How do you remove all the options of a select box and then add one option and select it with jQuery?
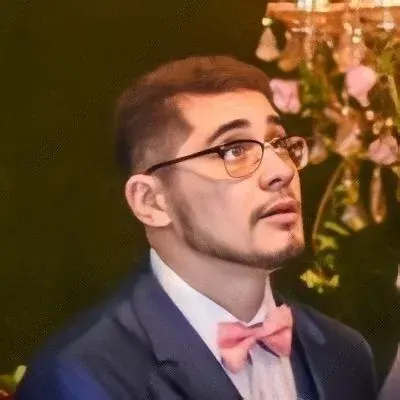
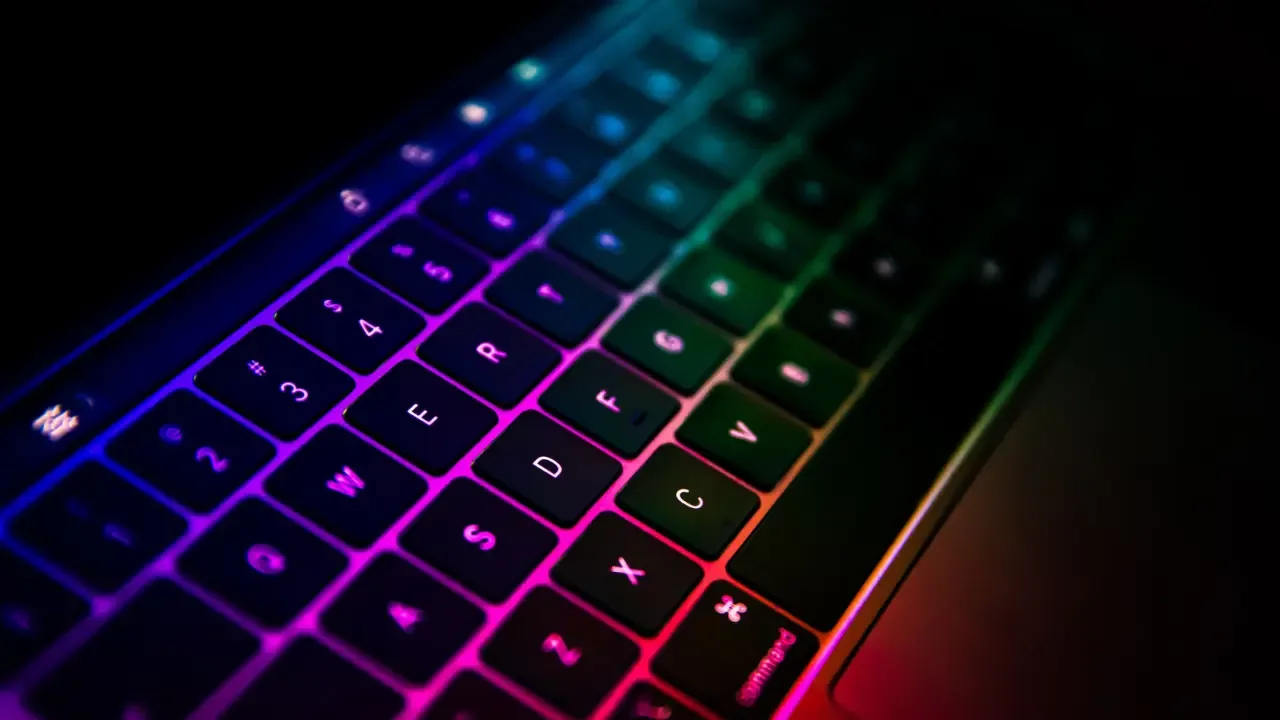
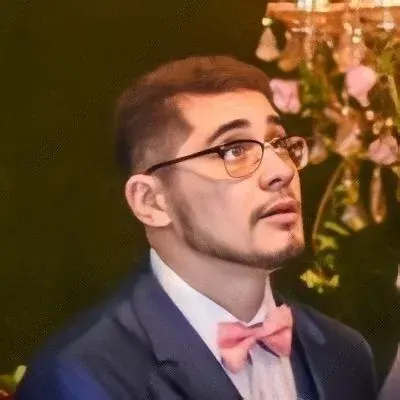
How to Remove All Options from a Select Box and Add One Option Using jQuery 🔄
Have you ever needed to manipulate a select box dynamically in your web application using jQuery? Perhaps you needed to remove all the options from the select box and replace it with just one option that you want to select programmatically. In this blog post, we will explore a common issue faced by developers and provide easy solutions to achieve this task. Let's dive in! 💦
The Problem 😕
Consider the following scenario: you have a select box with multiple options, and you want to remove all these options, then add one specific option and select it programmatically using jQuery. You may have already tried different approaches, but none seems to work as expected, especially in Internet Explorer (we all know how thorny IE can be! 🌐).
The code snippets shared by other developers may have provided some insights, but they didn't quite solve the problem entirely for you. You're looking for a foolproof solution that works consistently across different browsers. Don't worry, we've got your back! 🤗
The Solution 💡
To remove all the options from a select box and add one option that you want to select programmatically using jQuery, you can follow this step-by-step solution:
Find the select box using its ID: Start by selecting the select box using its
id
attribute. In your case, the ID ismySelect
. This can be done using the$('#mySelect')
jQuery selector.Remove all existing options: To remove all the options from the select box, use the
find('option').remove()
chain. This chain first finds all the options inside the select box and then removes them. By chaining theend()
method at the end, you ensure that the subsequent methods will be applied to the original selection.Add the desired option: After removing all the options, you can add the desired option using the
append()
method. This method takes a string representing the new option and appends it to the select box. Make sure to specify thevalue
attribute and the displayedtext
of the option according to your needs.Select the added option: To select the newly added option programmatically, use the
val()
method. Pass the desired value as the parameter, which should match thevalue
attribute of the option you added in the previous step. This ensures that the option will be selected in your select box.
Putting it all together, here's the code:
$('#mySelect').find('option').remove().end()
.append('<option value="whatever">text</option>')
.val('whatever');
But, What About Internet Explorer? 🌐
You mentioned that the previous code didn't work as expected in Internet Explorer. Don't worry; we got you covered! To ensure cross-browser compatibility, try the following alternative solution:
$('#mySelect').children().remove().end()
.append('<option selected value="whatever">text</option>');
This code achieves the same goal by clearing out all the children elements of the select box (i.e., the options) and then adding the desired option with the selected
attribute set to true.
You Made It! ✨
Congratulations, you've successfully learned how to remove all options from a select box and add one option while selecting it programmatically using jQuery! Now you can dynamically manipulate select boxes with ease. 🎉
Feel free to apply this knowledge to your web applications and save yourself from the headache of dealing with tricky select box manipulations. If you have any more questions or need further assistance, don't hesitate to reach out to us. We're here to help! 😊
Take It a Step Further! 💪
Now that you've mastered this select box manipulation technique, why stop here? You can explore more amazing functionalities provided by jQuery and level up your web development skills. Remember, practice makes perfect! 🚀
Let us know if you found this blog post helpful by leaving a comment below. Share it with your developer friends so they can benefit from this easy solution too. Together, we can make the web development world a better place! 👥
Happy coding! ✍️💻