How does `Array.prototype.slice.call` work?
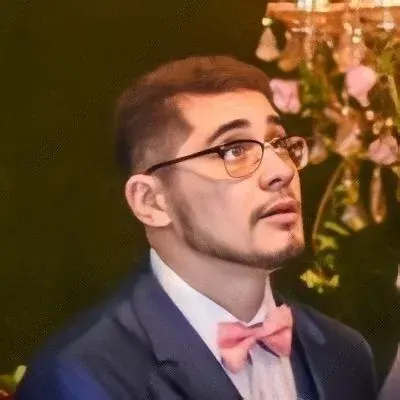
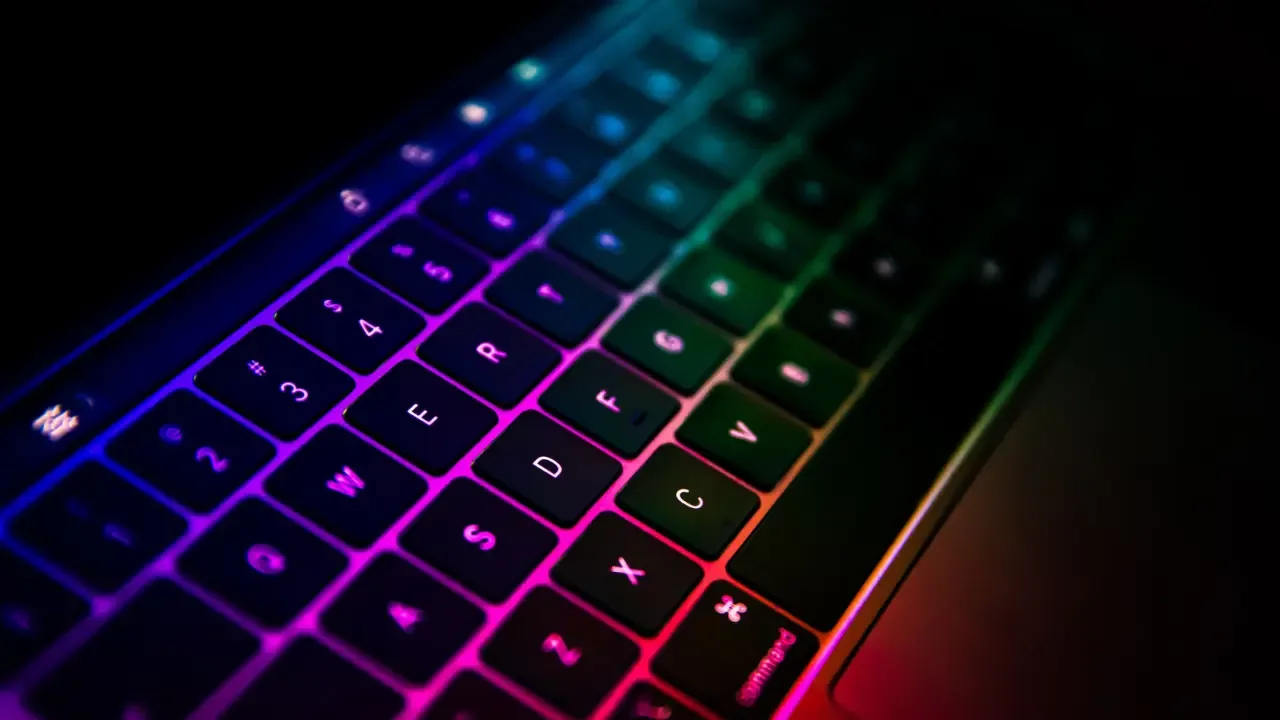
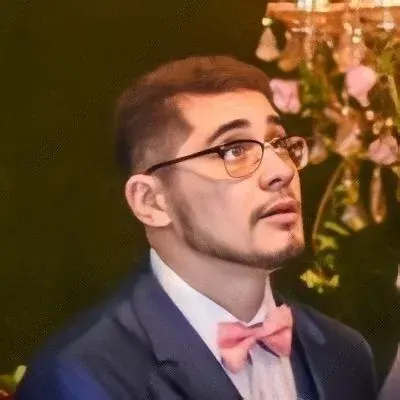
How Does Array.prototype.slice.call
Work?
You've come across this funky piece of code: Array.prototype.slice.call(arguments)
, and you're scratching your head wondering how it works. Fear not, my tech-savvy friend! In this blog post, we're going to dive deep into the world of Array.prototype.slice.call
and demystify its inner workings.
Understanding the Context
Before we jump into the nitty-gritty, let's first understand the context in which Array.prototype.slice.call
is used. You mentioned that you know it is used to turn arguments
into a real Array
. But what does that even mean?
In JavaScript, the arguments
object is a special object available within functions. It contains an array-like structure of all the arguments passed to the function. However, arguments
is not an actual array with all the array methods at our disposal. This is where Array.prototype.slice.call
comes to the rescue!
The Magic of Array.prototype.slice.call
To put it simply, Array.prototype.slice.call
is a clever way of borrowing the slice
method from the Array.prototype
and using it on array-like objects, such as arguments
.
Let's break it down step by step:
Array.prototype
refers to the prototype object of theArray
constructor function. It contains all the methods that are available on arrays.slice
is one of those methods available on theArray.prototype
. It allows us to extract a portion of an array and return it as a new array.By using the
call
method onslice
, we are essentially telling JavaScript to invoke theslice
method and passarguments
as its context, or more simply, as the value ofthis
. This allows us to treatarguments
as if it were an array and use theslice
method on it.The result is a real array containing all the elements from
arguments
. 🎉
To better visualize this, let's look at an example:
function sumAll() {
var args = Array.prototype.slice.call(arguments);
var total = 0;
args.forEach(function(num) {
total += num;
});
return total;
}
console.log(sumAll(1, 2, 3, 4)); // Outputs: 10
In the above example, we use Array.prototype.slice.call(arguments)
to convert arguments
into a real array. We can then iterate over this array using the forEach
method and calculate the sum of all elements.
Common Issues and Easy Solutions
Even though Array.prototype.slice.call
is a handy technique, it can sometimes lead to confusion and bugs. Here are a few common issues and their easy solutions:
1. Forgetting to use Array.prototype.slice.call
If you forget to use Array.prototype.slice.call
and try to directly use slice.call
, it won't work because slice
is not defined in the global scope. Remember to always add Array.prototype
before slice.call
.
2. Misunderstanding the Purpose
People often wonder why we need to convert arguments
into an array. Well, sometimes we want to perform operations that require array methods, such as forEach
, map
, or reduce
.
3. Using the Rest Parameter Instead
Since ES6, we can use the rest parameter syntax to convert function arguments into an array. It's often a more elegant and readable solution:
function sumAll(...args) {
var total = 0;
args.forEach(function(num) {
total += num;
});
return total;
}
Time to Slice and Dice!
Congratulations, you've just unlocked the secrets behind Array.prototype.slice.call
! It's a powerful technique that allows us to work with array-like objects as if they were real arrays.
Now that you understand how it works, go forth and slice and dice your arguments like a pro! Remember to use it wisely, and may your code be clean and free of bugs! 💪💻
If you have any questions or thoughts on this topic, feel free to leave a comment below! Let's keep the conversation going. 😊✨