How to delete an item from state array?
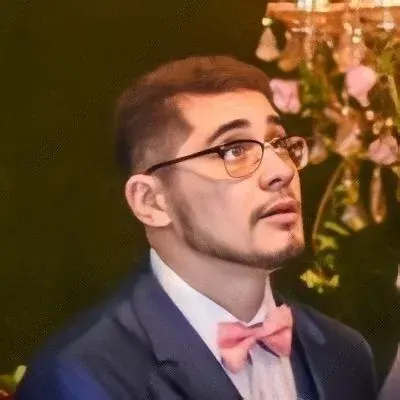
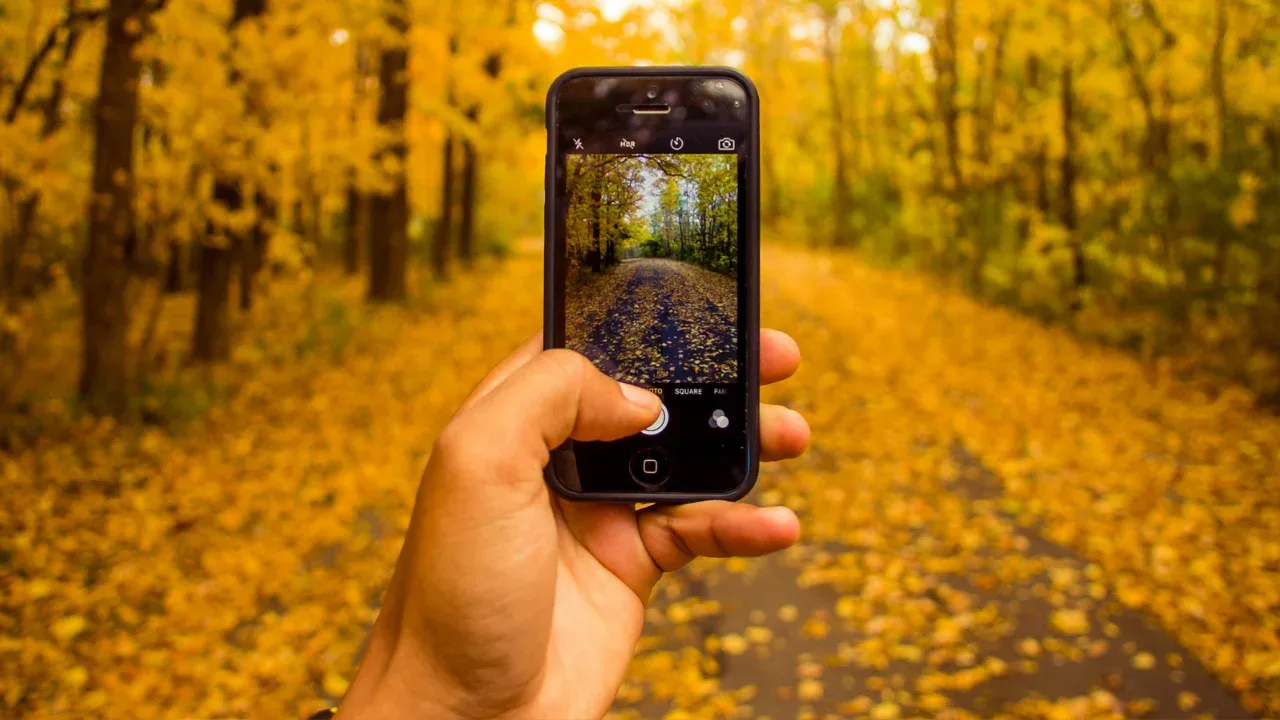
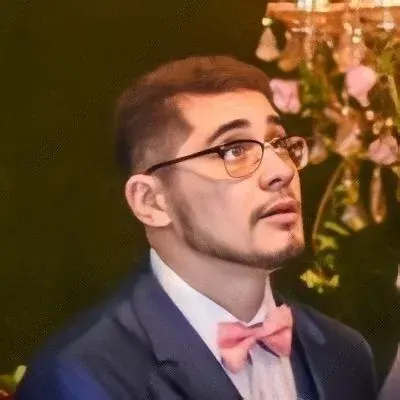
How to Delete an Item from a State Array in React: A Beginner's Guide
Have you ever encountered the daunting task of deleting an item from a state array in React? Fear not! In this guide, we will walk you through the common issues and provide you with easy solutions to remove a particular element from your state array. 🚀
The Problem
Let's dive into the problem at hand. You have a React component with a state array people
, and you want to remove a specific person from the array. In our example, we want to delete "Bob" from the people
array.
getInitialState: function() {
return {
people: []
}
},
// ...
removePeople(e) {
var array = this.state.people;
var index = array.indexOf(e.target.value); // Let's say it's Bob.
delete array[index];
}
The Solution 🎉
The issue here lies in the way we are trying to delete the element from the array. Instead of using the delete
operator, which only sets the element to undefined
, we should use the splice()
method. Here's how you can fix it:
removePeople(e) {
var array = this.state.people;
var index = array.indexOf(e.target.value); // Let's say it's Bob.
if (index > -1) {
array.splice(index, 1);
this.setState({ people: array });
}
}
In the modified solution, we use the splice()
method to remove the element at the index
position from the array. The splice()
method modifies the original array and returns a new array without the deleted element. We then update the state using this.setState()
to reflect the changes in the component.
Understanding the Solution
Let's break down the solution to gain a deeper understanding:
We retrieve the index of the element to be removed using the
indexOf()
method.If the element exists in the array (i.e.,
index > -1
), we remove it usingsplice(index, 1)
.Finally, we update the state with the modified array using
this.setState({ people: array })
.
Take it a Step Further
Now that you have mastered deleting an item from a state array, why not try implementing additional functionality? Here are a few ideas to get you started:
Add a confirmation prompt before deleting the item.
Enhance the UI by visually highlighting the item to be removed.
Implement undo functionality, allowing users to restore deleted items.
Conclusion
Deleting an item from a state array in React may seem challenging at first, but with the right approach, it becomes a breeze. By using the splice()
method and updating the state, you can easily remove any element from your array. Remember, always double-check your code for any syntax errors or logical mistakes.
If you found this guide helpful, don't forget to share it with your fellow developers and React enthusiasts. Happy coding! 💻👩💻👨💻
📢 Join the Discussion!
Have you come across any other challenges while working with React state arrays? Share your experiences or ask questions in the comments below. Let's learn from each other and grow as React developers!