How to add multiple classes to a ReactJS Component?
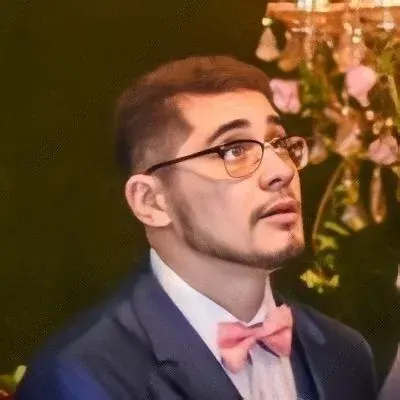
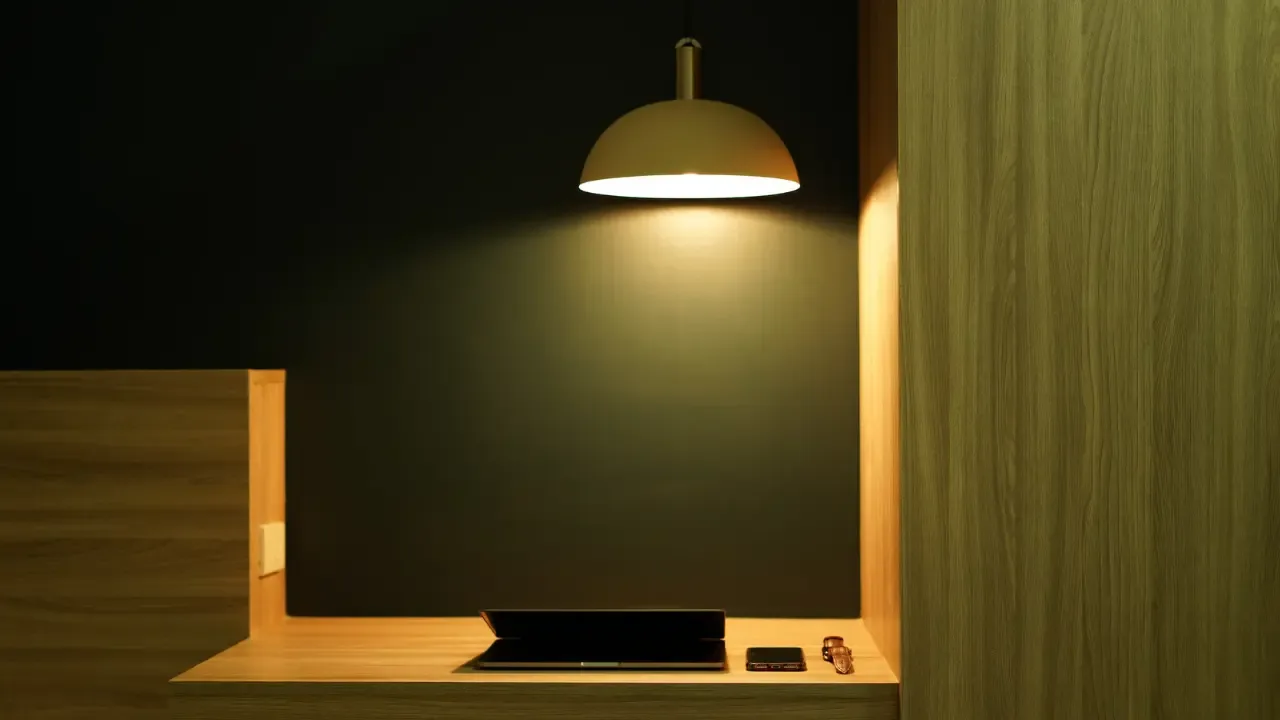
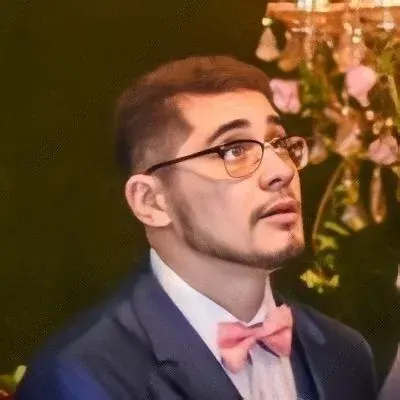
How to Add Multiple Classes to a ReactJS Component 🎉
Are you new to ReactJS and JSX and struggling to add multiple classes to a ReactJS component? Don't worry, we've got you covered! In this blog post, we will walk you through the process of adding multiple classes to a ReactJS component with easy-to-follow steps and examples.
The Problem 😕
Let's take a look at the code snippet you shared:
<li key={index} className={activeClass, data.class, "main-class"}></li>
You are trying to add multiple classes to the className
attribute on each <li>
. However, the way you are doing it is incorrect, and the classes are not being applied as expected.
The Solution 💡
To add multiple classes to a ReactJS component, you can make use of template literals or string concatenation. Here's how you can do it:
Using Template Literals
<li
key={index}
className={`${activeClass} ${data.class} main-class`}
></li>
In this solution, we are using template literals (enclosed within backticks ` `) to concatenate the classes together. The ${}
notation allows us to interpolate variables (activeClass
and data.class
) within the template.
Using String Concatenation
<li
key={index}
className={activeClass + " " + data.class + " main-class"}
></li>
In this solution, we are using the +
operator for string concatenation to combine the classes together. Make sure to separate each class with a space to ensure proper formatting.
Bringing it All Together 🚀
Let's integrate the solution into your existing code:
return (
<div className="acc-header-wrapper clearfix">
<ul className="acc-btns-container">
{accountMenuData.map(function (data, index) {
var activeClass = "";
if (self.state.focused === index) {
activeClass = "active";
}
return (
<li
key={index}
className={`${activeClass} ${data.class} main-class`}
onClick={self.clicked.bind(self, index)}
>
<a href="#" className={data.icon}>
{data.name}
</a>
</li>
);
})}
</ul>
</div>
);
By using template literals or string concatenation in the className
attribute, you can add multiple classes to your ReactJS component effortlessly.
Embrace the Power of Multiple Classes! 💪
Now that you know how to add multiple classes to a ReactJS component, it's time to level up your React skills. Start applying this technique in your projects and enhance the styling of your components.
If you found this blog post helpful, don't forget to share it with your fellow React enthusiasts! Let us know in the comments section if you have any questions or need further assistance.
Happy coding! 👩💻👨💻