How do you test for the non-existence of an element using jest and react-testing-library?
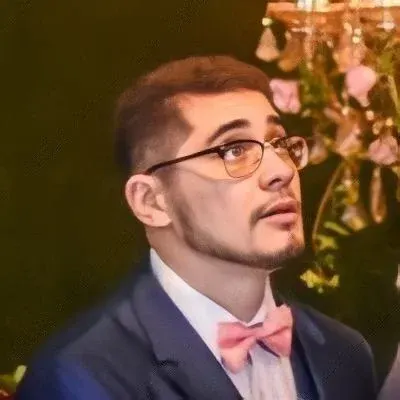
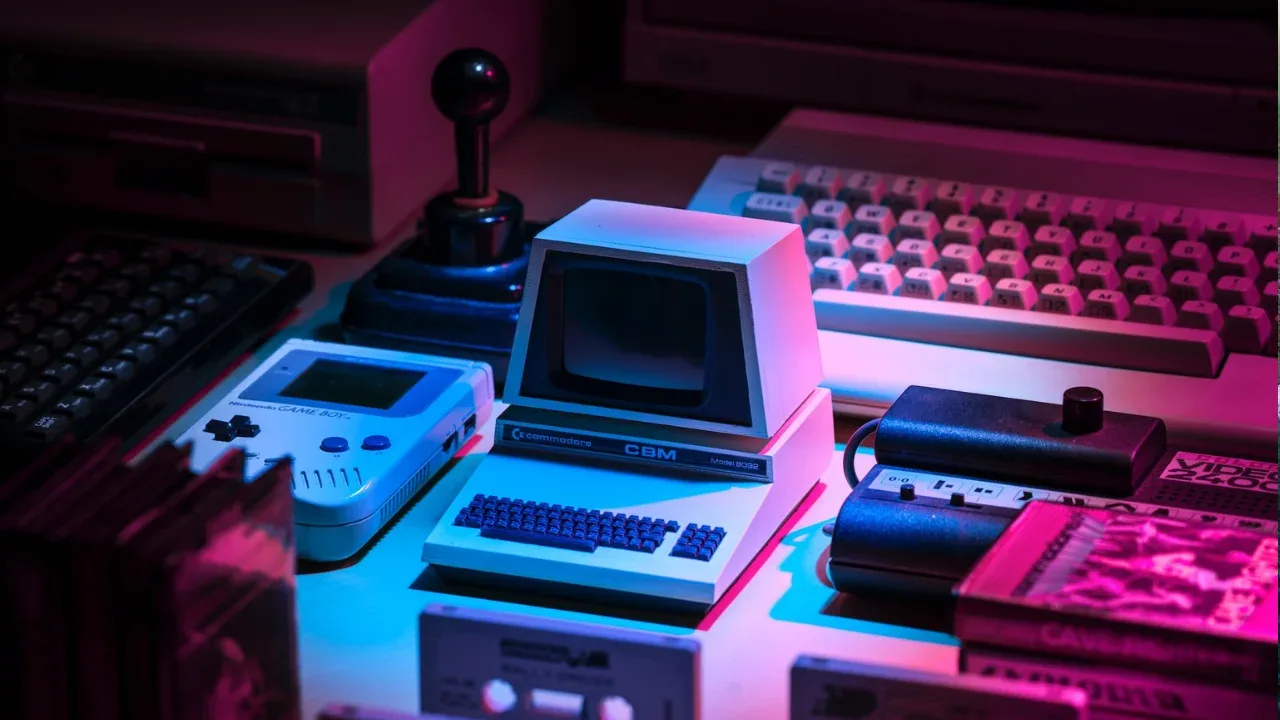
✨ Testing the Non-Existence of an Element in React with Jest and react-testing-library ✨
So you're writing unit tests for your component library using Jest and react-testing-library. 👩💻 Fantastic! But wait, there's a little challenge you've encountered. You want to verify that certain elements are not being rendered based on certain props or events. 🤔
The first instinct might be to use getByText
, getByTestId
, or similar methods provided by react-testing-library
to look for the absence of an element. However, using these methods would throw an error if the element is not found, causing the test to fail prematurely, before even reaching the expect
function. ❌
So, how do we tackle this issue and test for the non-existence of an element? Let's dive right into it! 🏊♀️
🧪 The Solution: Using Query Methods
In react-testing-library
, we have a set of query methods prefixed with query
instead of get
. These methods allow us to check for the presence or absence of an element without throwing an error. 🕵️♀️
Here are a few of these handy query methods:
queryByText
queryByTestId
queryByRole
queryByLabelText
These methods will return null
if the element is not found, instead of throwing an error. This behavior enables us to perform assertions on the non-existence of elements without obstructing subsequent test code. ✔️
Now, let's take a look at an example to illustrate how we can use these query methods effectively.
import { render } from 'react-testing-library';
test('MyComponent should not render a specific element', () => {
const { queryByText } = render(<MyComponent />);
const specificElement = queryByText('Specific Element');
expect(specificElement).toBeNull();
});
In this example, we render MyComponent
and then use queryByText
to search for the element with the text "Specific Element". Since the element is not supposed to be present, specificElement
will be null
, and our expectation toBeNull()
will pass. 🙅♀️
🚀 Engage with the Community
Testing for the non-existence of elements is a common challenge, but now you have the tools to overcome it! Try out the query methods in your own tests and see the magic unfold. ✨
If you have any further questions or run into any issues, feel free to reach out to our vibrant community. We're here to help! 🤝
Let's keep the conversation going! Leave a comment below and share your experiences with testing the non-existence of elements. Have you encountered any interesting scenarios? We'd love to hear them! 📢
Happy testing and happy coding! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
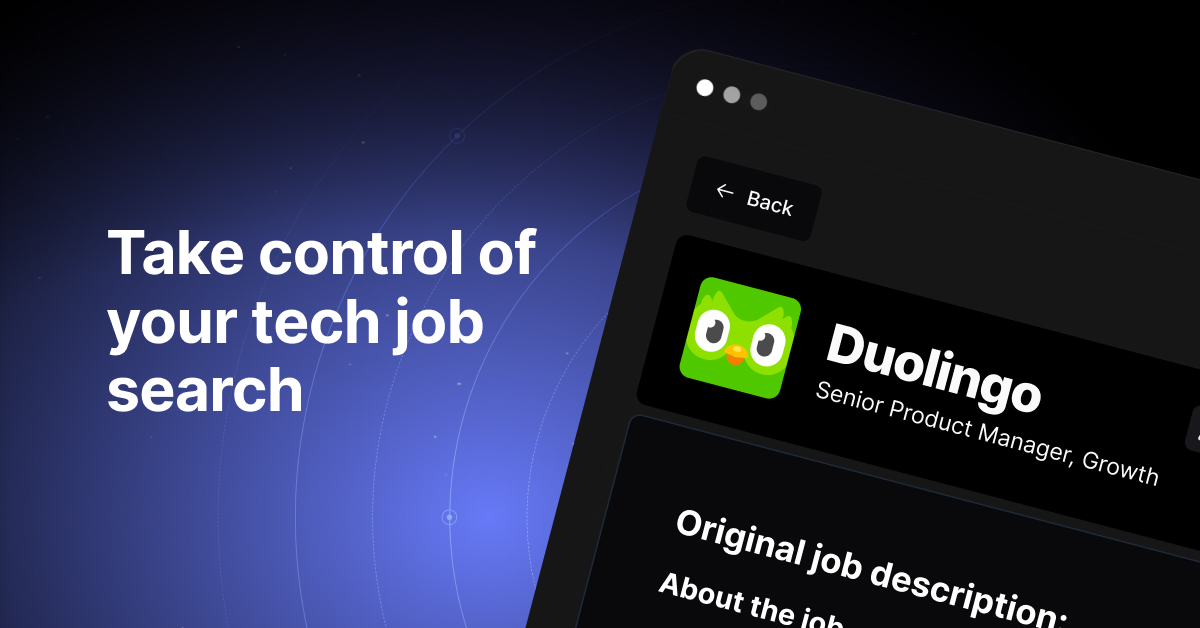