How to delay the .keyup() handler until the user stops typing?
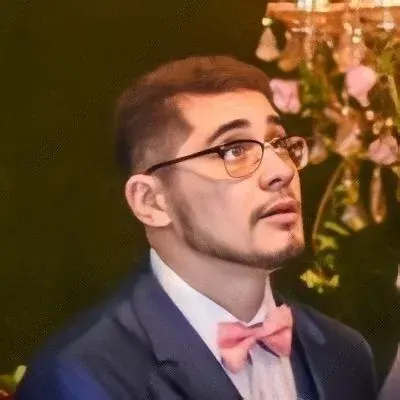
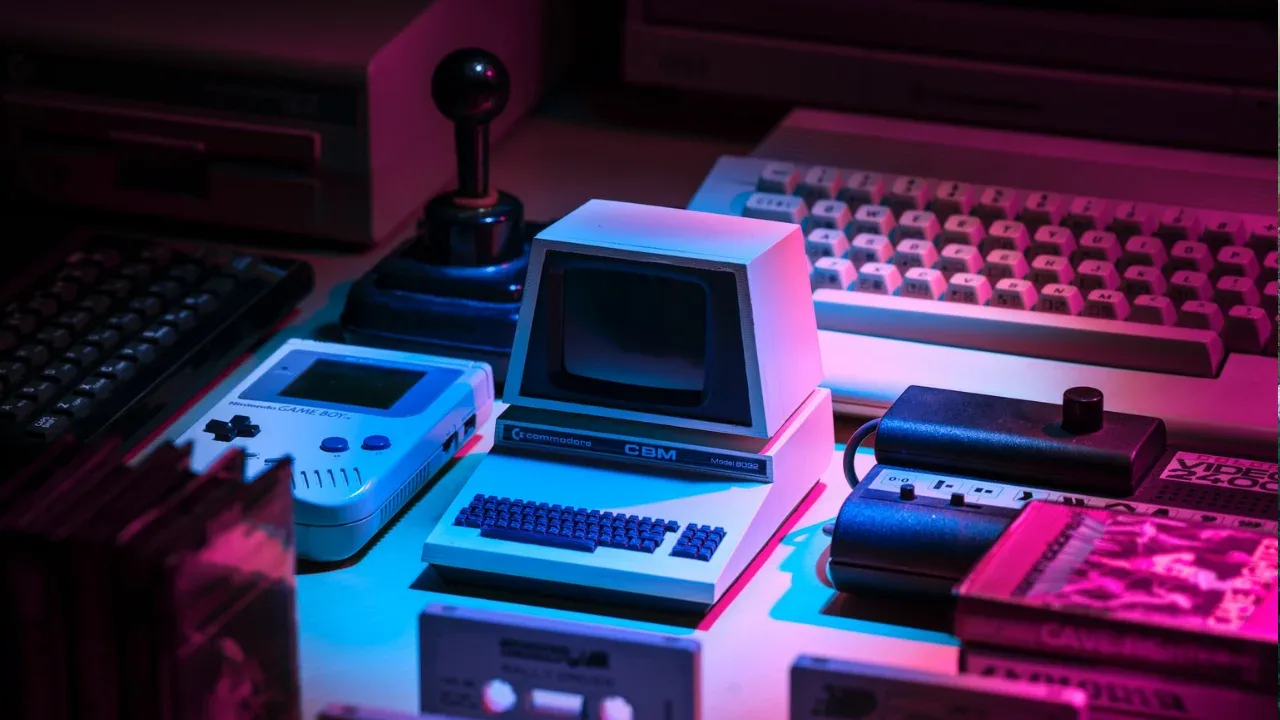
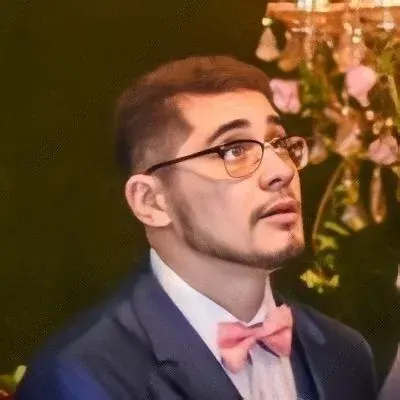
How to Delay the .keyup() Handler Until the User Stops Typing? 💡💬💭
Are you tired of your search field triggering multiple AJAX requests for every keystroke? 😫 Do you wish to optimize this process by implementing a delay so that it only searches once the user stops typing for a certain period of time? 🤔 Well, fret not! In this blog post, we'll dive into this common issue and provide you with easy solutions. 🚀
The Problem at Hand 🤷♀️🤔
Let's start by understanding the problem in detail. Imagine you have a search field, and every time a user types a character, it triggers the keyup
event. This means that if the user types "Windows," the search function will be invoked for each individual keystroke: "W," "Wi," "Win," "Wind," "Windo," "Window," and finally "Windows." This can potentially lead to redundant AJAX requests and unnecessary strain on your server. 😱
The Desired Solution 💡
To optimize this process, we want to introduce a delay so that the search function is only called once the user stops typing for a specified duration, let's say 200 milliseconds. This way, we can reduce the number of AJAX requests and only make the necessary calls when the user has finished typing. 🕑
Attempting the Obvious Solution ⌛❌
At first glance, it might seem logical to try using the setTimeout
function to accomplish this delay. However, as our context suggests, this approach didn't yield the desired results for our inquirer. 😕
Easy Solutions With Debouncing 🎯🛠
Fear not! We have just the right technique to solve this problem: debouncing. Debouncing allows us to delay the invocation of a function until the user stops performing a particular action, such as typing. 🛠️💤
Using JavaScript Libraries ✨
If you're using a JavaScript library, such as Lodash or Underscore, you're in luck! These libraries provide a debounce
function that simplifies the implementation process. Here's an example showcasing the usage of the Lodash debounce
function:
const debounceSearch = _.debounce(searchFunction, 200);
$('input').keyup(debounceSearch);
In this example, debounceSearch
is a debounced version of the searchFunction
(your AJAX call). The second parameter in the _.debounce
function determines the delay (200 milliseconds in this case). Now, the searchFunction
will only be called once the user stops typing for 200 milliseconds. 🙌
DIY Approach 🛠✋
If you prefer a do-it-yourself approach without external libraries, worry not, for it's quite doable! Here's a simple implementation using vanilla JavaScript:
let timeoutId;
function debounceSearch() {
clearTimeout(timeoutId);
timeoutId = setTimeout(searchFunction, 200);
}
document.querySelector('input').addEventListener('keyup', debounceSearch);
In this DIY approach, the debounceSearch
function is responsible for clearing the previous timeout (if any) and setting a new one. The searchFunction
will be invoked once the user stops typing for the specified duration (200 milliseconds).
Take It a Step Further! 🚀🌟
Now that you've learned how to delay the keyup
handler, why not take it a step further and enhance your search field with additional features? Here are a couple of ideas to get you started:
Implement a visual indication (e.g., a loading spinner) to let the user know that the search is in progress.
Add server-side pagination to display search results in chunks as the user types, providing a more seamless experience.
Join the Conversation! 💬👥
We hope this blog post helped you address the issue of delaying the .keyup()
handler until the user stops typing. Now, it's your turn to put these techniques into action! Have you encountered any other interesting solutions to this problem? What additional features have you added to your search functionality? Let us know in the comments below! 👇
Happy coding! 💻✨