How to append something to an array?
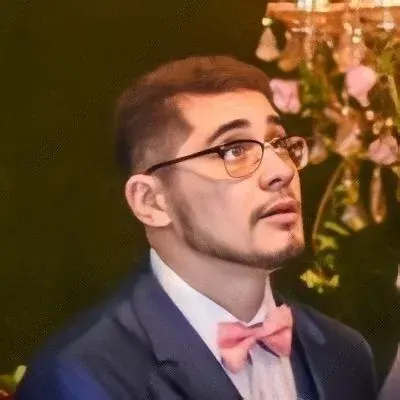
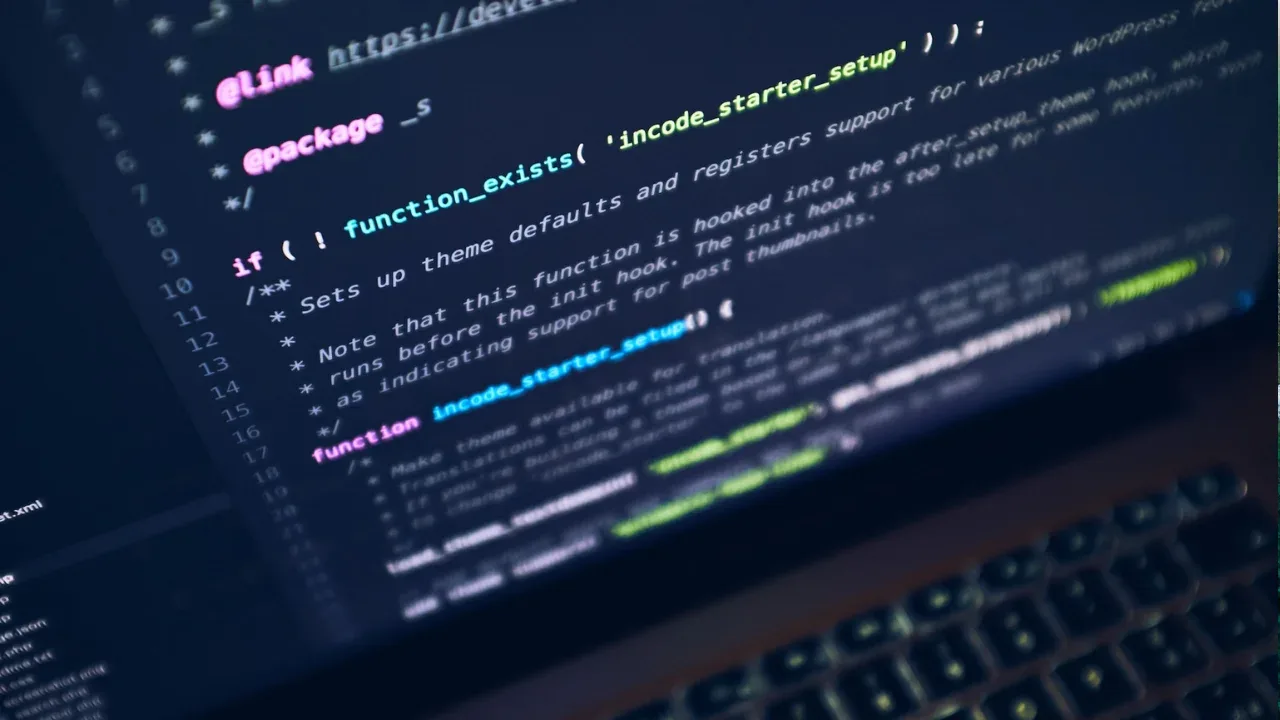
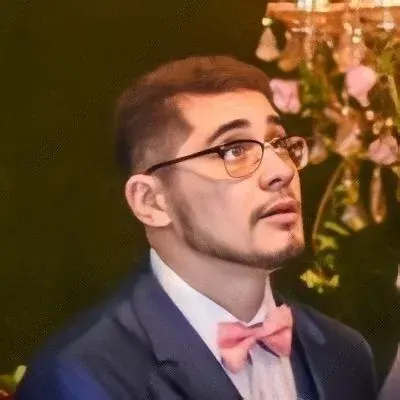
🚀 Easy Peasy Guide: How to Append Something to an Array in JavaScript
So you want to add some spice to your arrays? No worries, I've got you covered! In this super simple guide, we'll explore how to append an object, such as a string or number, to an array in JavaScript. 💪
The Problem: Appending something to an array
Sometimes, you just need to add a little extra to your array. Whether it's a new value or a shiny object, appending to an array can be handy. But how do we do it without pulling our hair out? 🤔
The Solution: Array.push()
Luckily, JavaScript provides us with an elegant solution - the Array.push()
method! This little gem allows us to effortlessly append any object to the end of our array. Let's see it in action:
const myArray = [1, 2, 3];
myArray.push(4); // Appending the number 4
myArray.push("hello"); // Appending the string "hello"
Voila! The push()
method adds the objects to the end of the array, growing it dynamically as needed. 🎉
But wait, there's more! The push()
method even returns the new length of the array, so we can keep track of how much we're growing:
const myArray = [1, 2, 3];
const newArrayLength = myArray.push(4); // Returns 4
console.log(newArrayLength); // Output: 4
Common Issues:
1. Forgetting to declare an array
If you don't declare an array before trying to append something to it, JavaScript won't know what you're talking about! Avoid this issue by declaring your array beforehand:
const myArray = []; // Declaring an empty array
myArray.push("hello"); // Appending to myArray
2. Attempting to push to something that's not an array
Pushing objects to a non-array variable is like trying to fit a square peg in a round hole. JavaScript won't let you get away with it! Check that your variable is an array before pushing to it:
const myArray = "I'm not an array!";
if (Array.isArray(myArray)) {
myArray.push("Oops!"); // This won't execute
} else {
console.log("myArray is not an array!"); // Output: myArray is not an array!
}
Your Turn: Let's Have Some Fun! 🎉
Now it's your turn to play with arrays and append your own objects! Whether you're adding numbers, strings, or even emojis, the push()
method is here to help you unleash your creativity! Share your favorite way of appending to arrays in the comments below! ✍️💬
Let's keep the conversation going on our community forum! We'd love to hear your thoughts and help you out with any coding conundrums.
That's all folks! Happy appending! 🚀