How to check if an array is empty or exists?
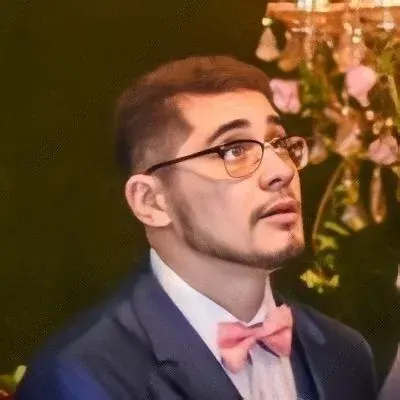
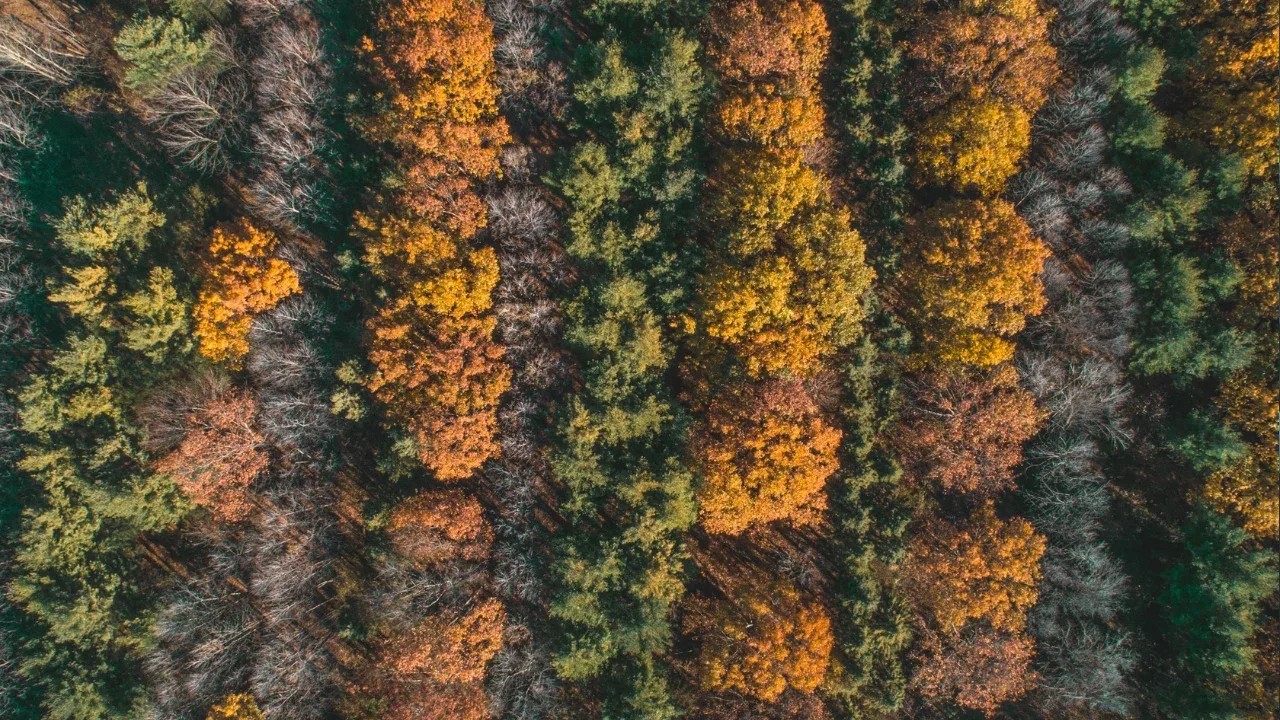
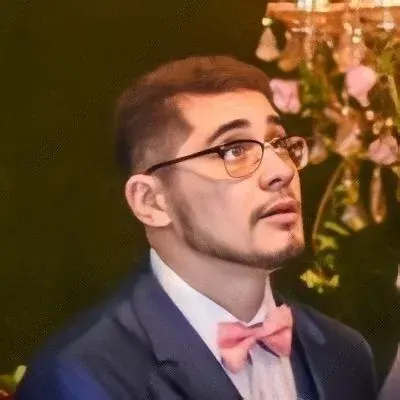
🔍 How to Check if an Array is Empty or Exists? 🤔
Hey there, tech enthusiasts! 👋 Are you having trouble checking if an array is empty or exists? 🤔 Don't fret, because we've got you covered! In this blog post, we'll address the common issues and provide you with easy solutions. Let's dive in! 💪
💥 The Problem: Overriding the Array All the Time 💥
So, you have a situation where you need to check if an image exists in the image_array
when your page is loading for the first time. If an image does exist, you want to load the last image. If not, you want to disable the preview buttons, alert the user to push a new image button, and create an empty array for future images.
Here's the code snippet you're working with:
if(image_array.length > 0)
$('#images').append('<img src="'+image_array[image_array.length-1]+'" class="images" id="1" />');
else{
$('#prev_image').attr('disabled', 'true');
$('#next_image').attr('disabled', 'true');
alert('Please get a new image');
var image_array = [];
}
But, uh-oh! The array in the else
statement seems to fire all the time. Even if an array exists, it just gets overridden, and the alert doesn't work as expected. 😱
💡 The Solution: Check the Array Existence Effectively 💡
To solve this issue and effectively check if an array is empty or exists, we need to make a few changes and optimize our code. Let's walk through the steps together:
Move the declaration of
image_array
outside theelse
statement. By declaring it before the conditional check, we ensure that it exists before any manipulation is done.
var image_array = [];
Now, let's modify our conditional check. Rather than checking
image_array.length > 0
, we'll useArray.isArray(image_array)
to check ifimage_array
is actually an array.
if (Array.isArray(image_array) && image_array.length > 0)
This will prevent any overriding issues and only execute the code if image_array
is an array with at least one element.
Additionally, it seems like we're trying to display the last image from the
image_array
. For that, we need to ensure that the array exists and contains elements. Let's update the code inside the conditional block as follows:
if (Array.isArray(image_array) && image_array.length > 0) {
$('#images').append('<img src="'+image_array[image_array.length-1]+'" class="images" id="1" />');
} else {
$('#prev_image').attr('disabled', true);
$('#next_image').attr('disabled', true);
alert('Please get a new image');
}
Finally, when you're loading HTML and setting
image_array
from PHP, make sure to check ifimages
exists and it's count is not 0. You can use thecount()
function in PHP to achieve this.
<?php if(count($images) != 0): ?>
<script type="text/javascript">
<?php echo "image_array = ".json_encode($images);?>
</script>
<?php endif; ?>
🎉 There you have it! Your array checking problem is solved and your code is working efficiently now. 🚀
📣 Take Action: Engage with Us! 📣
We hope this guide helped you understand how to check if an array is empty or exists. If you have any further questions or need clarification, feel free to leave a comment below. We're here to assist you! 👍
If you found this post helpful, don't hesitate to share it with your fellow developers. Let's spread the knowledge and help each other grow in the tech community! 🌐💖
Keep coding! 💻✨