How to convert an Object {} to an Array [] of key-value pairs in JavaScript
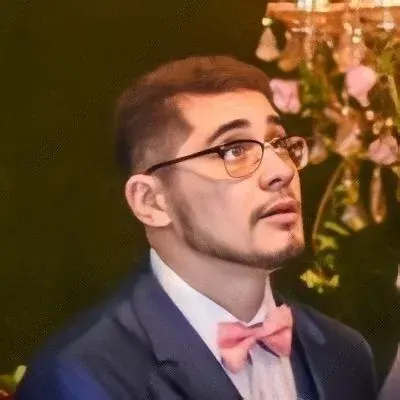
![Cover Image for How to convert an Object {} to an Array [] of key-value pairs in JavaScript](https://images.ctfassets.net/4jrcdh2kutbq/6XyqXj45QZ4M3eNKD0twkA/66b2d2f6d8c48cc92347d3e7bb893d5e/Untitled_design__14_.webp?w=3840&q=75)
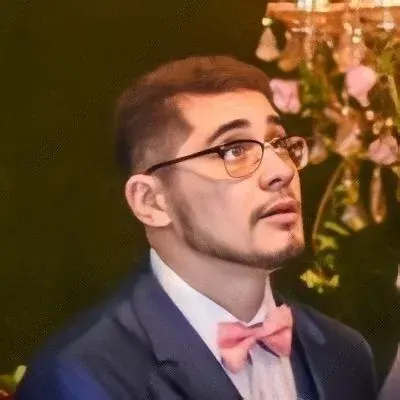
Converting an Object to an Array of Key-Value Pairs in JavaScript 💻
So, you have an object and you want to convert it into an array of key-value pairs in JavaScript? No worries! I've got you covered. 🤩
The Problem 🧐
Let's say you have an object like this:
const myObj = {"1": 5, "2": 7, "3": 0, "4": 0, "5": 0, "6": 0, "7": 0, "8": 0, "9": 0, "10": 0, "11": 0, "12": 0};
And you want to convert it into an array of key-value pairs like this:
[[1, 5], [2, 7], [3, 0], [4, 0], [5, 0], [6, 0], [7, 0], [8, 0], [9, 0], [10, 0], [11, 0], [12, 0]]
The Solution ✅
There are a couple of approaches you can take to solve this problem. Let's explore two simple and effective ways! 👇
1. Using the Object.entries() Method 🗝
JavaScript provides us with the handy Object.entries()
method which can be used to convert an object into an array of key-value pairs.
Here's how it works:
const myObj = {"1": 5, "2": 7, "3": 0, "4": 0, "5": 0, "6": 0, "7": 0, "8": 0, "9": 0, "10": 0, "11": 0, "12": 0};
const keyValueArray = Object.entries(myObj);
console.log(keyValueArray);
Running the code will output:
[
["1", 5],
["2", 7],
["3", 0],
["4", 0],
["5", 0],
["6", 0],
["7", 0],
["8", 0],
["9", 0],
["10", 0],
["11", 0],
["12", 0]
]
2. Using a for...in Loop 🔄
Another approach is to use a for...in
loop to iterate over the object's keys and push each key-value pair into a new array.
Here's the code:
const myObj = {"1": 5, "2": 7, "3": 0, "4": 0, "5": 0, "6": 0, "7": 0, "8": 0, "9": 0, "10": 0, "11": 0, "12": 0};
const keyValueArray = [];
for (let key in myObj) {
keyValueArray.push([key, myObj[key]]);
}
console.log(keyValueArray);
This will also output:
[
["1", 5],
["2", 7],
["3", 0],
["4", 0],
["5", 0],
["6", 0],
["7", 0],
["8", 0],
["9", 0],
["10", 0],
["11", 0],
["12", 0]
]
Time to Convert! ⏱️
Now that you know two easy ways to convert an object into an array of key-value pairs in JavaScript, you can confidently tackle this common problem in your code. 🚀
Would you like to learn more awesome JavaScript tips and tricks? Check out my blog and let's level up your coding skills together! 😎
Share this post with your fellow developers who might find it helpful. And don't forget to leave a comment below sharing your favorite method or any other creative solutions you've come up with. Let's keep the conversation going! 💬💪
Happy coding! ✌️