How to access the correct `this` inside a callback
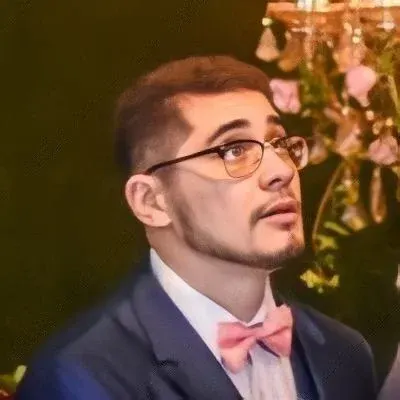

How to Access the Correct this
Inside a Callback: A Comprehensive Guide ๐๐ง๐
So, you're building JavaScript applications and you've come across the notorious problem of accessing the correct this
inside a callback. Fear not! In this guide, we'll dive into common issues related to this problem and provide you with easy solutions. By the end, you'll be a pro at handling your this
references like a boss! ๐ช๐ฅ
Understanding the Problem ๐๐
Let's start by examining the context around this question. You have a constructor function that registers an event handler. Inside the callback function, you want to access a property (data
) of the created object. But alas, this
does not refer to the object you expect it to be. ๐ซ
Issue: this
Refers to the Wrong Object ๐
โโ๏ธ
The problem stems from the fact that JavaScript, by default, treats this
differently inside a callback. When a function is called as a callback, this
is bound to a different object, often the global window
object or undefined
in strict mode. This results in the inability to access the desired object properties.
Solution 1: Arrow Functions ๐นโก๏ธ
One elegant solution is to use arrow functions introduced in ES6. Arrow functions retain the lexical scope of this
, meaning they inherit the this
value from the surrounding code. This solves our problem of accessing the correct object inside the callback.
function MyConstructor(data, transport) {
this.data = data;
transport.on('data', () => {
alert(this.data);
});
}
By using an arrow function as the callback, this
inside the arrow function will be the same as this
in the constructor function. Hurray! ๐
Solution 2: Bind the Callback ๐ค๐ช
Another solution is to explicitly bind the callback function to the correct object using the bind()
method. This ensures that the this
value inside the callback points to the intended object.
function MyConstructor(data, transport) {
this.data = data;
transport.on('data', function() {
alert(this.data);
}.bind(this));
}
By calling .bind(this)
on the callback function, we bind this
to the current object (MyConstructor
instance) and make it accessible inside the callback. Problem solved! โ๏ธ
Solution 3: Use a Local Variable ๐๐ข
If arrow functions and binding are not suitable for your scenario, you can store the value of this
in a local variable before entering the callback. This local variable can then be accessed inside the callback.
function MyConstructor(data, transport) {
var self = this; // Store the desired object context
this.data = data;
transport.on('data', function() {
alert(self.data);
});
}
By assigning this
to self
or any other variable name, we ensure that the correct object context is accessible inside the callback.
Encourage Reader Engagement ๐ฃ๐ฌ
These solutions should help you overcome the challenge of accessing the correct this
inside a callback. Experiment with different approaches and find the one that best suits your needs. Remember, understanding this
in JavaScript is an essential skill for any developer.
Have you encountered similar issues? How did you solve them? Share your experiences and tips in the comments below! Let's learn from each other. ๐ค๐ก
And don't forget to subscribe to our newsletter for more engaging content and helpful tips on JavaScript and web development. Stay ahead of the game! ๐ง๐
Keep coding and keep conquering those this
challenges like a pro! ๐๐ป
(Image Source: Unsplash)
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
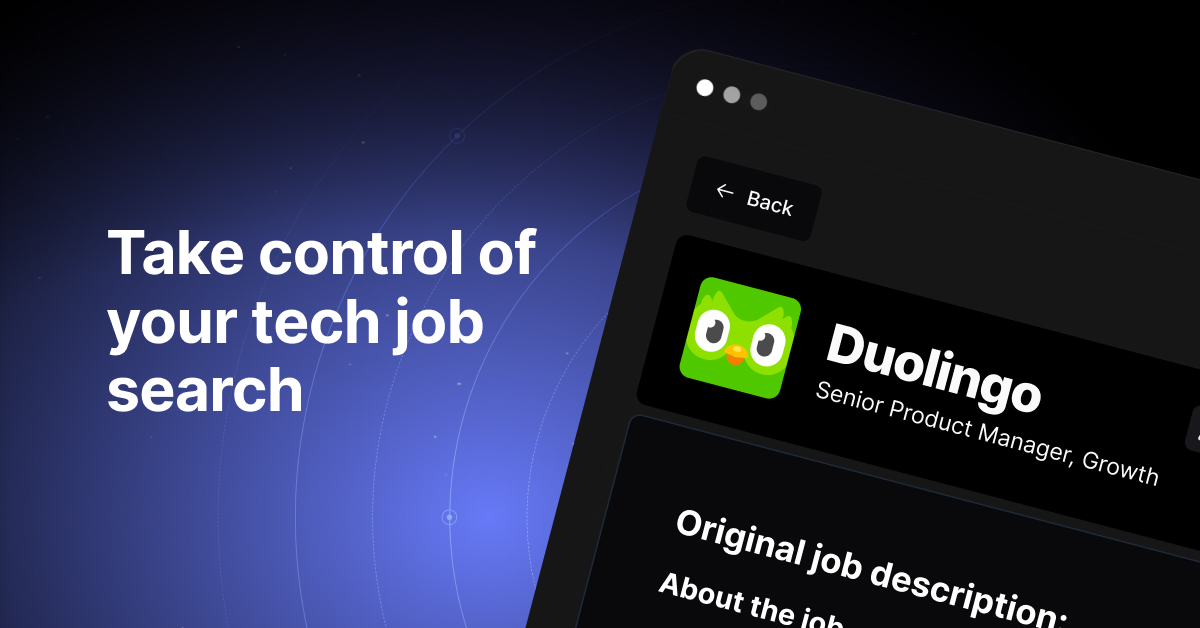