How to check if a string "StartsWith" another string?
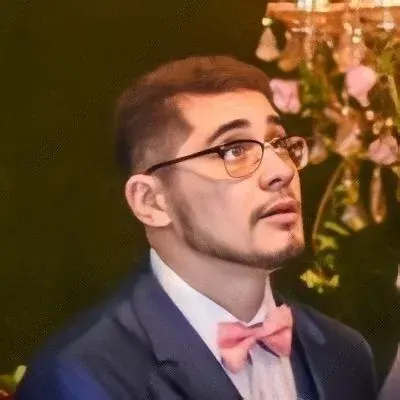
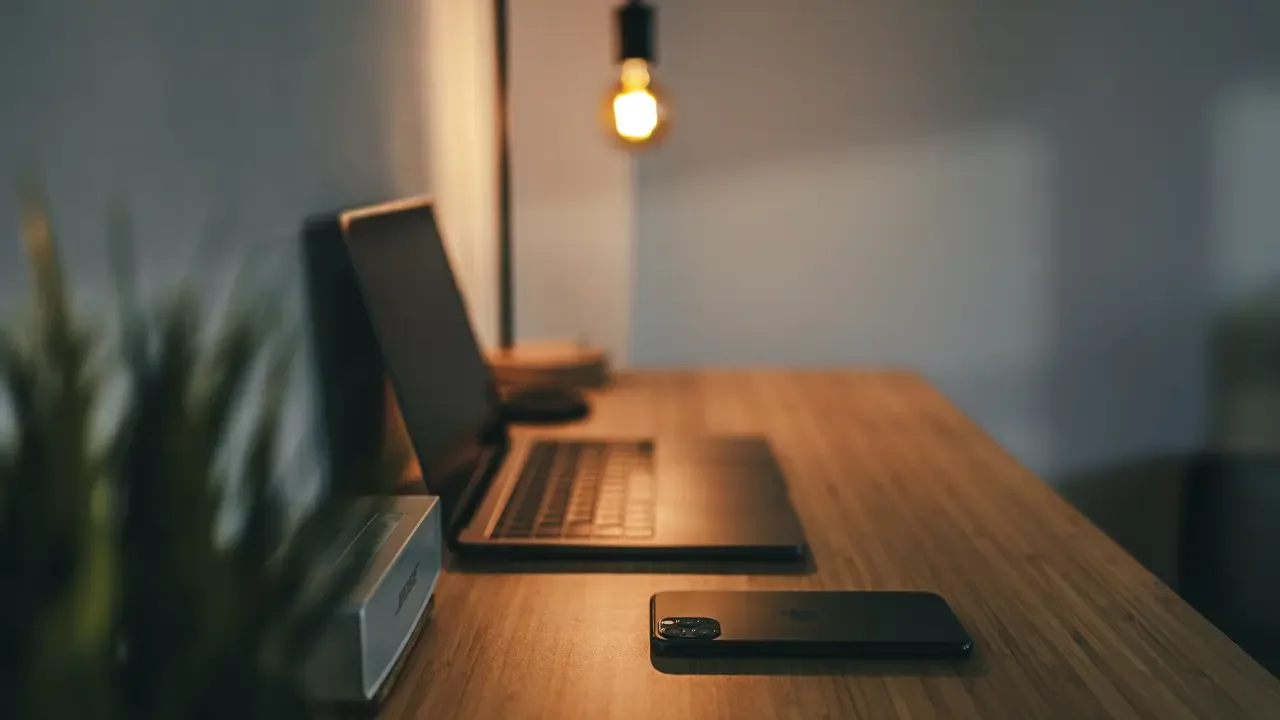
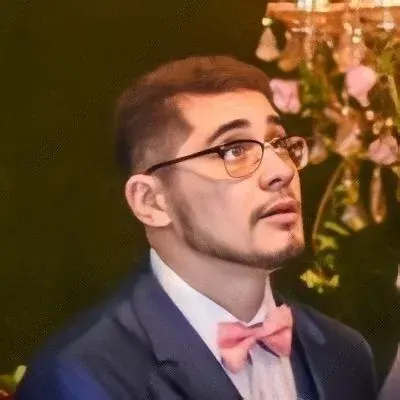
How to check if a string "StartsWith" another string? 🚀
Are you struggling to find a way to check if a string starts with another string? Look no further! In this blog post, we will tackle this common challenge and provide you with easy solutions. 💪
The Challenge 💡
Imagine you have a string called haystack
and you want to check if it starts with another string called needle
. This is similar to C#'s String.StartsWith
method. Let's take a look at an example:
var haystack = 'hello world';
var needle = 'he';
// Your goal is to find a way to check if haystack starts with needle
The Solution 💡
Solution 1: Using the "startsWith" method (ES6) ✨
If you are using ECMAScript 2015 (ES6) or a newer version of JavaScript, you can directly use the startsWith
method to check if a string starts with another string. Here's how you can do it:
var haystack = 'hello world';
var needle = 'he';
var startsWithNeedle = haystack.startsWith(needle);
console.log(startsWithNeedle); // Output: true
But hold on! Before you start using this method, there's an important thing to consider: browser support. As of now, the browser support for startsWith
method is not complete. So, if you need to support older browsers, you might need to look for an alternative solution.
Solution 2: Using a custom function 🛠️
If you need to support older browsers or prefer an alternative solution, you can create a custom function that checks if a string starts with another string. Here's a simple implementation:
function startsWith(haystack, needle) {
return haystack.slice(0, needle.length) === needle;
}
var haystack = 'hello world';
var needle = 'he';
var startsWithNeedle = startsWith(haystack, needle);
console.log(startsWithNeedle); // Output: true
In this solution, we use the slice
method to extract the first N characters from the haystack
string (where N is the length of the needle
string). Then, we compare it with the needle
string to determine if it starts with it.
Call-to-Action 📢
Now that you know how to check if a string starts with another string, it's time to put your knowledge into action! Try implementing it in your own code and see the results.
If you found this blog post helpful, don't forget to share it with your friends and colleagues. And if you have any questions or comments, please let us know in the comments section below. We would love to hear from you!
Happy coding! 💻🚀