How to count string occurrence in string?
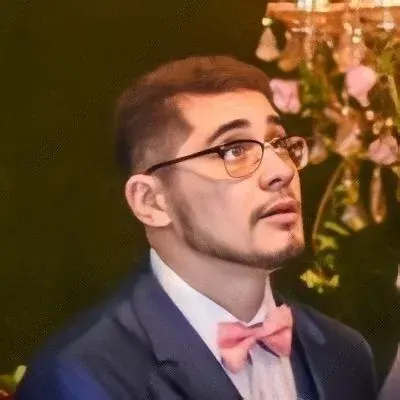
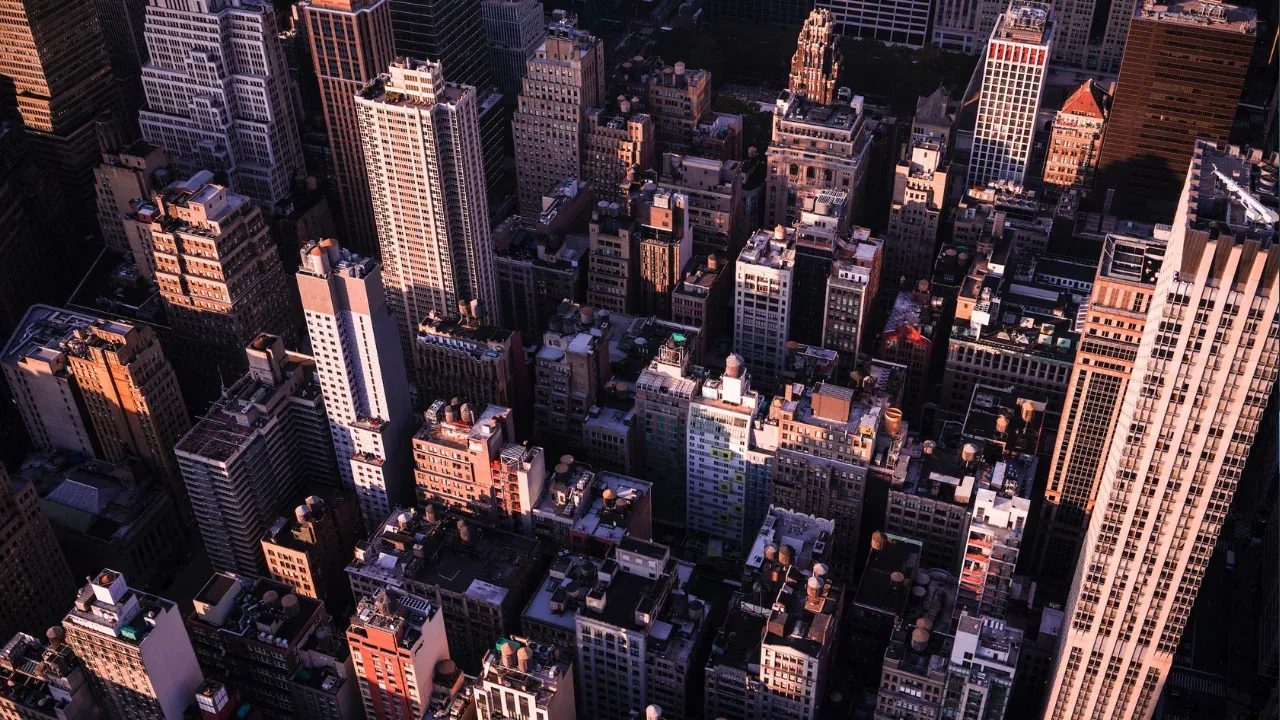
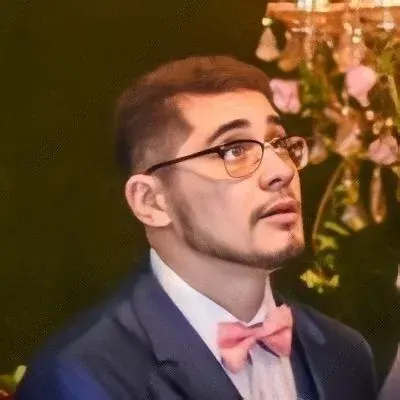
Easy Ways to Count String Occurrences in JavaScript
Are you tired of manually counting the number of times a specific string appears in a larger string? 🤔 Don't worry! We've got you covered. In this blog post, we'll explore some easy solutions to count string occurrences in JavaScript. Whether you're a beginner or an experienced developer, you'll find these methods useful. Let's dive right in! 💪
The Problem at Hand 😓
Imagine you have a string, and you want to count how many times a specific substring occurs within it. For instance, let's take an example:
var temp = "This is a string.";
You want to count how many times the substring "is" appears in the above string. In this case, the expected output should be 2
.
Solution 1: Using Regular Expressions (Regex) 🎯
One way to solve this problem is by harnessing the power of regular expressions. Regular expressions provide a flexible and efficient way to search for patterns in strings. In JavaScript, you can use the match()
function along with a regular expression to count string occurrences.
Here's an example of how you can do it:
var temp = "This is a string.";
var count = (temp.match(/is/g) || []).length;
console.log(count); // Output: 2
In the code above, we use the match()
function with the regular expression /is/g
to find all occurrences of the substring "is" in the temp
string. The || []
part ensures that even if no matches are found, the length will be 0
.
Solution 2: Using the split()
Method 🪓
Another approach to count string occurrences is by splitting the original string into an array of substrings and counting the length of that array. This method can be handy when dealing with simpler cases.
Here's how you can do it:
var temp = "This is a string.";
var count = temp.split("is").length - 1;
console.log(count); // Output: 2
In this solution, we split the temp
string at each occurrence of the substring "is" using the split()
method. The resulting array will have one more element than the number of occurrences. By subtracting 1
, we get the count of occurrences.
Conclusion and Call-to-Action 📚
Counting string occurrences in JavaScript doesn't have to be complicated. By using either regular expressions or splitting, you can effortlessly find the count you need. So, next time you encounter a similar problem, remember these easy solutions! 💡
We hope you found this guide helpful. If you have any questions or suggestions, feel free to leave a comment below. Also, don't forget to share this post with your fellow developers who might find it useful. Happy coding! 😄🚀