How to convert Set to Array?
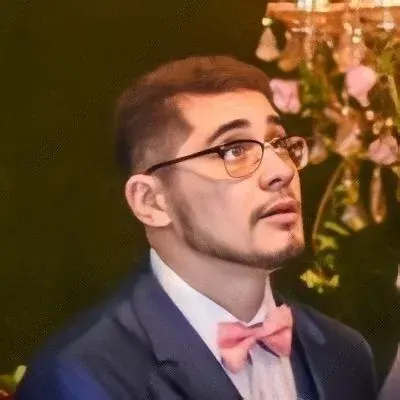
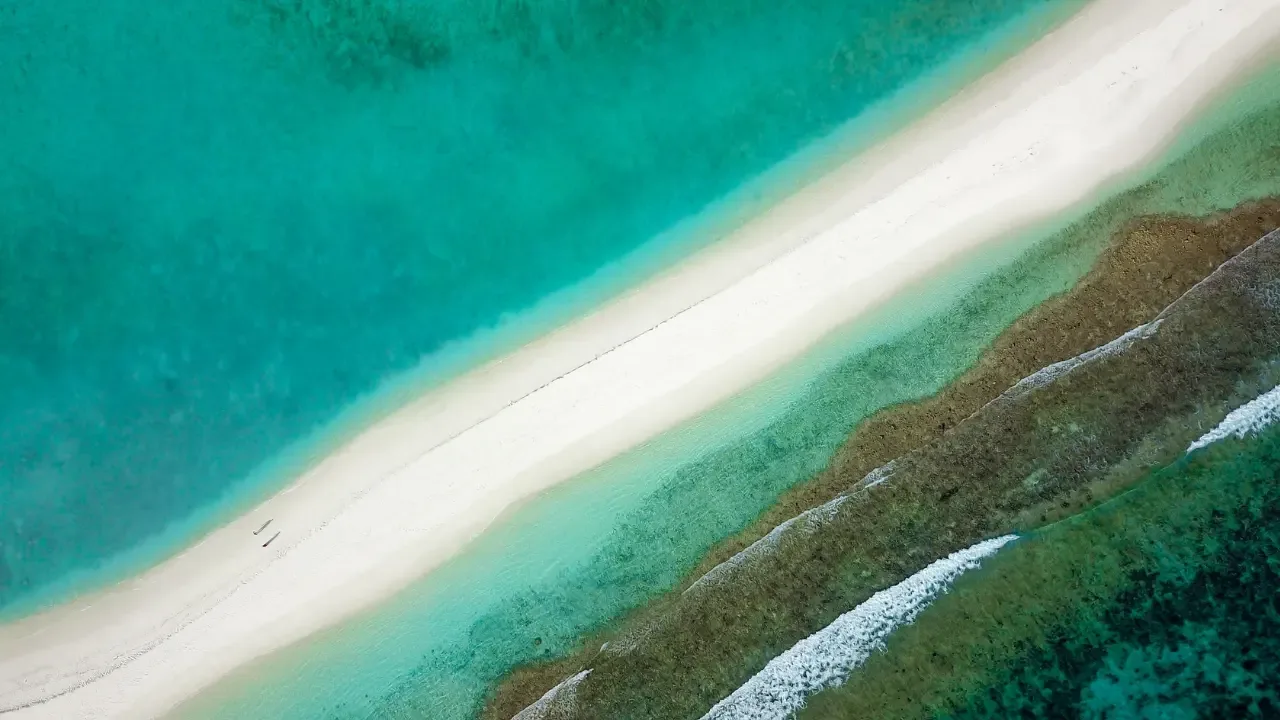
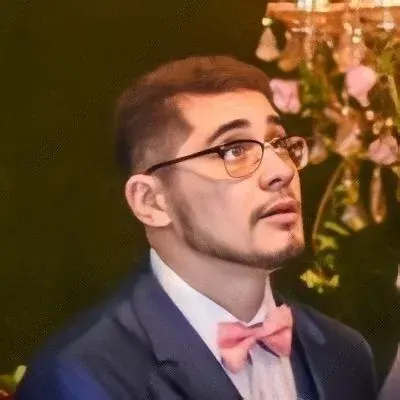
🔄 Converting Set to Array: A Handy Guide
Have you ever found yourself in a situation where you have a Set and you need to convert it into an Array? You're not alone! Many developers face this challenge when working with Sets. But fret not, because in this blog post, we'll explore easy solutions to convert a Set to an Array. Let's dive right in!
The Dilemma with Sets 😕
A Set is a great data structure when you need to store unique elements. However, it doesn't provide a straightforward way to access its values like Arrays do. The Set.prototype.values
method seems promising at first glance, but it requires a cumbersome next()
call. So, no luck there!
Additionally, you might have tried some popular conversion methods like Array.from
or Object.keys
. However, these methods aren't designed to handle Sets. 😔
The Quest for the Solution 🔍
Now, let's find a convenient inbuilt method or an idiomatic one-liner for converting a Set to an Array. We'll explore different approaches to accomplish this task.
Approach 1: Spread Operator 🌟
The spread operator is a powerful tool in JavaScript. Luckily, it can be used to convert a Set to an Array in just one line! 🎉
const mySet = new Set([1, 2, 3]);
const myArray = [...mySet];
console.log(myArray);
In this approach, we use the spread operator ...
to "spread" the values of the Set into a new Array. Voila! You've successfully converted your Set into an Array.
Approach 2: Array.from 🌈
Although we mentioned earlier that Array.from
alone does not work for Sets, it can come to the rescue when combined with the spread operator.
const mySet = new Set([1, 2, 3]);
const myArray = Array.from(mySet);
console.log(myArray);
By applying Array.from
on the Set, we can create a new Array with all the Set's values. Pretty neat, right? 🎩
Approach 3: Iteration using for...of 🌀
If you prefer a more traditional looping approach, the for...of
loop is your go-to solution.
const mySet = new Set([1, 2, 3]);
const myArray = [];
for (const value of mySet) {
myArray.push(value);
}
console.log(myArray);
Here, we iterate over the Set using for...of
, and for each value, we push it into a new Array. This method gives you more control in case you need to perform additional operations during the iteration.
🌟 Bonus Tip: Arrow Functions and One-liners! 🏆
If you're a fan of concise and elegant code, arrow functions can be your best friends. Let's see how we can create a one-liner using arrow functions:
const mySet = new Set([1, 2, 3]);
const myArray = Array.from(mySet, (value) => value);
console.log(myArray);
By utilizing an arrow function (value) => value
, we achieve the same result in a compact and expressive manner.
Engage with Us! 🎉
Now that you know how to convert a Set to an Array, you can tackle this challenge with ease. We hope you found this guide helpful and informative. If you have any questions or alternative methods, we'd love to hear from you! Share your thoughts in the comments section below.
May your Sets convert flawlessly and your Arrays be soaring high! Happy coding! 🚀