How to check a radio button with jQuery?
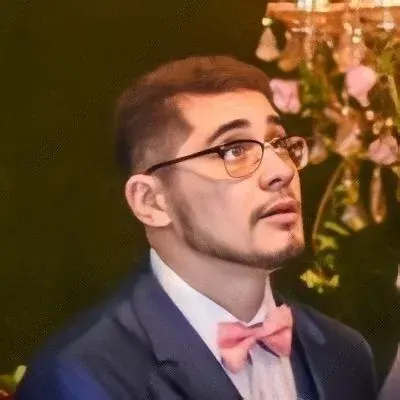
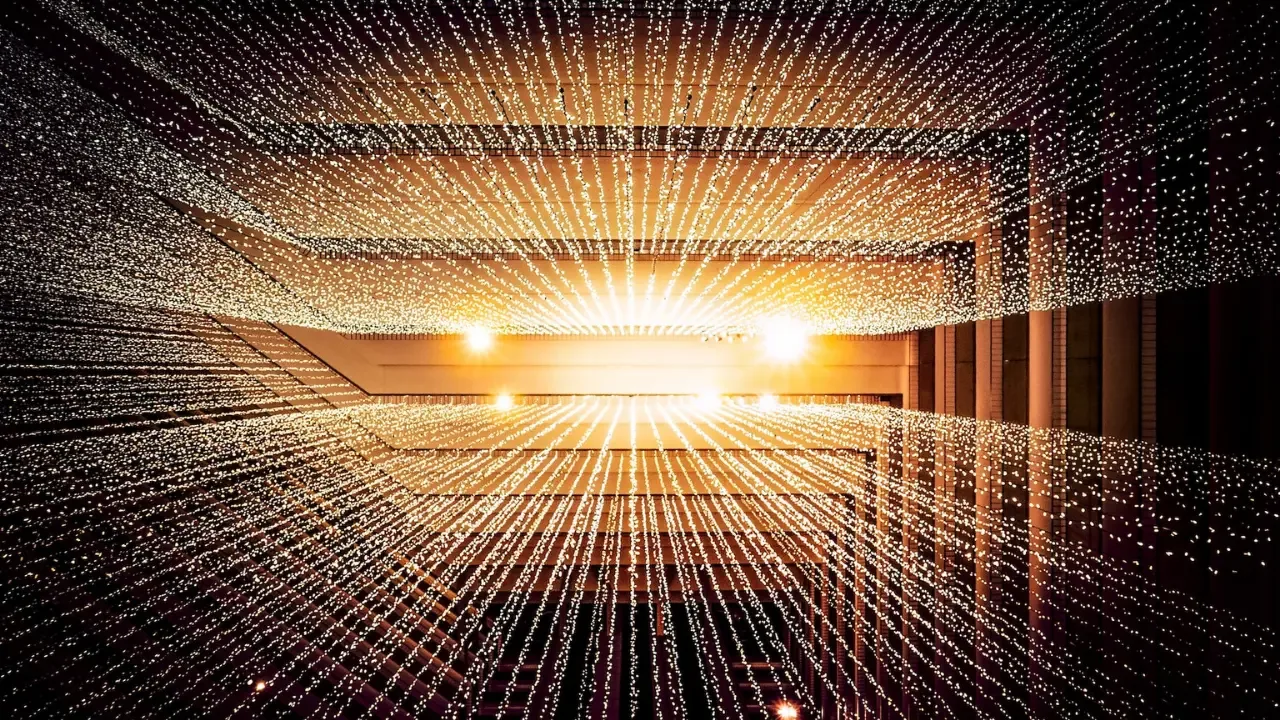
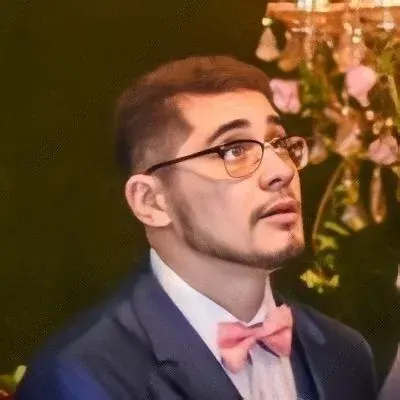
How to Check a Radio Button with jQuery: A Simple Guide 📻
Are you struggling with checking a radio button using jQuery? Don't worry, you're not alone! Many developers face this issue when trying to set radio button selections programmatically. In this article, we will explore common problems and provide easy solutions to help you check radio buttons effortlessly. So, let's tune in and get started! 🎶
The Setup 🔌
To begin, let's take a look at the code snippet you shared:
<form>
<div id='type'>
<input type='radio' id='radio_1' name='type' value='1' />
<input type='radio' id='radio_2' name='type' value='2' />
<input type='radio' id='radio_3' name='type' value='3' />
</div>
</form>
In this example, we have a form containing three radio buttons with unique IDs (radio_1, radio_2, radio_3), the same name attribute ('type'), and different values (1, 2, 3). The goal is to programmatically check one of these radio buttons using jQuery.
Attempt 1: jQuery("#radio_1").attr('checked', true); ❌
In your first attempt, you used the attr()
method to set the checked
property to true
for the radio button with ID #radio_1
. However, it didn't work as expected. 😕
Attempt 2: jQuery("input[value='1']").attr('checked', true); ❌
In an effort to find an alternative solution, you tried using a different selector: input[value='1']
. Unfortunately, this approach also failed to check the desired radio button. 😔
Attempt 3: jQuery('input:radio[name="type"]').filter('[value="1"]').attr('checked', true); ❌
Thinking logically, you applied a combination of selectors and filters: input:radio[name="type"]
and .filter('[value="1"]')
. However, this approach still didn't do the trick. 😩
The Straightforward Solution 💡
Instead of directly modifying the checked
attribute, you can leverage the prop()
method in jQuery to achieve the desired outcome. Here's how:
jQuery("#radio_1").prop('checked', true);
By using prop()
instead of attr()
, you can successfully check the radio button with the ID #radio_1
. 🥳
Key Takeaways 🌟
Changing the
checked
attribute withattr()
doesn't work consistently when checking a radio button.Utilize the
prop()
method in jQuery to set thechecked
property totrue
.With
prop()
and the correct selector (such as ID or class), you can effortlessly check a radio button programmatically.
🎉 Congratulations! You have successfully learned how to check a radio button using jQuery. Now, go ahead and try implementing it in your own project. If you have any additional questions or encounter any issues, don't hesitate to reach out.
Let us know if this guide helped you! Comment below and share your thoughts. And as always, keep exploring and keep coding! 🚀