How to break/exit from a each() function in JQuery?
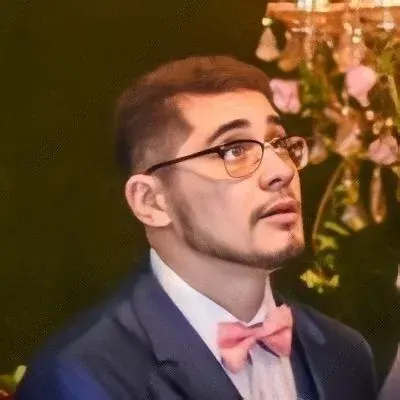
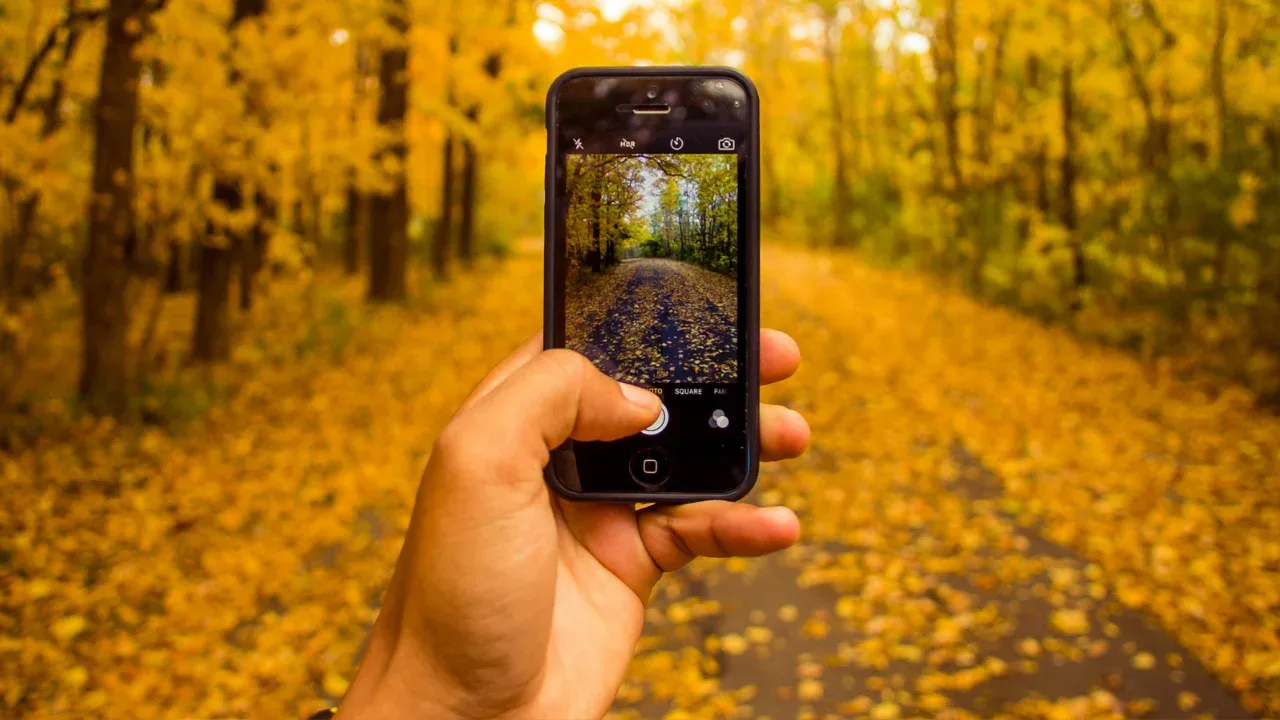
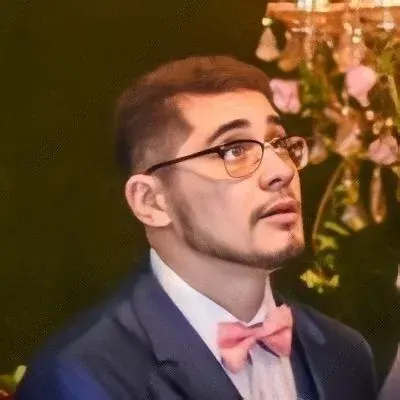
How to Break/Exit from a each()
Function in jQuery?
š Have you ever found yourself stuck in a loop and couldn't find a way to break out of it? You're not alone! In this guide, we'll explore how to break or exit from an each()
function in jQuery and provide easy solutions to common problems.
The Challenge: Escaping the each()
Block
Let's start with a common scenario. Imagine you have the following code:
$(xml).find("strengths").each(function() {
// Code
// How can I escape from this block based on a condition?
});
In this case, you're iterating over a collection of elements using the each()
function. However, there might be situations where you need to break out of the loop prematurely based on a certain condition.
Solution: Using a Return Statement
To exit the each()
block based on a condition, you can use a return statement with the value false
. Here's how you can implement it:
$(xml).find("strengths").each(function() {
if (condition) {
return false; // Breaks out of the each() loop
}
// Code to execute for each item
});
By returning false
, you tell jQuery to stop iterating and exit the each()
block. This is a simple and effective way to break out of the loop when needed.
Handling Nested each()
Loops
Now, let's take it up a notch! What if you have nested each()
loops and want to break out of both loops simultaneously? Consider the following example:
$(xml).find("strengths").each(function() {
$(this).each(function() {
// I want to break out from both each loops at the same time
});
});
In this case, you have an outer each()
loop and an inner each()
loop. To break out of both loops, you can use a named function and label it using the break
statement. Here's how it works:
$(xml).find("strengths").each(function() {
var outerLoop = this;
$(this).each(function() {
// Code
if (condition) {
break outerLoop; // Breaks out of both each loops
}
// Code to execute for each item
});
});
By using the break
statement with the name of the function, you can break out of both loops and resume execution outside of them.
Continue Instead of Breaking Out
But what if you want to skip to the next iteration instead of completely breaking out of the loop? Well, we've got you covered on that too! You can achieve that by using the return true;
statement inside the each()
block. Here's an example:
$(xml).find("strengths").each(function() {
// Code
if (condition) {
return true; // Continues to the next iteration
}
// Code to execute for each item
});
By returning true
, you tell jQuery to move on to the next iteration of the loop.
In Conclusion
Breaking out of an each()
loop in jQuery can be achieved by using a return statement with false
to exit from the loop prematurely. To break out of nested loops, you can use named functions and the break
statement. On the other hand, using return true
allows you to skip to the next iteration instead of breaking out of the loop entirely.
Now that you have a better understanding of these techniques, go ahead and try them out in your code! If you have any questions or other topics you'd like us to cover, feel free to reach out in the comments below.
š¤ Remember, sharing is caring! If you found this guide helpful, don't forget to share it with your fellow developers. Happy coding! š