Checking the URL Every Second โฐ
Checking the URL every second might be a possible solution, but it's not efficient and could lead to performance issues. Additionally, constantly polling the URL can drain the device's battery on mobile devices. We need a better alternative! ๐
Solution: Using the onhashchange
Event ๐
As mentioned earlier, the onhashchange
event is the key to detecting URL changes after the hash. Instead of continuously checking the URL, we can rely on this event to handle the changes dynamically. Here's an example:
window.onhashchange = handleURLChange;
function handleURLChange() {
// Code to handle URL change after the hash
console.log("URL has changed!");
}
By defining a specific function to handle the URL changes, we can keep our code clean and organized. ๐งน
Engage with Us! ๐๐ฃ
Did you find this guide helpful in detecting URL changes after the hash in JavaScript? Have you faced any other challenges related to URL manipulation? We would love to hear your thoughts and experiences! ๐ฌ๐ค
Feel free to leave a comment below and let us know how you handle URL changes in your JavaScript projects. Don't forget to share this post with your friends and colleagues who might find it useful. Together, we can master URL manipulation in JavaScript! ๐๐
Stay tuned for more insightful blog posts and tutorials. Happy coding! ๐ป๐
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
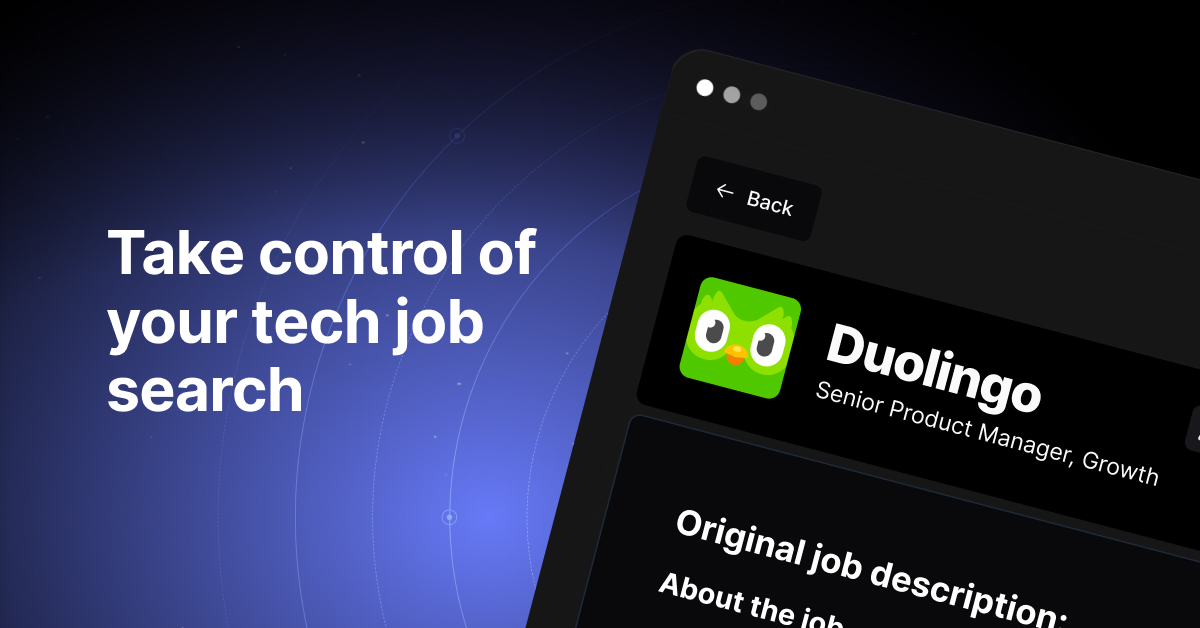