How to convert a string to an integer in JavaScript
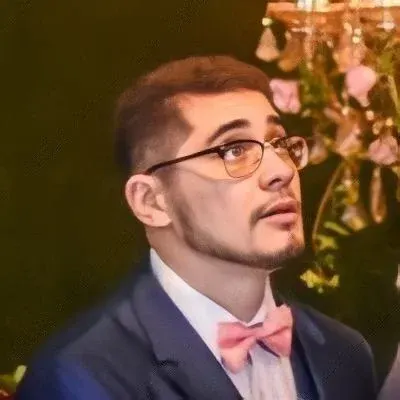
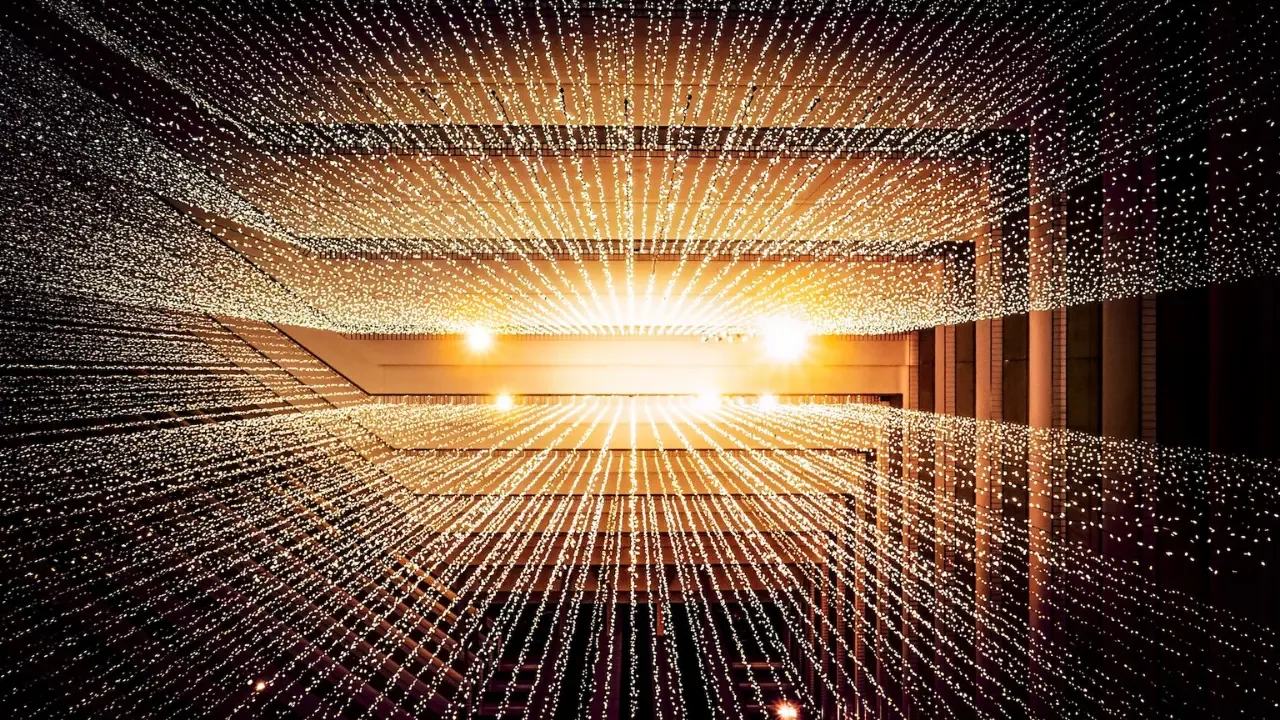
🔢How to Convert a String to an Integer in JavaScript🔢
So, you want to convert a string to an integer in JavaScript? Don't worry, you're not alone! Converting a string to an integer is a common task and can come in handy in various programming scenarios.
⚠️ Common Issues ⚠️
Before we dive into the solutions, let's address some common issues you might face when converting a string to an integer in JavaScript:
1️⃣ Issue #1: Numeric Characters Only
JavaScript's parseInt()
function only considers the numeric characters in the string. If the string contains non-numeric characters, it will stop parsing and return the value parsed so far. For example, parseInt("123abc")
will return 123
.
2️⃣ Issue #2: Leading Zeros Ignored
parseInt()
ignores leading zeros in a string. For example, parseInt("0123")
will return 123
. However, if the string starts with 0x
or 0b
, parseInt()
will interpret it as a hexadecimal or binary number, respectively.
3️⃣ Issue #3: Floating Point Numbers
If the string contains a floating-point number, parseInt()
will truncate it at the decimal point. For example, parseInt("3.14")
will return 3
.
💡 Easy Solutions 💡
Here are a few simple ways to convert a string to an integer in JavaScript:
1️⃣ Solution #1: parseInt()
The most basic way to convert a string to an integer is by using the built-in parseInt()
function. It takes the string as the first argument and an optional radix as the second argument (which specifies the base of the number). If the radix is not provided, it defaults to 10.
const str = "123";
const int = parseInt(str);
console.log(int); // Output: 123
2️⃣ Solution #2: Number()
Another approach is to use the Number()
function, which converts the entire string (including floating-point numbers) to a number.
const str = "456";
const int = Number(str);
console.log(int); // Output: 456
3️⃣ Solution #3: +
Operator
The simplest way to convert a string to an integer is by using the unary +
operator. It attempts to convert the string into a number and works well for strings that contain only numeric characters.
const str = "789";
const int = +str;
console.log(int); // Output: 789
📣 Get Involved! 📣
Now that you know how to convert a string to an integer in JavaScript, go ahead and try it out in your own code! Share your experiences and let us know which method worked best for you. If you have any questions or alternative solutions, drop a comment below. Happy coding! 😄👩💻
🔗 References:
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
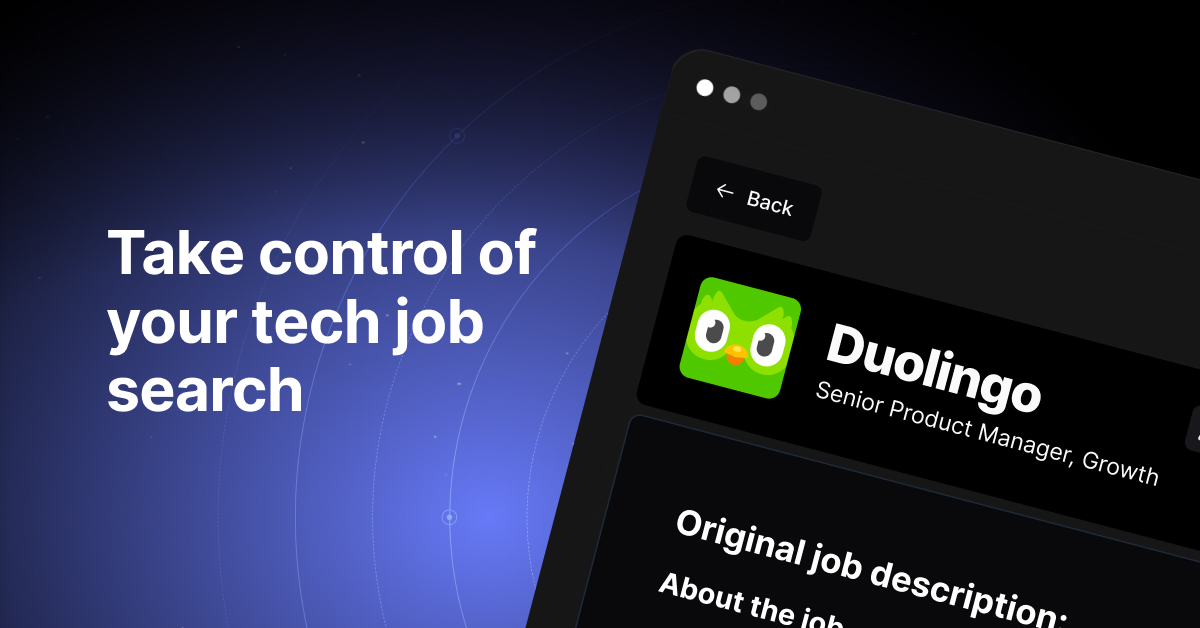