How does the "this" keyword work, and when should it be used?
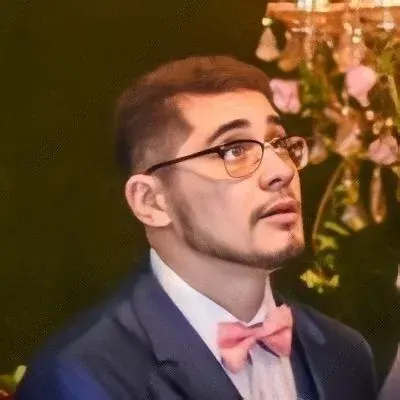
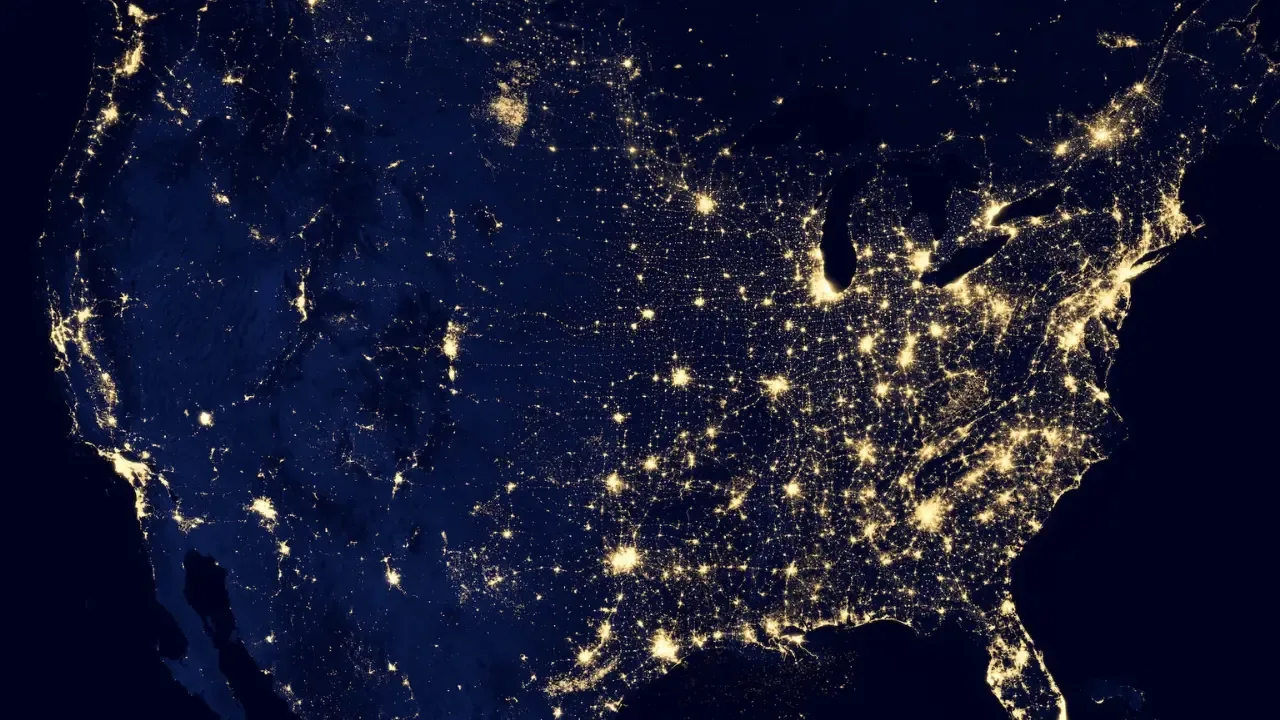
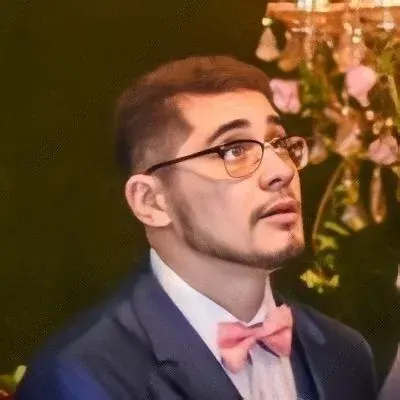
๐ Tech Blog: A Guide to Understanding the "this" Keyword in Programming
Welcome, fellow tech enthusiasts! Today, we dive into the intriguing world of the "this" keyword in programming. ๐
Have you ever found yourself scratching your head, wondering how and when to use the "this" keyword? You're not alone! Many developers have experienced the mysterious behavior of "this" and sought a clear explanation โ just like our friend who reached out to us for help. So, let's get right into it!
๐ค What is the "this" Keyword and Why Does it Behave Strangely?
The "this" keyword in programming languages like JavaScript and Java refers to the current object or instance being worked on. It allows us to access and manipulate the properties and methods associated with that particular object. ๐คนโโ๏ธ
But why does it sometimes behave strangely? ๐คจ Well, that's because its value depends on the context in which it is used. Its behavior can change based on how and where it is being invoked. This is where things can get a little tricky, leading to unexpected results if misused or misunderstood.
๐ก Understanding the Different Usages of "this"
To fully grasp the concept of "this," let's take a look at its different usages:
1. Method Invocation
When "this" is used within a method of an object, it refers to the object itself. Imagine a "car" object with a "drive" method. When we invoke the "drive" method using dot notation, like "car.drive()", the "this" keyword within "drive" refers to the "car" object. ๐
const car = {
brand: "Tesla",
drive() {
console.log("Driving a", this.brand);
}
};
car.drive(); // Output: Driving a Tesla
2. Constructor Function
In JavaScript, when creating objects using a constructor function, "this" refers to the newly created instance of the object. ๐ญ Here's an example using a "Person" constructor function:
function Person(name, age) {
this.name = name;
this.age = age;
}
const john = new Person("John", 25);
console.log(john.name, john.age); // Output: John 25
3. Event Handlers
In the context of event handlers, such as "click" or "submit" events, "this" refers to the element that triggered the event. ๐ฑ๏ธ This allows us to access and manipulate the properties and behaviors of that particular element.
const button = document.querySelector("button");
button.addEventListener("click", function() {
console.log("Button clicked:", this);
});
๐ก Pro Tip: In JavaScript, arrow functions do not have their own "this" binding. They inherit it from the enclosing scope.
๐ Unlocking the Power of "this"
Now that we understand the different usages of "this", let's explore some best practices to avoid common pitfalls and make the most of this powerful keyword:
Consistency is ๐: Be consistent with your use of "this" throughout your codebase. Ensure it always refers to the expected object or instance to maintain clarity.
Bind, Apply, or Call: For fine-grained control over the value of "this" within functions, you can use the "bind", "apply", or "call" methods. These allow you to explicitly set or change the value of "this".
Arrow Functions: Consider using arrow functions when you don't need to access the "this" value of the enclosing scope. Arrow functions automatically bind "this" to the context in which they are defined.
๐ข Your Turn to Dive In!
Congratulations, you've unlocked a deeper understanding of the elusive "this" keyword in programming! You now possess the knowledge to wield it wisely and avoid those perplexing moments of unexpected behavior.
Feel free to experiment with "this", and don't shy away from sharing your experiences or any additional questions you may have in the comments section below. Let's continue this fascinating conversation! ๐
Happy coding! ๐ปโจ