How to detect a mobile device using jQuery
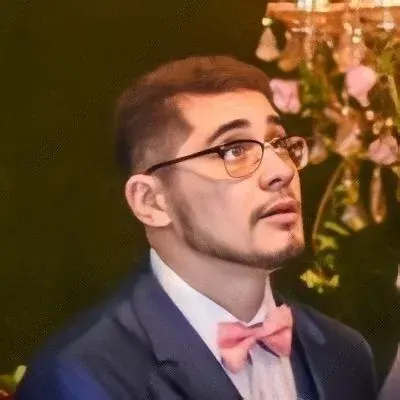
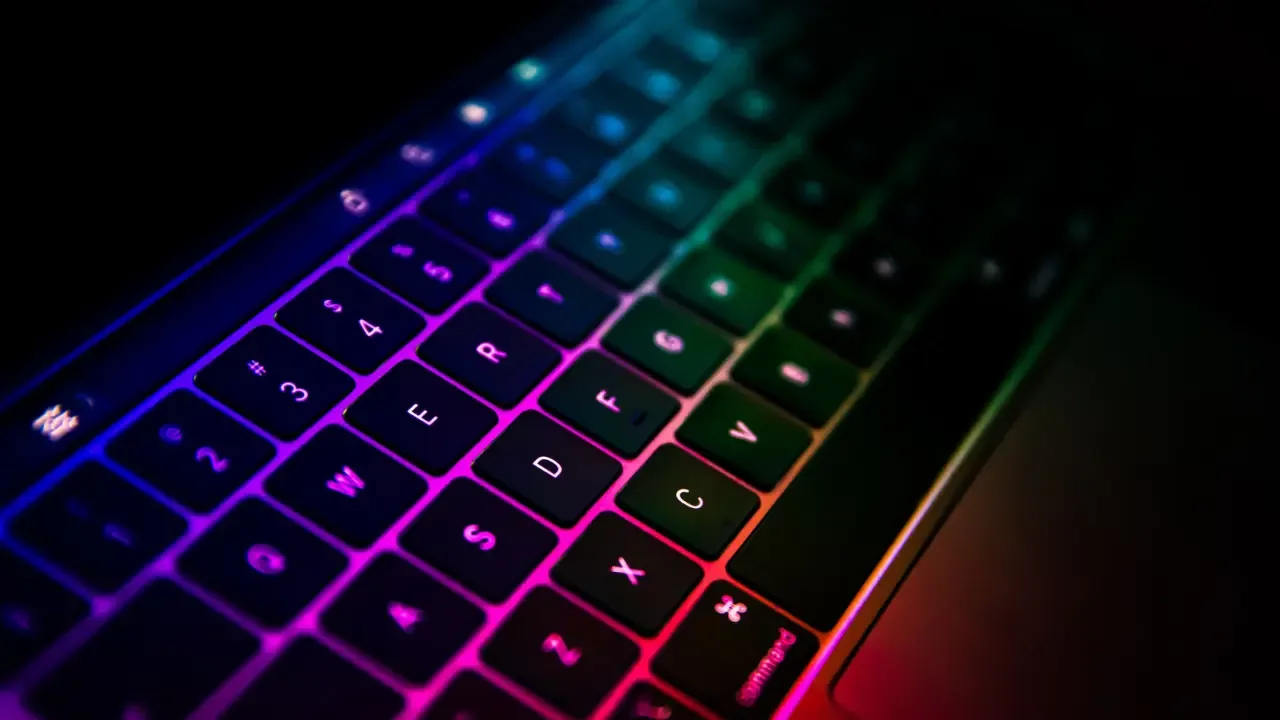
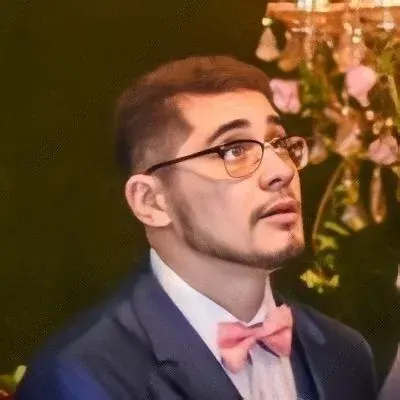
📱 How to Detect a Mobile Device Using jQuery 📱
Are you tired of running the same script on both desktop and mobile browsers? Do you want to optimize your website for a better user experience on handheld devices? Well, you're in luck! In this blog post, we'll explore how to detect a mobile device using jQuery and provide easy solutions to common issues.
🧐 The Problem: Detecting a Mobile Device
Traditionally, CSS media queries have been the go-to solution for detecting the type of device accessing your website. However, when it comes to running different scripts based on the device, we need something more powerful. That's where jQuery comes into play!
💡 The Easy Solution: Using jQuery to Detect Mobile Devices
To detect a mobile device using jQuery, we can leverage the navigator.userAgent
property. This property provides information about the user's browser, including the device they are using. Here's an example code snippet:
// Check if the user is on a mobile device
if (/Mobi|Android/i.test(navigator.userAgent)) {
// Run your mobile-specific script here
console.log('Running mobile script');
} else {
// Run your desktop-specific script here
console.log('Running desktop script');
}
🔎 Explaining the Code
Let's break down the code snippet to better understand how it works:
The
navigator.userAgent
property returns a string containing the user agent header sent by the browser.We use the
test()
function with a regular expression to match the string against patterns indicating mobile devices. In this example, we're checking for the strings "Mobi" and "Android", which are commonly found in theuserAgent
string for mobile devices.If a match is found, we can assume that the user is on a mobile device and run our mobile-specific script. Otherwise, we'll run our desktop-specific script.
💪 The Compelling Call-to-Action: Engage and Share!
Now that you know how to detect a mobile device using jQuery, it's time to put your newfound knowledge into action! Try implementing this technique in your projects and see the difference it makes. Don't forget to share your success stories and any additional tips or solutions you discover along the way. Let's create a community where we can all learn and grow together! 🌟
🔗 Conclusion
Detecting a mobile device using jQuery opens up a world of possibilities for optimizing your website's performance and user experience. By leveraging the navigator.userAgent
property and a simple code snippet, you can easily run different scripts based on the device accessing your site. So go ahead, give it a try, and elevate your web development game to the next level!
📣 Now, over to you! Have you ever encountered challenges while detecting mobile devices? How did you solve them? Share your thoughts and experiences in the comments below and let's keep the conversation going! 🎉