How to detect DIV"s dimension changed?
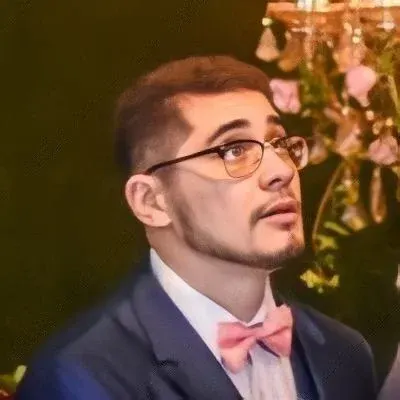
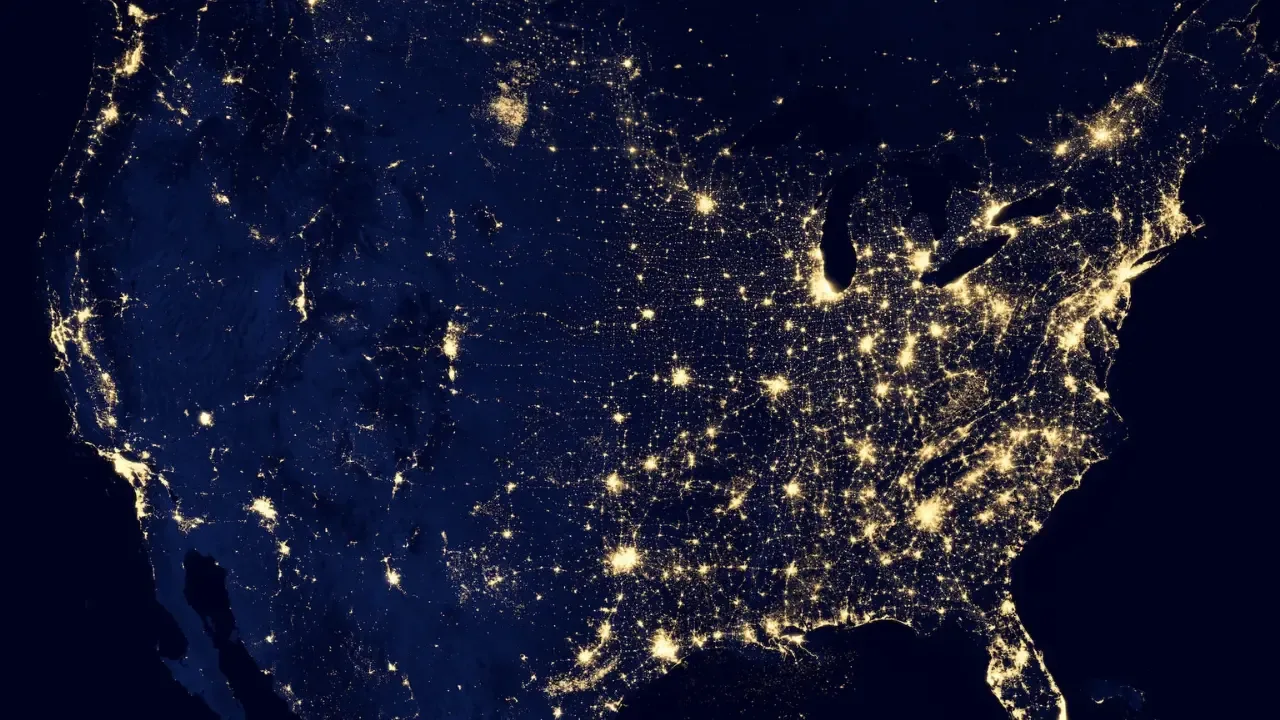
How to Detect DIV's Dimension Change - A Complete Guide
So you have a DIV element with a 100% width, and you want to detect when its dimension changes. Maybe you have some inner elements that get repositioned, causing the DIV's size to change. In this blog post, we'll explore the common issues around detecting DIV dimension changes and provide you with easy solutions to tackle this problem. 😎
The Challenge of Detecting DIV Dimension Changes
Detecting changes in a DIV's dimension can be tricky because the browser does not provide a built-in event for this specific purpose. However, there are a few workarounds that you can use to achieve the desired result.
Approach 1: jQuery's Resize Event
In the context you provided, you've tried using jQuery's resize event, but it doesn't seem to be working as expected. Let's dive into this approach and see what might be the issue.
The code snippet you shared includes a DIV with the id "test_div" and a jQuery script that binds a callback function to the resize event of this DIV. However, the resize event is not triggered for DIV elements by default, hence no console log is outputted.
<html>
<head>
<script type="text/javascript" language="javascript" src="http://code.jquery.com/jquery-1.6.1.min.js"></script>
<script type="text/javascript" language="javascript">
$('#test_div').bind('resize', function(){
console.log('resized');
});
</script>
</head>
<body>
<div id="test_div" style="width: 100%; min-height: 30px; border: 1px dashed pink;">
<input type="button" value="button 1" />
<input type="button" value="button 2" />
<input type="button" value="button 3" />
</div>
</body>
</html>
Easy Solution: Create Your Own Resize Event Listener
To detect changes in a DIV's dimension, we can create our own custom resize event listener using JavaScript and DOM APIs. Here's a step-by-step guide on how to achieve it:
First, let's give our DIV a specific class name for easy identification. Replace the line
<div id="test_div"...
with<div id="test_div" class="resizable"...
.Next, add the following JavaScript code after binding the callback function to the resize event:
$(window).on('resize', function() {
$('.resizable').each(function() {
var currentWidth = $(this).width();
var currentHeight = $(this).height();
var storedWidth = $(this).data('lastWidth');
var storedHeight = $(this).data('lastHeight');
if (currentWidth !== storedWidth || currentHeight !== storedHeight) {
// Dimension has changed, do something here
console.log('resized');
// Update the stored width and height
$(this).data('lastWidth', currentWidth);
$(this).data('lastHeight', currentHeight);
}
});
});
What this code does is listen for the window's resize event, iterate over all DIV elements with the class "resizable", and compare their current width and height with the previously stored values. If the dimensions have changed, the code executes the desired behavior (in this case, logging 'resized' to the console) and updates the stored width and height values.
Wrapping Up
And there you have it! A simple yet effective way to detect changes in a DIV's dimension. By creating your own resize event listener, you can now be notified whenever the DIV's size changes. Remember to customize the code snippet to suit your specific needs and desired actions.
If you found this guide helpful or have additional questions, feel free to leave a comment below. We'd love to hear from you and see how you're tackling DIV dimension change detection. Happy coding! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
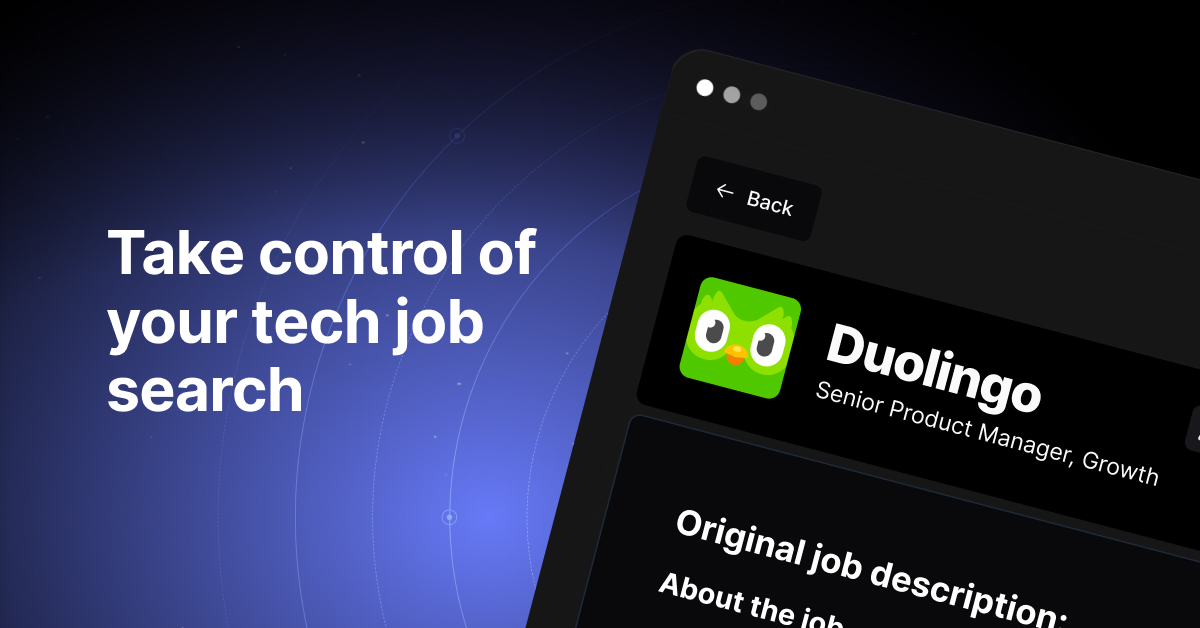