How to compare arrays in JavaScript?
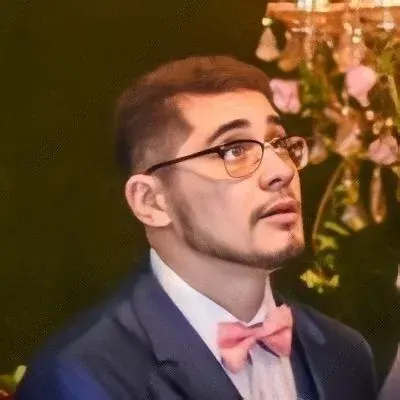
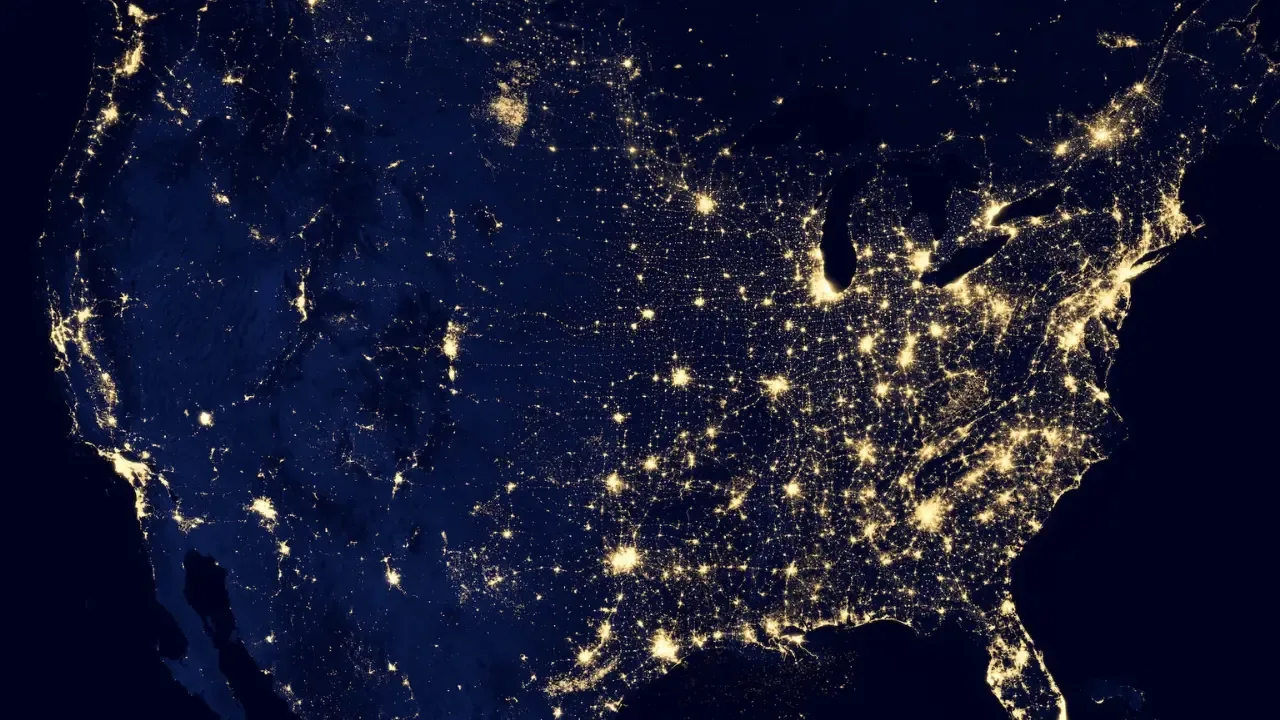
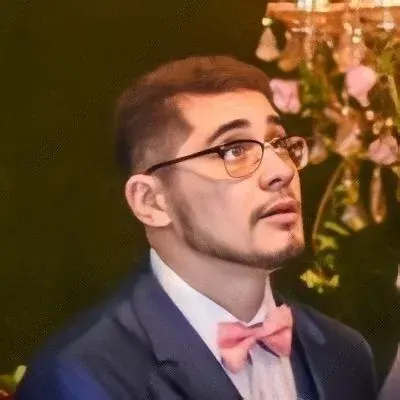
Comparing Arrays in JavaScript: Finding the Best and Fastest Method 😎
So, you want to compare arrays in JavaScript and get a simple true or false answer without the need to iterate through each value? I got you covered! 🛡️
The Challenge: Why Standard Comparison Doesn't Work ❌
Just using the ==
or ===
comparison operator won't give you the desired result when comparing arrays in JavaScript. If you try it, you'll get false as the output, just like the example below:
var a1 = [1, 2, 3];
var a2 = [1, 2, 3];
console.log(a1 == a2); // Returns false
Don't worry, you're not alone in facing this challenge! 🤝
JSON.stringify(): A Quick Solution 🐇
One way to compare arrays is by using the JSON.stringify()
function. This function converts the arrays into JSON strings, making it possible to perform a simple string comparison.
Here's how it works:
var a1 = [1, 2, 3];
var a2 = [1, 2, 3];
console.log(JSON.stringify(a1) == JSON.stringify(a2)); // Returns true
By converting the arrays to strings, we can now compare them successfully. This solution is simple and efficient, but is it the best way? Let's find out! 🧐
Finding a Better Solution: The Problem with JSON.stringify() 🐢
While JSON.stringify()
gets the job done, it might not be the most performant solution. This method involves converting the entire array into a string, which can be time-consuming and memory-intensive for larger arrays.
The Optimal Solution: A Custom Array Comparison Function ⚡
To make a fair comparison between arrays, we need to implement a custom comparison function that performs an element-wise comparison. This way, we can avoid converting arrays to strings and iterate directly through the values.
Let's see how we can write our own comparison function:
function compareArrays(arr1, arr2) {
if (arr1.length !== arr2.length) {
return false;
}
for (let i = 0; i < arr1.length; i++) {
if (arr1[i] !== arr2[i]) {
return false;
}
}
return true;
}
var a1 = [1, 2, 3];
var a2 = [1, 2, 3];
console.log(compareArrays(a1, a2)); // Returns true
In this custom function, we first check if the arrays have the same length. If they don't, we can instantly conclude that they are not identical.
Then, we loop through each element of the arrays and compare them one by one. As soon as we find a pair of elements that don't match, we can return false and stop the comparison early.
If the loop completes without finding any differences, we can confidently conclude that the arrays are identical.
This approach is not only faster than JSON.stringify()
, but it also allows us to stop the comparison as soon as a difference is found, saving unnecessary iterations. 🚀
Join the Discussion: Tell Us Your Preferred Method! 💬
Now that you know multiple ways to compare arrays in JavaScript, which one do you prefer? Have you encountered any other challenges while comparing arrays? Let's share our knowledge and experiences in the comments below!