How to access POST form fields in Express
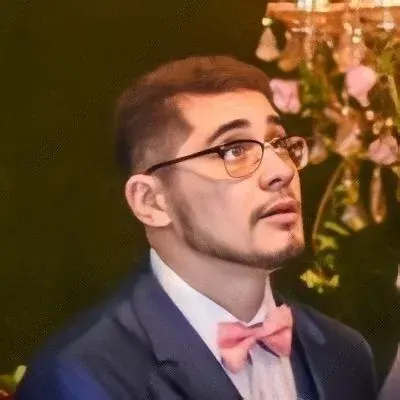
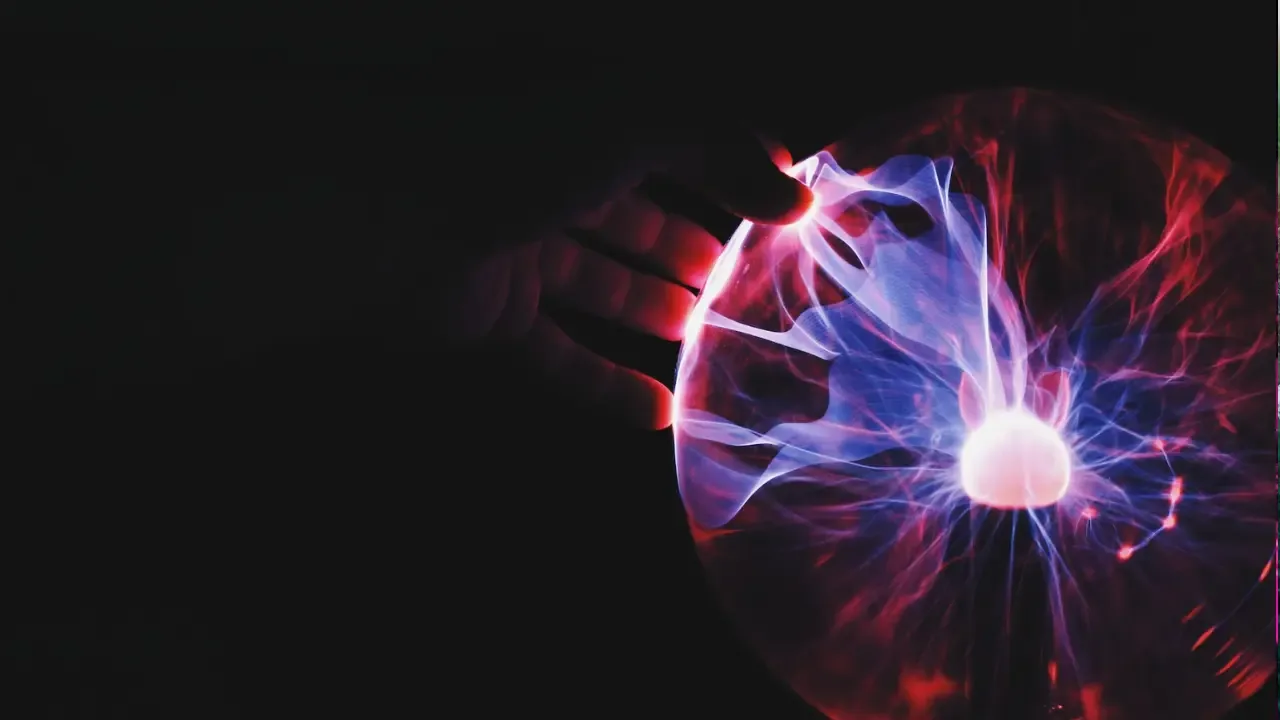
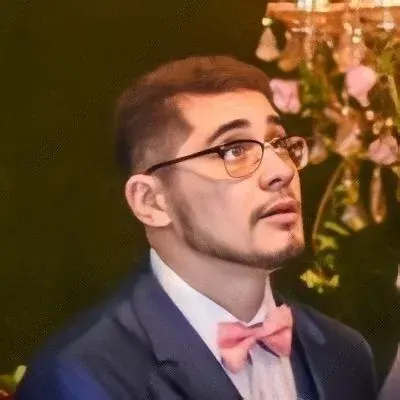
📝 How to Access POST Form Fields in Express 🌐
Welcome, fellow developers! Today, we're going to tackle a common issue that many of us have faced when working with Express.js and Node.js: accessing POST form fields. 🤔
Imagine this scenario: you have a simple form with an email input field, and you want to retrieve the value of that field on the server-side using Express. Let's dive in! 💻
👉 The Form 👈
Here's a snippet of the HTML form we'll be working with:
<form id="loginformA" action="userlogin" method="post">
<div>
<label for="email">Email: </label>
<input type="text" id="email" name="email"></input>
</div>
<input type="submit" value="Submit"></input>
</form>
🏃♂️ The Express.js Code 🏃♀️
In your Express.js app, you have a route that handles the form submission. Here's an example:
app.post('/userlogin', function(sReq, sRes){
var email = sReq.query.email.;
}
🚫 The Issue 🚫
Now, here's where things can get confusing. The developer in this context tries to access the value of the email field using sReq.query.email
or sReq.query['email']
or even sReq.params['email']
. However, all of these approaches return undefined
. 😱
🤔 The Solution 🤔
First of all, we need to understand that query
and params
are used to access values from the URL parameters or query string, not from the body of a POST request. 📩
To access the POST form fields, we'll need to use the body-parser
middleware. This middleware is essential for parsing the body of an incoming request. Here's how you can implement it in your Express.js app:
Install the
body-parser
package by runningnpm install body-parser
.Require the
body-parser
module in your Express.js app:const bodyParser = require('body-parser');
Register the
body-parser
middleware in your app:app.use(bodyParser.urlencoded({ extended: false }));
Finally, you can access the values of the form fields using the
body
property of the request object:app.post('/userlogin', function(sReq, sRes) { var email = sReq.body.email; // Now you can do whatever you need with the email value });
And there you have it! By implementing the body-parser
middleware and accessing the form field through sReq.body.email
, you can successfully retrieve the value of the email field from the POST request body. 🎉
💡 Pro Tip: Don't forget to include the name
attribute on your HTML input fields. This attribute is crucial for mapping the form field values to the request body properties.
So, the next time you find yourself struggling to access POST form fields in Express.js, remember the power of the body-parser
middleware. 💪
🔥 Your Turn! 🔥
Now that you have the solution, it's time to give it a try! Update your code, test it out, and let us know your results in the comments below. If you have any other tips or questions, we'd love to hear them too! 🗣
Keep coding, keep learning, and happy form field accessing! 🚀🌈
📚 Further Reading: