How to auto-reload files in Node.js?
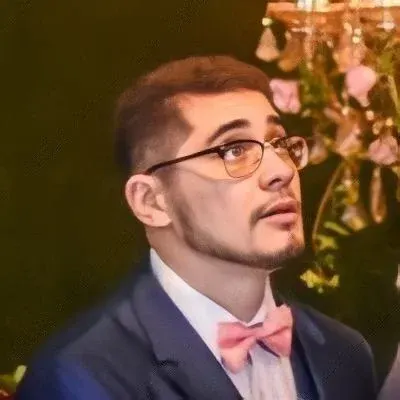
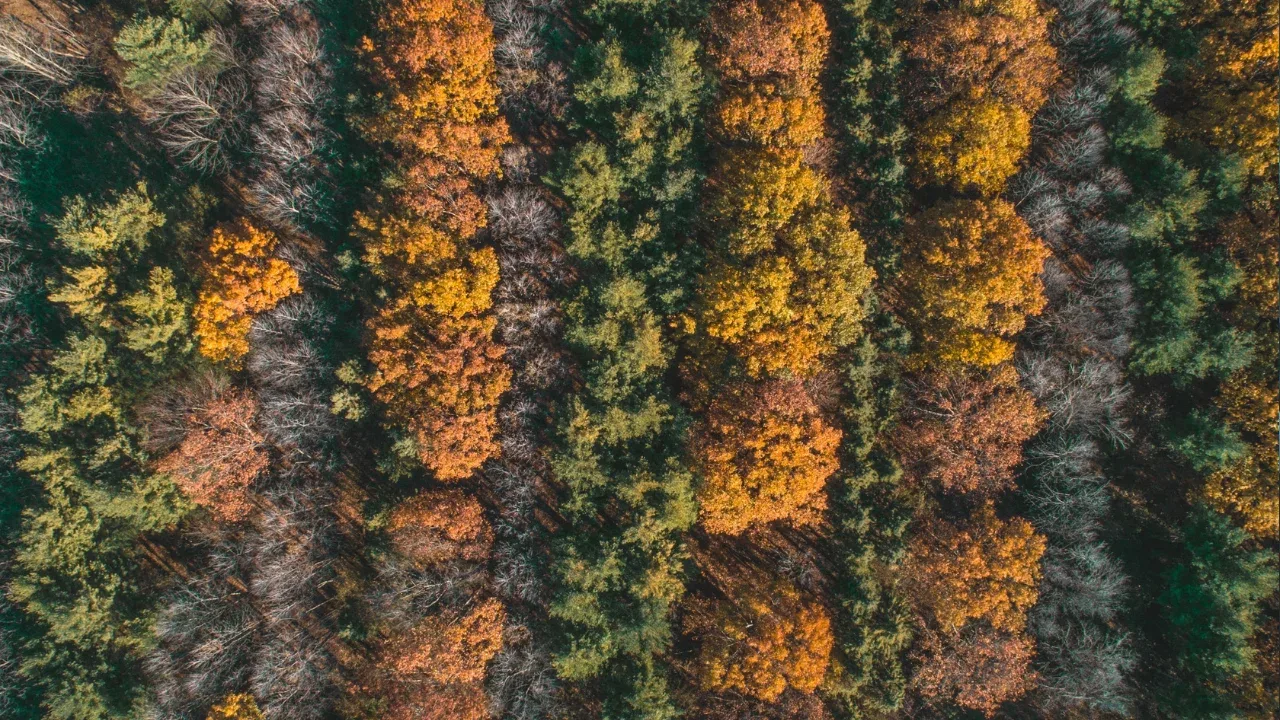
🎉 Auto-reload Files in Node.js: Never Restart Your Server Again! 🚀
Are you tired of manually restarting your Node.js server every time you make a file change? We feel your pain! 😩 But don't worry, we've got you covered with an easy solution to automate the process and save you time and frustration. Let's dive in and learn how to auto-reload files in Node.js!
The Challenge: require() and File Reloads
In Node.js, the require()
function plays a vital role in loading modules. However, it doesn't automatically reload files if they have already been required. This means that any changes you make to a file won't take effect until you manually restart your server. Want to avoid that hassle? We've got the answer!
The Solution: Using process.watchFile()
To achieve auto-reloading of files in Node.js, we can utilize the process.watchFile()
method. This handy function allows you to monitor a file for changes and take appropriate actions when it is modified.
Here's an example code snippet that demonstrates how to use process.watchFile()
to reload your files automatically:
const fs = require('fs');
const path = require('path');
const filePath = path.join(__dirname, 'path/to/file.js');
function reloadFile() {
delete require.cache[require.resolve(filePath)];
const reloadedFile = require(filePath);
console.log('File reloaded successfully!');
}
fs.watchFile(filePath, (curr, prev) => {
if (curr.mtime !== prev.mtime) {
reloadFile();
}
});
In this example, we first define the file path we want to monitor using fs
and path
modules. Then, we create a reloadFile
function that removes the module from the cache using delete require.cache
and requires it again. Finally, we use fs.watchFile()
to watch for changes in the file and trigger the reloadFile
function when a modification is detected.
Note: You can customize the filePath
variable to point to the specific file you want to auto-reload.
Dealing with Global Variables
One potential issue you may encounter, as mentioned in the provided code snippet, is that the process.compile()
statement throws an error stating that 'require' is not defined. This occurs because process.compile()
evaluates the file independently and isn't aware of the Node.js global variables.
To resolve this issue, you can switch from using process.compile()
to eval()
, which runs the code in the current scope and has access to all the Node.js globals. Here's an updated version of the previous code snippet using eval()
:
const fs = require('fs');
const path = require('path');
const filePath = path.join(__dirname, 'path/to/file.js');
function reloadFile() {
const content = fs.readFileSync(filePath, 'utf8');
eval(content);
console.log('File reloaded successfully!');
}
fs.watchFile(filePath, (curr, prev) => {
if (curr.mtime !== prev.mtime) {
reloadFile();
}
});
By using eval()
, we can eliminate the 'require is not defined' error and ensure smooth auto-reloading of files.
Your Turn: Try Auto-reloading Now! 💻
Now that you know how to auto-reload files in Node.js, it's time to leverage this knowledge and make your development process more efficient. Implement auto-reloading in your project and let us know how it works for you!
Have any questions or faced any challenges during the process? Comment down below and share your thoughts, and we'll be more than happy to assist you. Let's create a vibrant discussion around auto-reloading files in Node.js!
Happy coding! 🎉✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
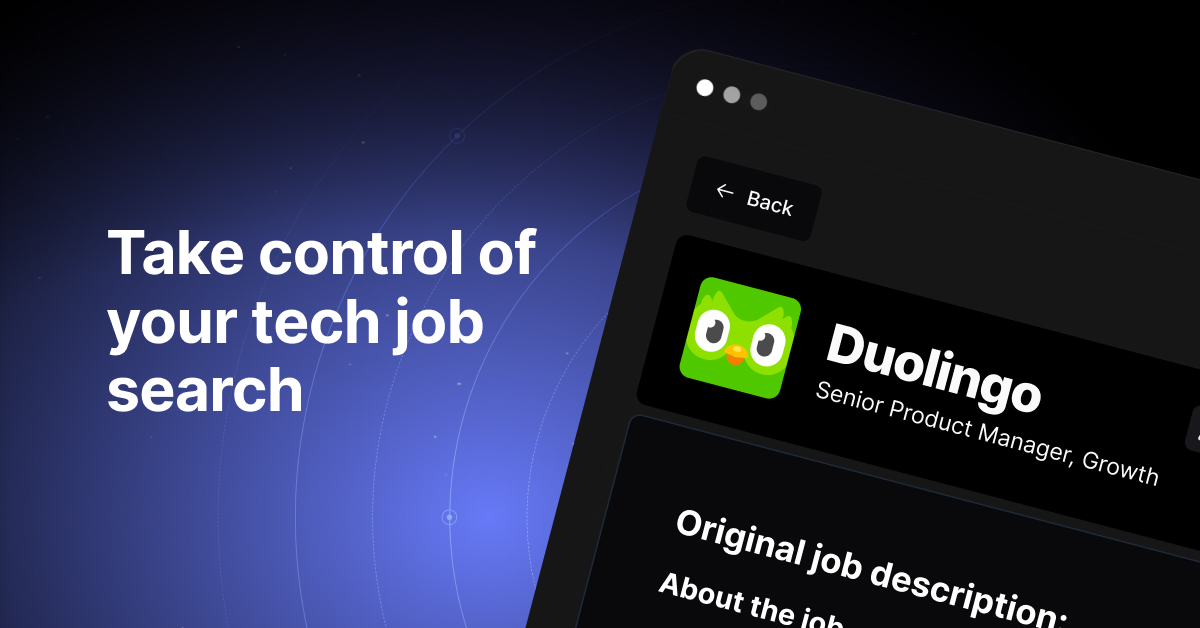