How does JavaScript .prototype work?
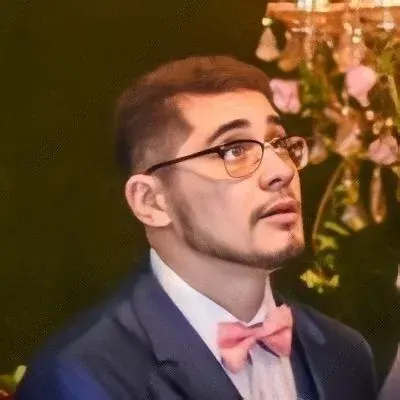
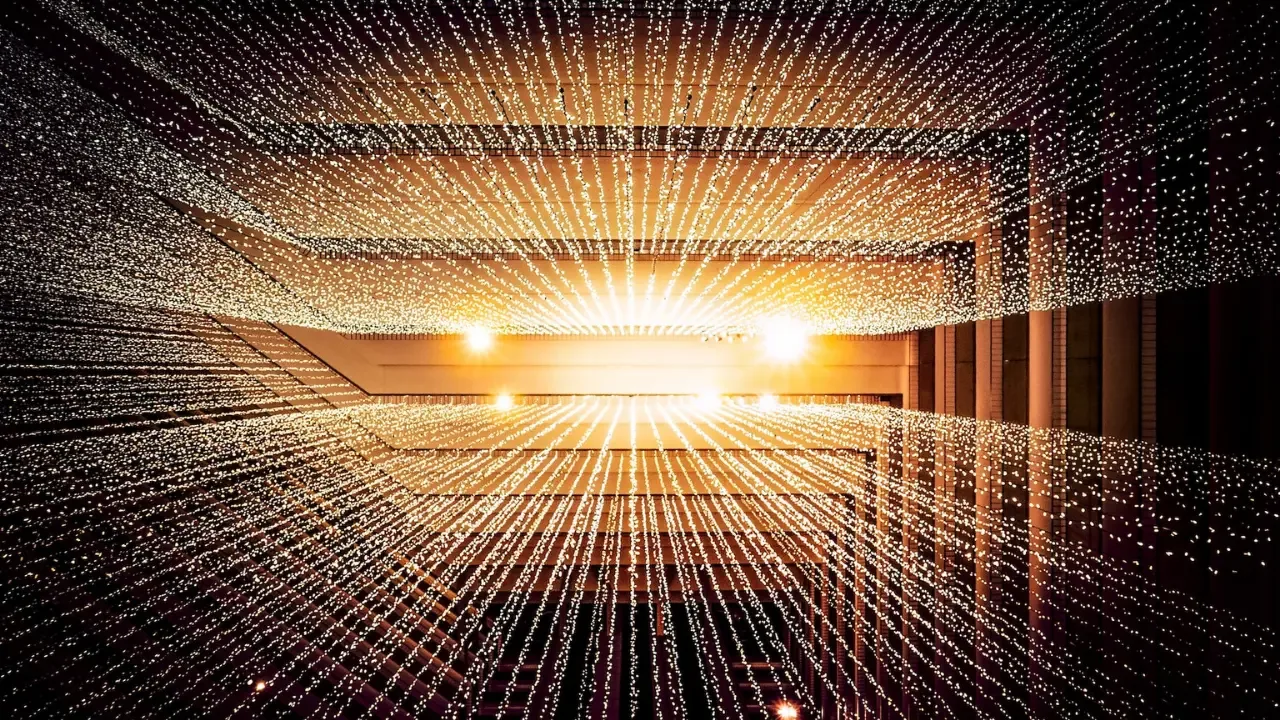
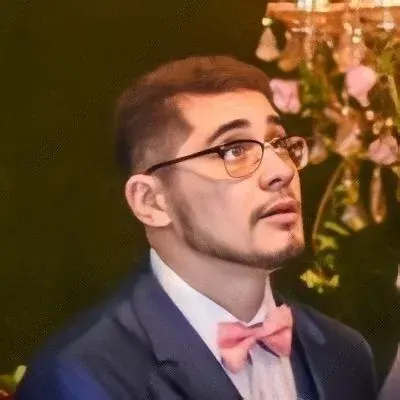
How does JavaScript .prototype
work? ๐ค
If you've delved into JavaScript, you might have come across the concept of .prototype
and wondered what it really does. ๐คทโโ๏ธ Don't worry, you're not alone! In this blog post, we'll demystify the JavaScript .prototype
and explain how it works. By the end, you'll have a crystal-clear understanding of this important concept. ๐
Understanding the Basics ๐
In traditional object-oriented programming languages (like Java or C++), we have classes and objects. However, JavaScript takes a different approach. Instead of using classes, it utilizes prototype-based programming. ๐ญ
In JavaScript, objects are created from constructor functions or through object literals. Each object has an internal link to another object called its prototype. Think of it as a blueprint that defines the properties and methods of the object. ๐๏ธ
Prototype Chaining: The Magic Behind JavaScript Objects โจ
When you access a property or method on an object, JavaScript follows a process called prototype chaining to look for it. It first checks the properties and methods of the object itself. If it doesn't find them, it then looks up to its prototype, then the prototype's prototype, and so on, until it either finds the desired property or reaches the end of the chain (when the prototype is null
). ๐งต
This allows objects to inherit properties and methods from their prototypes, creating a hierarchy of shared functionality. ๐ค Let's illustrate this with an example:
var obj = new Object(); // Not the correct way
obj.prototype.test = function() {
alert('Hello?');
};
var obj2 = new obj(); // This is wrong!
obj2.test();
Here, we're creating obj
using new Object()
, but this is not the proper way to create objects with prototypes in JavaScript. ๐ซ
The Correct Way to Use Prototypes โ
To create objects with prototypes in JavaScript, we need to use constructor functions. These functions serve as templates for creating objects with shared properties and methods. Here's the correct way to do it:
function MyObject() {} // A first-class functional object
MyObject.prototype.test = function() {
alert('OK');
};
var obj = new MyObject(); // Creating object with the constructor function
obj.test(); // Alerts 'OK'
In this updated example, MyObject()
serves as a constructor function, and we attach the test
method to its prototype. Now, when we create an object using new MyObject()
, it properly inherits the test
method and alerts 'OK'. โ๏ธ
Additional Resources for Deep Dive ๐
If you want to explore this topic further, there are great resources available. One of the best is the slides by John Resig that shed light on the intricacies of JavaScript's prototype system. ๐
Wrap Up and Engage! ๐
We've covered the fundamentals of JavaScript .prototype
, understanding how it relates to object instantiation and the proper way to use it. ๐ Now it's your turn! Put your newfound knowledge to the test and experiment with prototypes in your JavaScript projects. Share your experiences and let us know how you're leveraging .prototype
to enhance your code. ๐ก
Leave a comment below and join the conversation! If you found this blog post helpful, don't forget to share it with your fellow JavaScript enthusiasts. Let's spread the love for JavaScript and its powerful prototype-based programming! ๐ช