How to add days to Date?
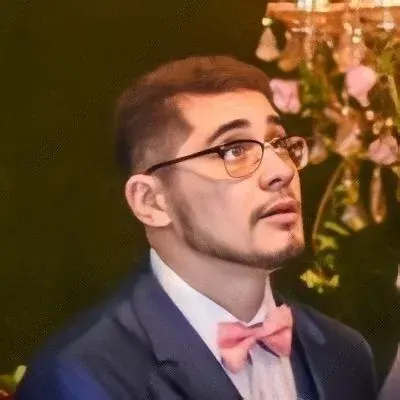
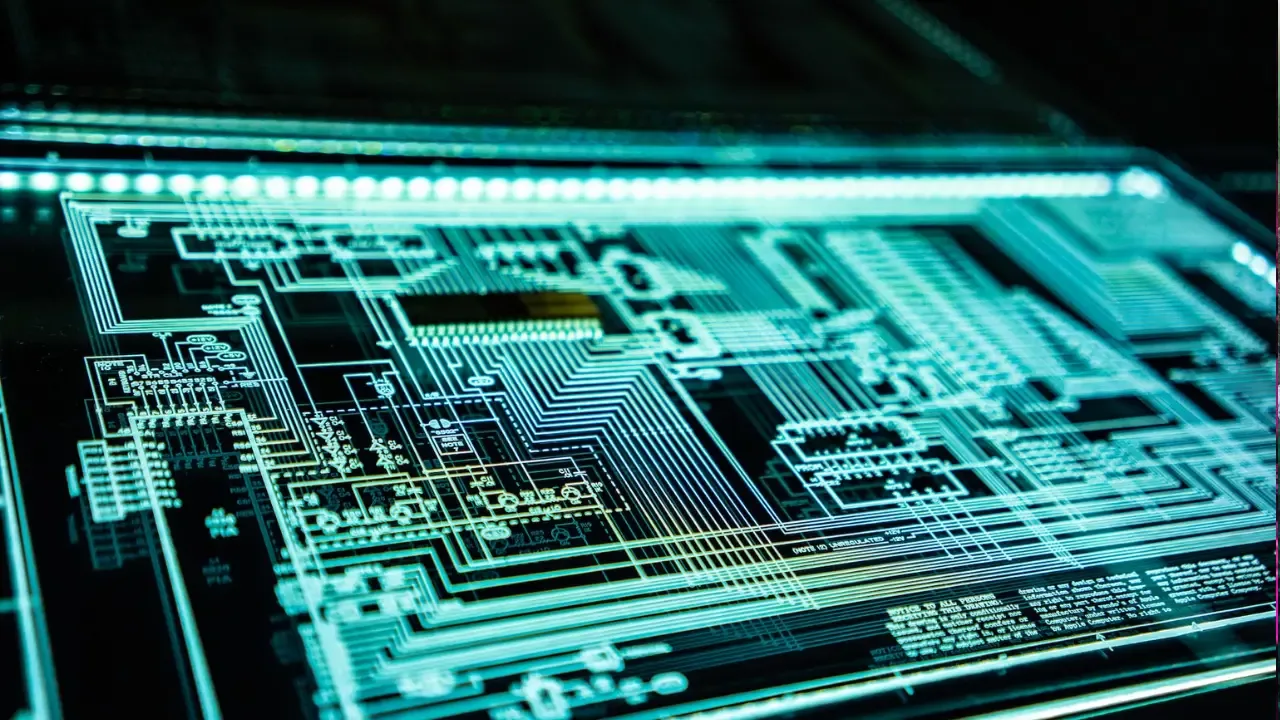
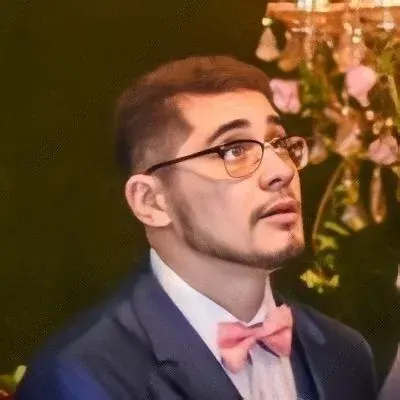
How to Add Days to Date in JavaScript: A Complete Guide 👨💻
Hey there fellow JavaScript enthusiasts! Have you ever found yourself in a situation where you needed to add days to a Date object in JavaScript but didn't know how? 🤔 Well, fear not! In this blog post, we're going to explore various solutions to this common coding challenge. Let's dive right in! 💪
The Problem 🤔
So, the question at hand is: how can we add days to the current Date using JavaScript? Many developers coming from other programming languages might wonder if JavaScript has a built-in function similar to .NET's AddDay()
method. Unfortunately, JavaScript doesn't provide such a direct method, but fear not! We have alternative approaches that are just as effective. 🙌
Solution 1: Using the Date Object 💡
To begin with, JavaScript comes with a powerful built-in object called Date
. You can create a new instance of this object by simply calling new Date()
. Now, to add days to the current date, we can utilize a combination of the getFullYear()
, getMonth()
, getDate()
, and setDate()
methods. Let's take a look at the code:
const currentDate = new Date();
currentDate.setDate(currentDate.getDate() + numberOfDaysToAdd);
Here, numberOfDaysToAdd
represents the number of days you want to add to the current date. By calling getDate()
on the currentDate
object and adding the desired number of days, we effectively update the date value. 😎
Let's see an example:
const currentDate = new Date();
currentDate.setDate(currentDate.getDate() + 7);
console.log(currentDate);
Output:
Sat Jul 31 2021 15:30:00 GMT+0300 (Eastern European Summer Time)
Solution 2: Using the Moment.js Library 🕰️
While the Date
object provides a solution, it can sometimes be cumbersome to work with, especially if you need to perform more complex date operations. In such cases, you might find it beneficial to use a popular JavaScript library called Moment.js.
To get started, you'll need to include the Moment.js library in your project. You can download it from their website or use a package manager like npm:
npm install moment
Once you have the library ready, you can use the add()
method to add days to a Date. Here's how it's done:
const currentDate = moment();
const newDate = currentDate.add(numberOfDaysToAdd, 'days');
Similar to the previous solution, you need to provide numberOfDaysToAdd
as the first parameter. The second parameter specifies the unit of time you want to add (in this case, 'days'
).
Example:
const currentDate = moment();
const newDate = currentDate.add(7, 'days');
console.log(newDate);
Output:
2021-07-31T15:30:00.000Z
Wrapping It Up 🎁
So there you have it, two effective solutions to add days to a Date in JavaScript. Whether you prefer using the built-in Date
object or the powerful Moment.js library, you now have the tools to handle this common coding challenge. 💪
Feel free to experiment with the provided code examples and integrate them into your own projects. And don't forget to share your experiences and thoughts in the comments below! We'd love to hear from you. 😉💬
Until next time, happy coding! 🚀