How to detect escape key press with pure JS or jQuery?
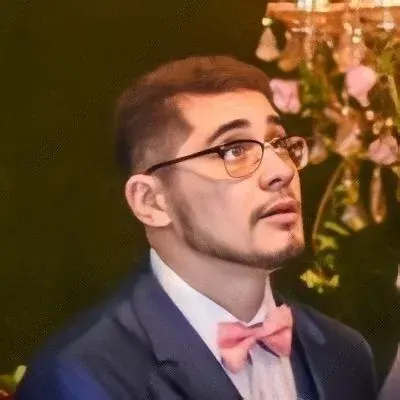
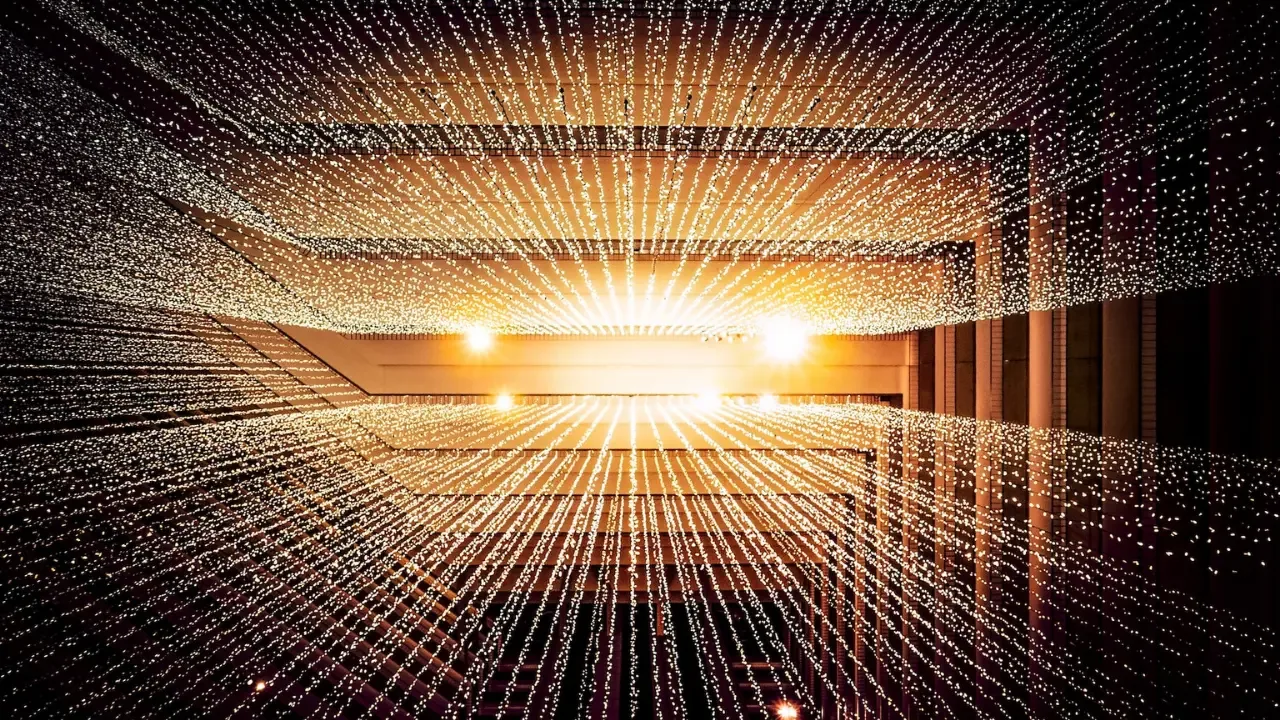
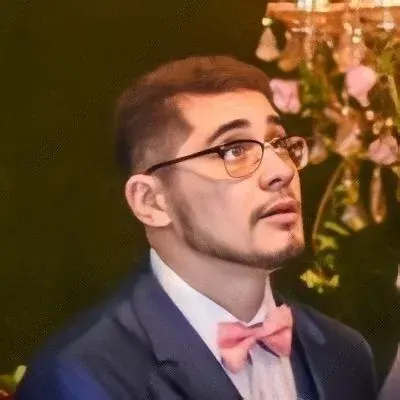
How to Detect Escape Key Press with Pure JS or jQuery?
Are you struggling to detect the escape key press in different browsers like IE, Firefox, and Chrome? We have got you covered! In this blog post, we will address this common issue and provide you with easy solutions using both pure JavaScript and jQuery. So, let's dive right in!
The first thing you need to understand is that different browsers have different ways of handling key events. This is why the code you mentioned may not work consistently across all browsers. But don't worry, we have some handy solutions for you.
Pure JavaScript Solution
document.addEventListener('keydown', function(event) {
if (event.key === "Escape" || event.key === "Esc") {
// Close your modal window or perform any desired action
}
});
In this solution, we use the keydown
event to detect when a key is pressed. We then check if the key that was pressed is either "Escape" or "Esc". If it matches, you can implement your desired action, such as closing a modal window.
jQuery Solution
$(document).on('keydown', function(e) {
if (e.key === "Escape" || e.key === "Esc") {
// Close your modal window or perform any desired action
}
});
For those who prefer using jQuery, we have an alternative solution. We use the keydown
event and check for the same key values as in the pure JavaScript solution. Again, when a match is found, you can close your modal window or perform any other necessary action.
Cross-Browser Solution
If you want a solution that works consistently across all major browsers, including IE, Firefox, and Chrome, you can combine both approaches:
function onEscapeKey(event) {
if (event.key === "Escape" || event.key === "Esc" || event.keyCode === 27) {
// Close your modal window or perform any desired action
}
}
document.addEventListener('keydown', onEscapeKey);
$(document).on('keydown', onEscapeKey);
By combining both pure JavaScript and jQuery solutions, you can ensure that your code handles the escape key press reliably across different browsers.
Conclusion
Detecting the escape key press may seem like a simple task, but it can become tricky when dealing with different browsers. However, with the solutions provided in this blog post, you can easily detect the escape key press using either pure JavaScript or jQuery. Remember to choose the solution that best fits your project and enjoy consistent cross-browser functionality.
If you have any questions or other key-related issues you'd like assistance with, let us know in the comments below. Happy coding! 💻🚀
Note: This post was inspired by a question on the Stack Overflow community. You can find the original question here.