How to create an array containing 1...N
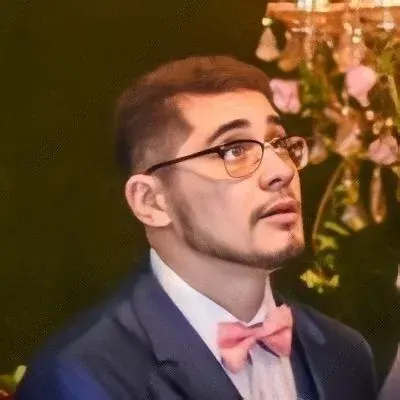
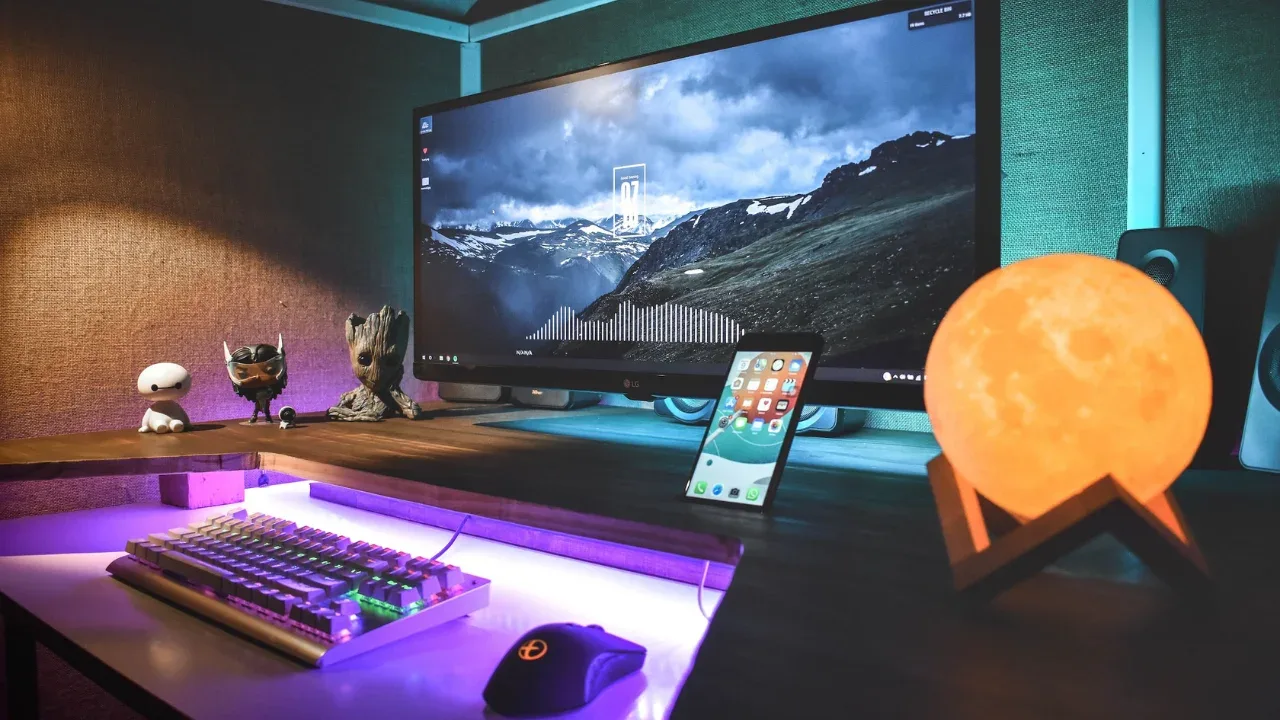
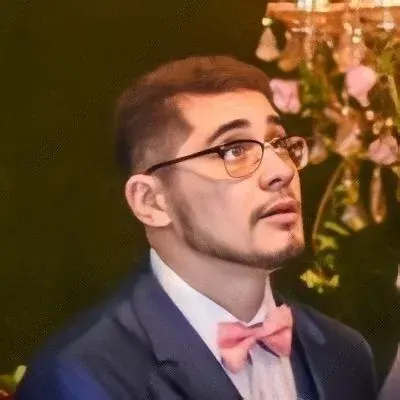
How to Create an Array Containing 1...N in JavaScript π€π‘
Are you tired of using a loop to create an array containing numbers from 1 to N in JavaScript? Do you want a simpler, more efficient way to achieve the same result? Well, you're in luck! In this blog post, we'll explore alternative approaches to create such an array without the need for a loop. Let's dive in! π
The Traditional Approach π
Before we explore alternative solutions, let's quickly revisit the traditional approach. The code snippet provided uses a loop to iterate from 1 to N and push each number into the foo
array. While this method is functional, it might feel a bit clunky and inelegant. Here's the code again for reference:
var foo = [];
for (var i = 1; i <= N; i++) {
foo.push(i);
}
The Splice Method π£
One alternative method to create an array containing numbers from 1 to N is to use JavaScript's splice()
method. This method allows us to remove or replace elements in an array, but it can also be leveraged to insert new elements. By combining splice()
with the spread operator ...
, we can achieve our desired result without using a loop. Take a look at this code snippet:
var foo = [...Array(N).keys()].splice(1);
In this approach, we first create an array of length N using Array(N)
, with each element being undefined
. We then use Array.keys()
to get an array-like object containing the indices of the array. By spreading this object within the []
brackets, we convert it into a regular array. Finally, we use splice(1)
to remove the first element (which is 0
) and get an array containing numbers from 1 to N.
The Map Function πΊοΈ
Another elegant solution involves using JavaScript's map()
function. This function applies a provided callback function to each element of an array and returns a new array with the results. By using the map()
function in conjunction with the Array.from()
method, we can easily create an array containing numbers from 1 to N. Here's how you can do it:
var foo = Array.from({ length: N }, (_, i) => i + 1);
In this code snippet, we use Array.from()
to create an array with a length of N, leaving each element as undefined
. The callback function (element, index) => index + 1
is then applied to each element during iteration, resulting in an array containing the desired numbers.
The Spread Operator π
If you prefer a more concise approach, you can combine the Array.from()
method with the spread operator ...
to create the desired array. Here's the code:
var foo = [...Array(N)].map((_, i) => i + 1);
In this snippet, the spread operator [...Array(N)]
converts the array-like object returned by Array(N)
into a regular array. We then use map()
with the same callback function as before to create an array containing numbers from 1 to N.
Conclusion and Challenge! π
Congratulations on learning alternative ways to create an array containing numbers from 1 to N in JavaScript! You are now equipped with multiple techniques that can make your code more readable and concise. π
Challenge: Think of a scenario where you can apply one of these alternative methods. Share your thoughts in the comments below! Let's start a discussion and see which approach resonates best with our readers. π¬
Remember, the beauty of coding lies in finding innovative solutions to everyday problems. Explore, experiment, and keep pushing the boundaries of what's possible! Happy coding! π»π