How to append to a file in Node?
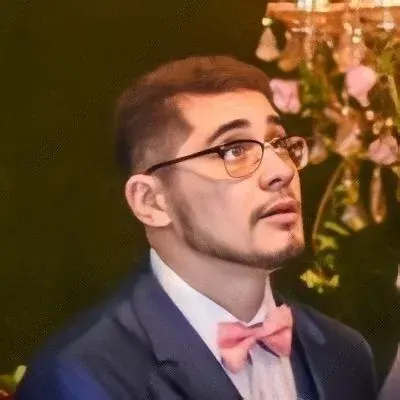
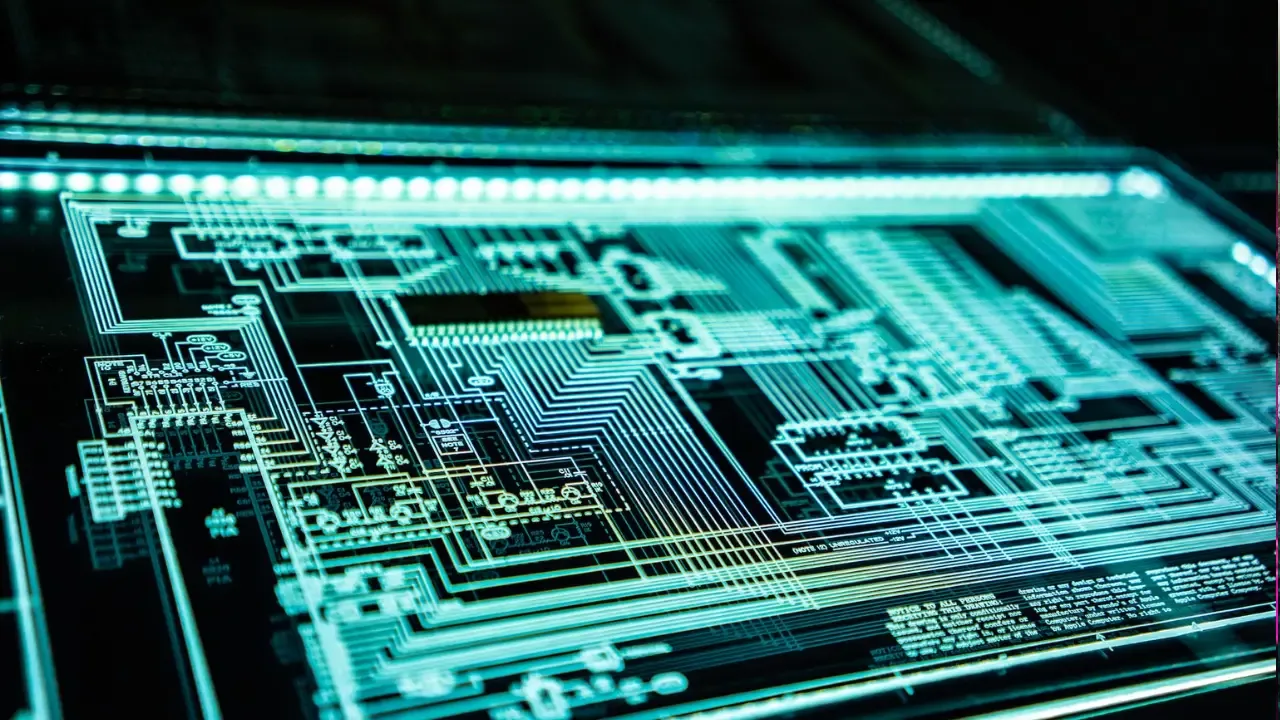
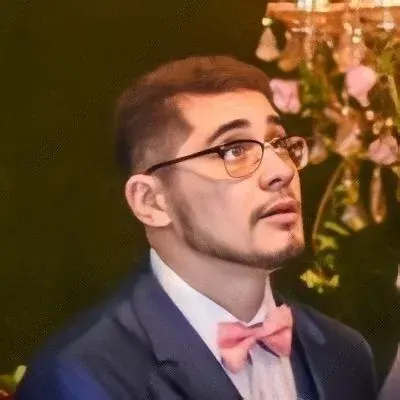
How to Append to a File in Node? 📝💻
Are you struggling with erasing the content each time you try to append a string to a log file in Node? 🤔
The usual suspect for this problem is using the writeFile
method. As the name suggests, the method overwrites the file each time it is called. 😱 But don't worry, we have an easy solution for you! 💡
Let's Start Appending! 🚀
To append a string to a file in Node, we need to use the fs.appendFile
method instead of fs.writeFile
. This method ensures that the content is added at the end of the file, rather than erasing the existing content. 📝😅
Let's take a look at how you can use fs.appendFile
:
const fs = require('fs');
fs.appendFile('log.txt', 'Hello Node', function (err) {
if (err) throw err;
console.log('String appended to file!');
});
And that's it! You have successfully appended the string to the file without erasing the existing content. 🎉🙌
Recap and Additional Tips 💡
Use
fs.appendFile
instead offs.writeFile
to avoid erasing the file content.Remember to have the necessary permissions to write to the file you want to append to.
If the file doesn't exist,
fs.appendFile
will create it for you.If you want to append multiple strings, you can call
fs.appendFile
multiple times.
Time to Get Appending! ⌨️
Now that you know the easy way to append to a file in Node, it's time to give it a try! Get creative with your log files and start appending strings without losing any previous content. 😄
If you have any questions or other topics you'd like us to cover, feel free to leave a comment below. We love hearing from our readers and are always here to help! 💌
Keep coding and happy appending! 👩💻👨💻