How do you set the document title in React?
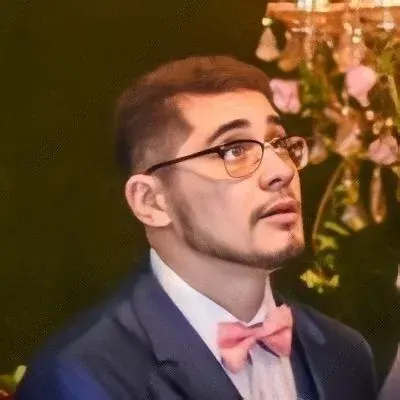
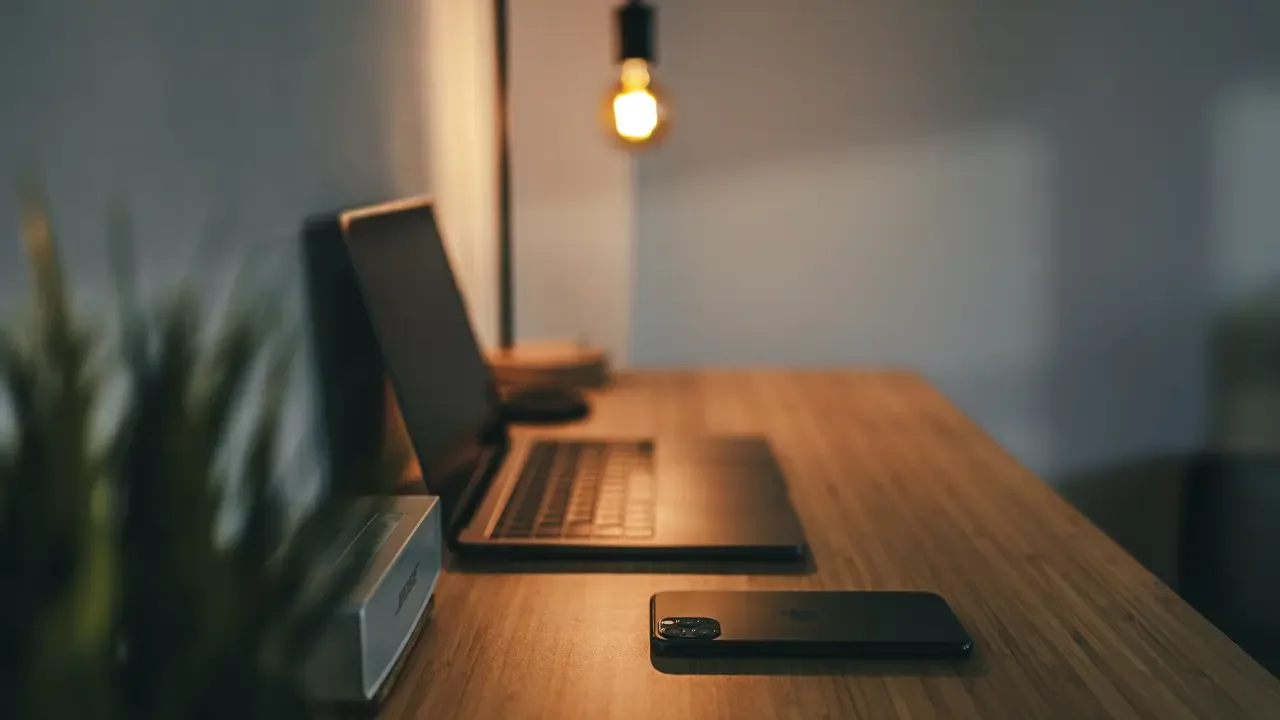
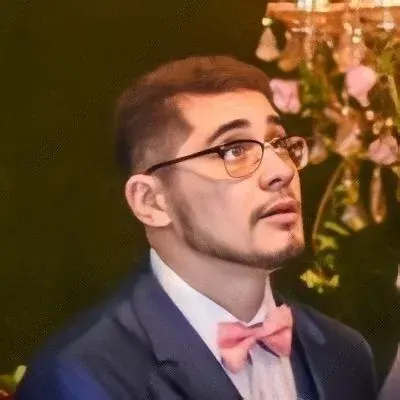
How to Set the Document Title in React: A Simple Guide 📝🔍
So, you want to set the document title in your React application? 🤔 We've got you covered! In this guide, we'll address the common issues you might encounter and provide you with easy solutions. Let's dive right in! 💪
The Problem 😩
You mentioned that you tried using react-document-title
, but it seems to be out of date. 😕 Additionally, setting document.title
in the constructor
and componentDidMount()
methods didn't work for you. Let's explore some possible reasons for these issues and how to solve them. 🧐
Possible Causes and Solutions 🛠️
1. Timing Issue ⏰
The most common reason for the document.title
not updating could be the timing of when you are trying to set it. If you set the title too early, it might not reflect the desired changes.
💡 Solution: To ensure the title updates correctly, you can use the componentDidUpdate()
lifecycle method. This method is triggered after the component updates, allowing you to set the document title at the right time, like so:
componentDidUpdate() {
document.title = "Your New Document Title";
}
2. External Libraries and Dependencies 📚
The library you mentioned, react-document-title
, seems to be outdated. It is always recommended to use up-to-date and well-maintained libraries to avoid compatibility issues.
💡 Solution: Instead of relying on an external library, you can make use of the useEffect
hook introduced in React 16.8. This hook allows you to perform side effects in functional components, including updating the document title. Here's an example:
import React, { useEffect } from "react";
function YourComponent() {
useEffect(() => {
document.title = "Your New Document Title";
return () => {
document.title = "Previous Document Title";
};
}, []);
// ...
}
In this example, we are using useEffect()
with an empty dependency array, []
. This ensures that the effect runs only once, similar to the behavior of componentDidMount()
.
3. Async Updates or AJAX Requests 🔄
If you are making asynchronous updates or AJAX requests that affect the document title, you need to make sure that the title gets updated after the data has loaded or the updates have been made.
💡 Solution: You can use async/await or promises to handle the asynchronous part of your code, and then set the document title once you receive the data or complete the updates. Here's an example:
async function fetchData() {
// Fetch data or make updates
// ...
document.title = "Your New Document Title";
}
// Call your async function where needed, like within a click event or after data has loaded
Ensure that your async code runs successfully, and once the desired changes are made, set the document title accordingly.
Conclusion and Call-to-Action 🏁💬
Setting the document title in React is achievable once you understand the right timing, choose the appropriate approach without relying on outdated libraries, and handle any asynchronous updates appropriately. 🎉
Now it's your turn! Share your experiences or any other tips you have for setting the document title in React in the comments below. Let's learn from each other! 💡💬
Remember, if you found this guide helpful, feel free to share it with your fellow React developers. Happy coding! 👩💻👨💻