How to check if a string is a valid JSON string?
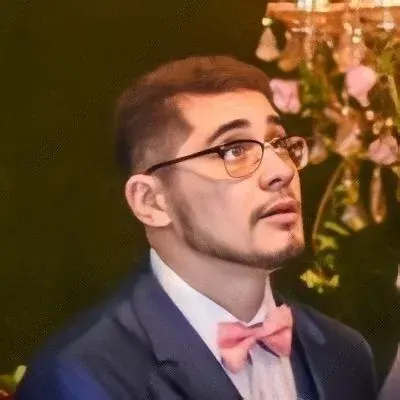
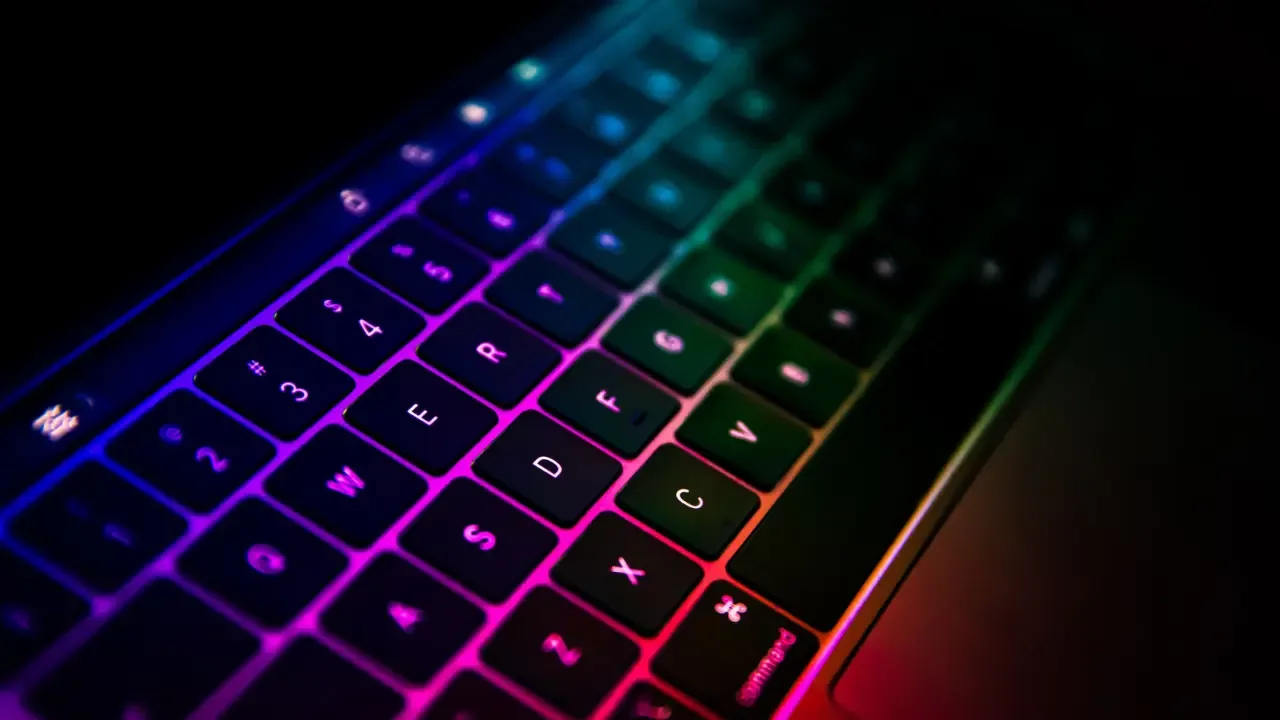
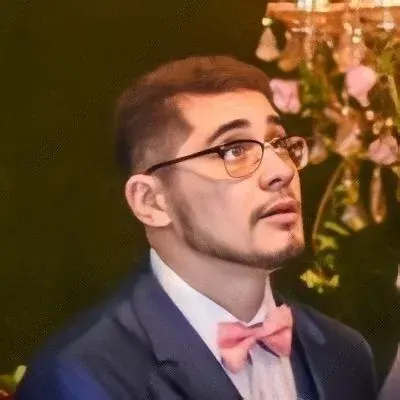
π§© How to Check if a String is a Valid JSON String?
Are you struggling to determine if a string is a valid JSON string? Fret not, because we've got you covered! In this blog post, we'll address common issues and provide you with easy solutions to tackle this problem. π
The Problem: Validating JSON Strings
As our context suggests, we need to check if a given string is a valid JSON string. Let's take a look at some examples to understand the problem better:
isJsonString('{ "Id": 1, "Name": "Coke" }') // should be true
isJsonString('foo') // should be false
isJsonString('<div>foo</div>') // should be false
The goal is to determine whether the given string adheres to the JSON syntax or not. However, there is a catch! We cannot use the try
/catch
approach, as it conflicts with our debugger settings, causing it to break on invalid JSON strings. So, we need a different solution. π
The Solution: Regular Expressions to the Rescue! π¦ΈββοΈ
Thankfully, regular expressions provide an elegant solution to this problem. By using a regular expression pattern, we can match valid JSON strings without relying on error handling. Let's dive into the code:
function isJsonString(str) {
try {
JSON.parse(str);
return true;
} catch (error) {
return false;
}
}
A New Approach: Parsing vs. Pattern Matching
To avoid using try
/catch
and satisfy our need for pattern matching, we can employ regular expressions. Here's the modified code:
function isJsonString(str) {
const jsonPattern = /^[\],:{}\s]*$/
.replace(/\\(?:["\\/bfnrt]|u[0-9a-fA-F]{4})/g, '@')
.replace(
/(?:[^"\\]|["\\/bfnrt]|u[0-9a-fA-F]{4})/g,
'\u2022'
);
return jsonPattern.test(str);
}
By utilizing regular expressions, we can validate whether the given string adheres to the JSON syntax or not. This approach is more efficient and avoids unnecessary try
/catch
statements.
Time to Put it to the Test! β
Let's put our solution through its paces with the same examples from before:
console.log(isJsonString('{ "Id": 1, "Name": "Coke" }')); // true
console.log(isJsonString('foo')); // false
console.log(isJsonString('<div>foo</div>')); // false
Success! Our function accurately determines whether a string is a valid JSON string or not, all without causing any unexpected debugger interruptions.
Join the Discussion! π¬
Now that you've learned a cool technique for checking JSON strings, we would love to hear your thoughts. Do you have any other creative solutions to this problem? Let us know in the comments section below! π
In Conclusion
Validating JSON strings without using try
/catch
can be a daunting task. However, with the power of regular expressions and a little bit of code, we were able to overcome this challenge. Remember to use the provided code and follow the examples to implement this solution in your own projects.
Thanks for reading! Stay tuned for more tech tips and tricks on our blog. π
Disclaimer: The examples and solutions provided in this blog post are for educational purposes only. It's always recommended to thoroughly test the code in your specific use case before applying it to production environments.