How to access a child"s state in React
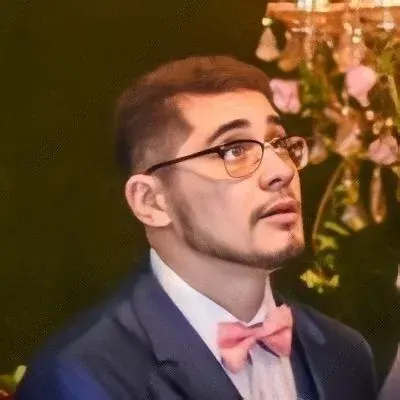
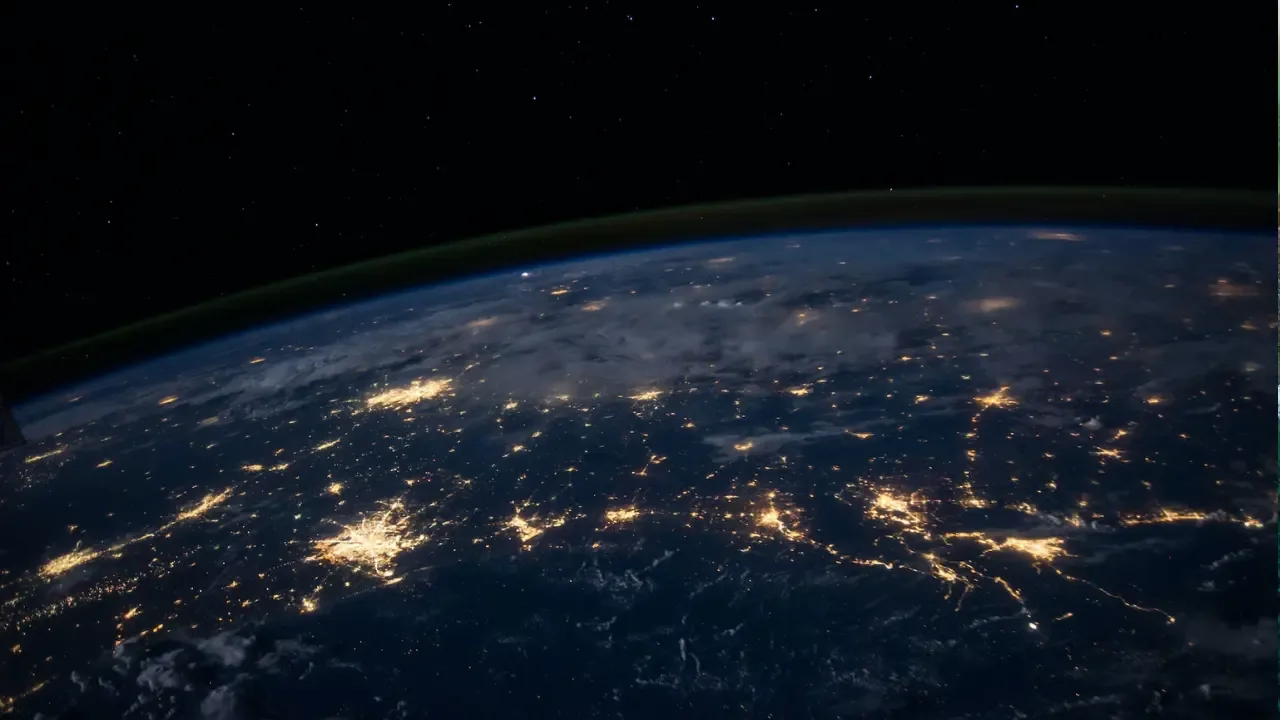
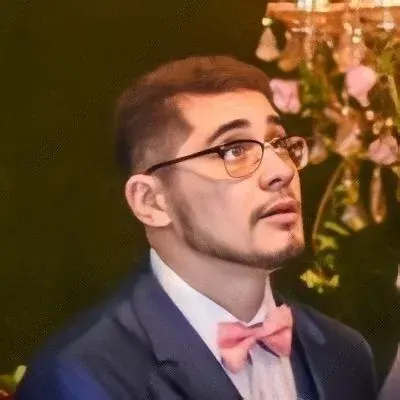
How to Access a Child's State in React: A Complete Guide
Have you ever found yourself in a situation where you needed to access the state of a child component in React? 🤔 You're not alone! In this blog post, we'll explore how to tackle this common issue and provide you with easy solutions. So let's dive in! 💪
The Scenario
Let's take a look at the scenario presented by our fellow developer:
"I have the following structure:
FormEditor
- holds multiple instances ofFieldEditor
FieldEditor
- edits a field of the form and saves various values about it in its state
When a button is clicked within FormEditor
, I want to be able to collect information about the fields from all FieldEditor
components, information that's in their state, and have it all within FormEditor
."
Solution 1: Lifting State Up 🎈
One way to access the state of child components is by lifting the state up to the parent component, in this case, the FormEditor
. By managing the state at a higher level, you can easily access and manipulate it as needed.
Here's an example of how you can implement this approach:
// FormEditor.jsx
import React, { useState } from 'react';
import FieldEditor from './FieldEditor';
const FormEditor = () => {
const [fields, setFields] = useState([]);
const handleFieldUpdate = (fieldData) => {
// Update the fields state with the received data
setFields([...fields, fieldData]);
};
const handleButtonClick = () => {
// Access and process the fields state
console.log(fields);
// ... additional logic
};
return (
<div>
{fields.map((field, index) => (
<FieldEditor
key={`field-${index}`}
onUpdate={handleFieldUpdate}
/>
))}
<button onClick={handleButtonClick}>Collect Information</button>
</div>
);
};
export default FormEditor;
In the above example, we've lifted the fields
state up to the FormEditor
. Each FieldEditor
component receives an onUpdate
prop, which is a callback function passed down from the parent component. Whenever a field is updated in FieldEditor
, it calls this function, updating the state in the FormEditor
component.
Solution 2: Using Refs 📌
Another approach to accessing the child component's state is by using React refs. Refs provide a way to directly access and interact with a child component's DOM node or instance.
Let's see how it can be implemented in this scenario:
// FormEditor.jsx
import React, { useRef } from 'react';
import FieldEditor from './FieldEditor';
const FormEditor = () => {
const fieldEditorRefs = useRef([]);
const handleButtonClick = () => {
const fieldData = fieldEditorRefs.current.map((ref) => ref.current.getFieldData());
console.log(fieldData);
// ... additional logic
};
return (
<div>
{fields.map((field, index) => (
<FieldEditor
key={`field-${index}`}
ref={(ref) => (fieldEditorRefs.current[index] = ref)}
/>
))}
<button onClick={handleButtonClick}>Collect Information</button>
</div>
);
};
export default FormEditor;
In this approach, we create a fieldEditorRefs
ref using React's useRef
hook. Inside the render
method, we pass a callback to the ref
prop of each FieldEditor
component, capturing the reference to it. The handleButtonClick
function then iterates over the refs and calls a method getFieldData()
defined in the FieldEditor
component to collect the necessary data.
While using refs can provide direct access to a child component's state, it's important to note that you should use this approach sparingly and consider whether lifting state up might be a more suitable solution.
Conclusion
Accessing a child's state in React can be achieved by lifting the state up or using refs. Depending on your specific scenario, you can choose either approach or explore other techniques available in the React ecosystem.
Remember, the key is to carefully assess your application's requirements and decide which solution works best for you. Now it's your turn! 👊
Have you encountered any challenges when accessing a child's state in React? Share your experiences and insights in the comments below, and let's learn from each other! 🙌